How to Check if a Single Cell Value is NaN in Pandas
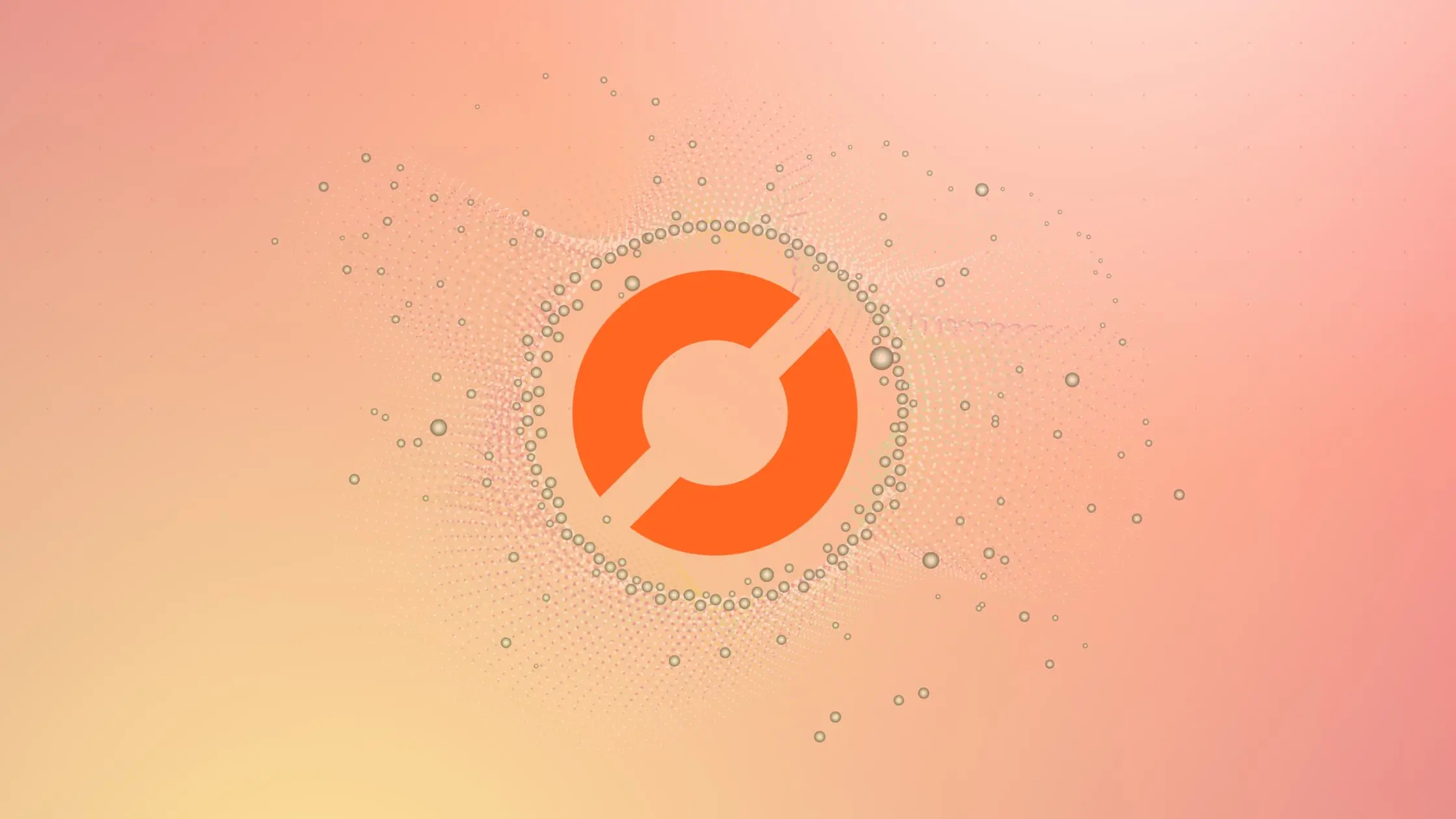
How to Check if a Single Cell Value is NaN in Pandas
As a data scientist or software engineer, you know that working with data can be messy. Missing data or NaN (Not a Number) values can be a common problem when dealing with large datasets. As a result, it’s essential to know how to handle such scenarios effectively.
In this article, we’ll explore how to check if a single cell value is NaN in Pandas, a popular data manipulation library in Python. We’ll also discuss why it’s essential to identify and handle missing data and NaN values in your datasets.
Why is it Important to Handle NaN Values in your Datasets?
Missing data or NaN values can arise due to various reasons. For instance, data may not have been collected for a particular attribute, or the data may have been lost during the data collection process. Regardless of the reason, missing data can lead to inaccurate results, biased analysis, and incorrect decision-making.
Ignoring NaN values in your datasets can lead to skewed statistics, incorrect calculations, and misleading visualizations. Therefore, it’s crucial to identify and handle NaN values appropriately to avoid erroneous results.
How to Check if a Single Cell Value is NaN in Pandas
Pandas provides several methods to check if a value is NaN. One of the most common ways is to use the isna()
method. The isna()
method returns a Boolean value True
if the value is NaN; otherwise, it returns False
.
To check if a single cell value is NaN in Pandas, you can use the following code:
import pandas as pd
# create a sample dataframe
data = {'Name': ['John', 'Doe', 'Mary', 'Jane'],
'Age': [25, 30, 35, 40],
'Salary': [50000, 60000, None, 70000]} # Note the NaN here
df = pd.DataFrame(data)
# check if the value in row 2 and column 'Salary' is NaN
print(pd.isna(df.at[2, 'Salary']))
In the above example, we create a sample dataframe with four rows and three columns. The ‘Salary’ column has a missing value or NaN in the third row. We then use the isna()
method to check if the value in row 2 and column ‘Salary’ is NaN. The code returns True
, indicating that the value is NaN.
Alternatively, you can use the isnull()
method instead of isna()
. The isnull()
method is an alias for isna()
and returns the same result. Both methods are interchangeable.
# check if the value in row 2 and column 'Salary' is NaN using isnull()
print(pd.isnull(df.at[2, 'Salary']))
Handling NaN Values in your Datasets
Now that we know how to check if a single cell value is NaN in Pandas, let’s explore how to handle NaN values in your datasets.
Drop NaN Values
One way to handle NaN values is to drop them from your dataset. You can use the dropna()
method to drop any row or column that contains a NaN value from your dataframe.
# drop any row that contains a NaN value
df.dropna(inplace=True)
# drop any column that contains a NaN value
df.dropna(axis=1, inplace=True)
In the above example, we use the dropna()
method to drop any row that contains a NaN value from our dataframe. We can also drop any column that contains a NaN value by setting the axis
parameter to 1.
Replace NaN Values
Another way to handle NaN values is to replace them with a specific value. You can use the fillna()
method to replace NaN values with a particular value.
# replace NaN values in the Salary column with 0
df['Salary'].fillna(0, inplace=True)
# replace NaN values in the Age column with the mean age
mean_age = df['Age'].mean()
df['Age'].fillna(mean_age, inplace=True)
In the above example, we use the fillna()
method to replace NaN values in the ‘Salary’ column with 0 and NaN values in the ‘Age’ column with the mean age.
Conclusion
In this article, we’ve discussed how to check if a single cell value is NaN in Pandas and why it’s essential to handle NaN values in your datasets. We’ve also explored two ways to handle NaN values in your datasets: dropping NaN values and replacing NaN values with a specific value.
By identifying and handling NaN values appropriately in your datasets, you can ensure accurate and reliable analysis, leading to better decision-making. Pandas provides an easy-to-use interface to manipulate and handle NaN values effectively, making it an essential tool in any data scientist or software engineer’s toolkit.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.