How to Check for Duplicate Values in Pandas DataFrame Column
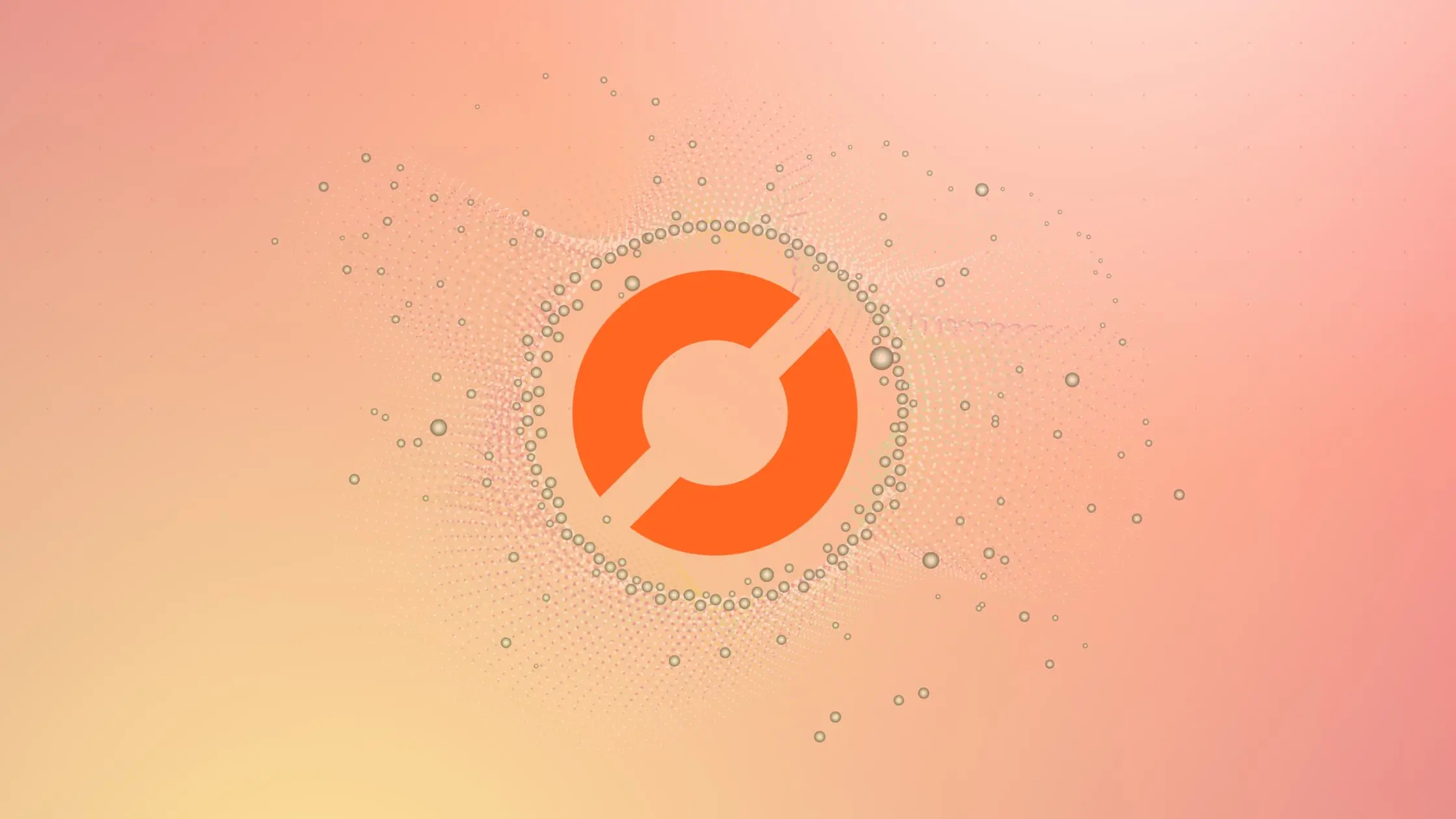
As a data scientist or software engineer, you may often work with large datasets that contain numerous rows and columns. In such cases, it is common to encounter duplicate values in a particular column of a Pandas DataFrame. Duplicate values can be problematic as they can skew your analysis and lead to inaccurate results. Therefore, it is essential to identify and remove them from your dataset.
In this article, we will explore how you can check for duplicate values in Pandas DataFrame column and how to deal with them.
What is Pandas DataFrame?
Before we dive into the details of identifying duplicate values, let’s first understand what Pandas DataFrame is.
Pandas is a popular open-source data manipulation library for Python. It provides data structures such as Series and DataFrame, which allow you to work with structured data efficiently. A DataFrame is a two-dimensional table-like data structure that consists of rows and columns. It is similar to a spreadsheet or a SQL table.
Identifying Duplicate Values in DataFrame Column
To check for duplicate values in a Pandas DataFrame column, you can use the duplicated()
method. This method returns a boolean Series that indicates whether a row is a duplicate or not.
Let’s consider an example where we have a DataFrame that contains information about customers and their order history.
import pandas as pd
# create a sample DataFrame
data = {'Product': ['Laptop', 'Mouse', 'Keyboard', 'Monitor', 'Laptop', 'Headphones'],
'Price': [800, 20, 30, 300, 800, 50]}
df = pd.DataFrame(data)
Output:
Product Price
0 Laptop 800
1 Mouse 20
2 Keyboard 30
3 Monitor 300
4 Laptop 800
5 Headphones 50
Now, let’s say we want to check for duplicate values in the Product
column. We can accomplish this by calling the duplicated()
method on the DataFrame and passing the column name as an argument.
# check for duplicate values in ProductID column
duplicate_values = df['Product'].duplicated()
print(duplicate_values)
This will return a boolean Series indicating whether a row in the ProductID
column is a duplicate or not.
0 False
1 False
2 False
3 False
4 True
5 False
Name: Product, dtype: bool
In this case, we can see that there is one duplicate values in the Product
column at row 4.
Removing Duplicate Values
Once you have identified the duplicate values in your DataFrame column, you may want to remove them to avoid any issues in your analysis. You can use the drop_duplicates()
method to remove the duplicate rows from your DataFrame.
Let’s say we want to remove the duplicate rows from the Product
column in our example DataFrame. We can call the drop_duplicates()
method on the column to remove the duplicate rows.
# remove duplicate values in ProductID column
df = df.drop_duplicates(subset=['Product'], keep='first')
print(df)
This will remove the duplicate rows and return the updated DataFrame.
Product Price
0 Laptop 800
1 Mouse 20
2 Keyboard 30
3 Monitor 300
5 Headphones 50
In this case, we can see that the duplicate row with Product
named Laptop
has been removed.
Conclusion
In this article, we discussed how you can check for duplicate values in a Pandas DataFrame column and remove them to avoid any issues in your analysis. We used the duplicated()
method to identify the duplicate rows and the drop_duplicates()
method to remove them.
It is essential to identify and remove duplicate values from your dataset as they can skew your analysis and lead to inaccurate results. By following the steps outlined in this article, you can ensure that your data is clean and accurate, which is essential for any data analysis or machine learning task.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.