How to Check if a Particular Cell in Pandas DataFrame is Null
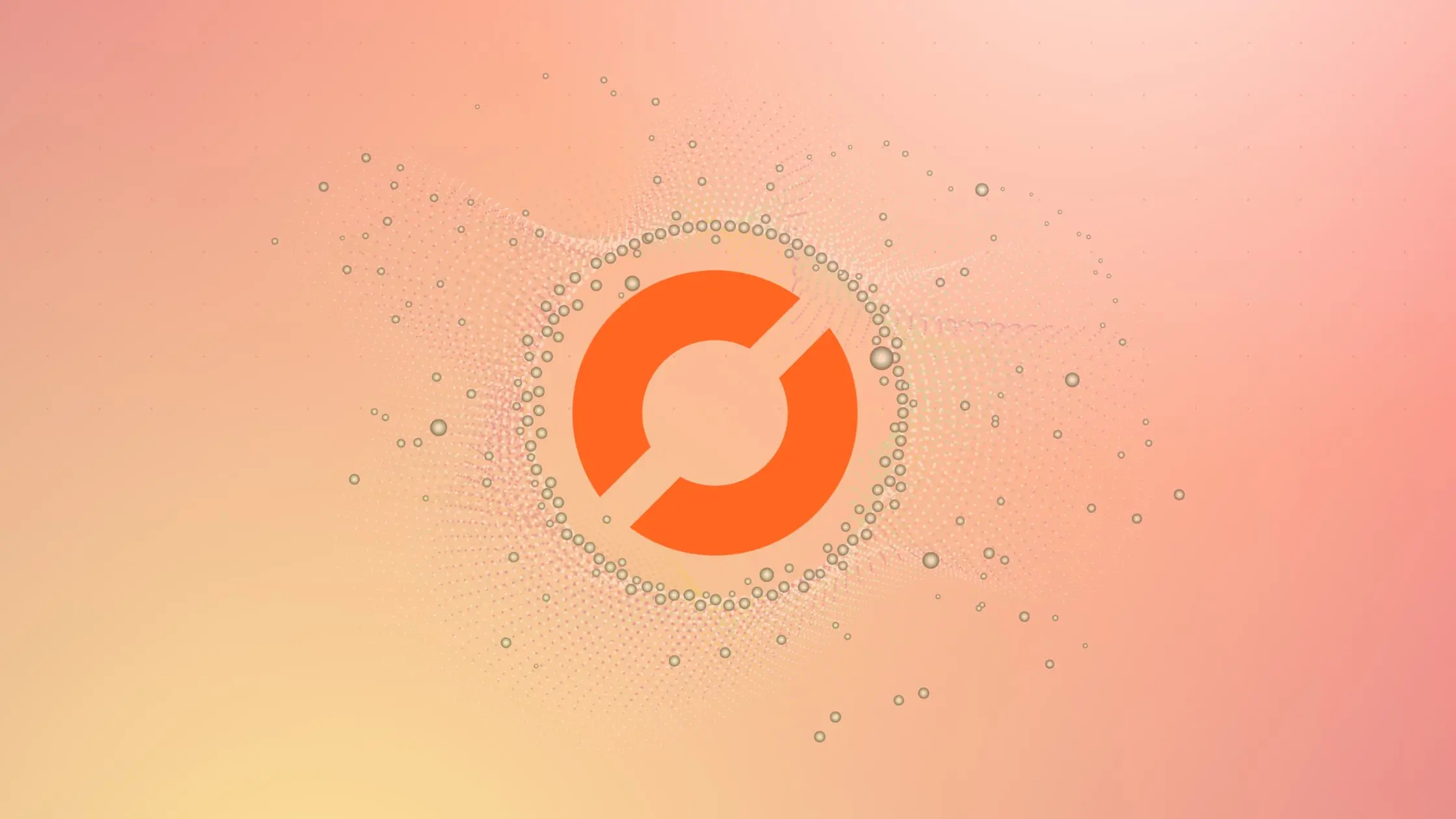
How to Check if a Particular Cell in Pandas DataFrame is Null
As a data scientist or software engineer, working with large datasets is a common task. Pandas is a popular data manipulation library in Python that simplifies the process of working with tabular data. However, one common problem that data scientists face is checking for missing values in their data. In this article, we will explore how to check if a particular cell in a pandas DataFrame is null.
What is a Pandas DataFrame?
Before we dive into how to check for null values, it is important to understand what a pandas DataFrame is. A DataFrame is a two-dimensional tabular data structure with rows and columns. Each column can have a different data type, such as integers, floats, or strings. A DataFrame is similar to a spreadsheet or SQL table, and it is a powerful tool for manipulating and analyzing data.
Checking for Null Values in Pandas DataFrame
Missing values in a DataFrame can be represented by NaN (Not a Number) or None. NaN is a special floating-point value that represents missing or undefined data. None is a Python object that represents missing or undefined data of any data type.
To check for null values in a pandas DataFrame, we can use the isnull()
method. The isnull()
method returns a DataFrame of the same shape as the input DataFrame, but with boolean values indicating whether each cell is null or not.
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({'col1': [1, 2, None], 'col2': [3, None, 5]})
# check for null values
null_df = df.isnull()
print(null_df)
Output:
col1 col2
0 False False
1 False True
2 True False
In the example above, we created a DataFrame with two columns, col1
and col2
, and three rows. We used the isnull()
method to check for null values in the DataFrame, and the output is a DataFrame of the same shape as the input DataFrame, with boolean values indicating whether each cell is null or not.
We can also use the notnull()
method to check for non-null values in a DataFrame. The notnull()
method returns the opposite of the isnull()
method, a DataFrame of the same shape as the input DataFrame, but with boolean values indicating whether each cell is not null or not.
# check for non-null values
not_null_df = df.notnull()
print(not_null_df)
Output:
col1 col2
0 True True
1 True False
2 False True
In the example above, we used the notnull()
method to check for non-null values in the DataFrame, and the output is a DataFrame of the same shape as the input DataFrame, with boolean values indicating whether each cell is not null or not.
Accessing a Particular Cell in Pandas DataFrame
Now that we know how to check for null values in a pandas DataFrame, let’s explore how to access a particular cell in a DataFrame. We can use the iloc
method to access a particular cell in a DataFrame by its row and column index.
# access a particular cell
cell_value = df.iloc[1, 1]
print(cell_value)
Output:
nan
In the example above, we used the iloc
method to access the cell in the second row and second column of the DataFrame. The output is nan
, which stands for Not a Number, indicating that the value in that cell is null.
Checking if a Particular Cell is Null in Pandas DataFrame
To check if a particular cell in a pandas DataFrame is null, we can combine the methods we learned above. We can use the isnull()
method to check if a cell is null, and the iloc
method to access the cell by its row and column index.
# check if a particular cell is null
cell_is_null = df.isnull().iloc[1, 1]
print(cell_is_null)
Output:
True
In the example above, we used the isnull()
method to check if the cell in the second row and second column of the DataFrame is null, and the iloc
method to access the cell by its row and column index. The output is True
, indicating that the value in that cell is null.
Conclusion
Checking for null values in a pandas DataFrame is a common task in data science and software engineering. In this article, we explored how to check if a particular cell in a pandas DataFrame is null. We learned how to use the isnull()
and notnull()
methods to check for null and non-null values in a DataFrame, and how to use the iloc
method to access a particular cell in a DataFrame by its row and column index. By combining these methods, we can easily check if a particular cell in a pandas DataFrame is null, and use this information to clean, transform, or analyze our data.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.