How to Change Index Value in Pandas Dataframe
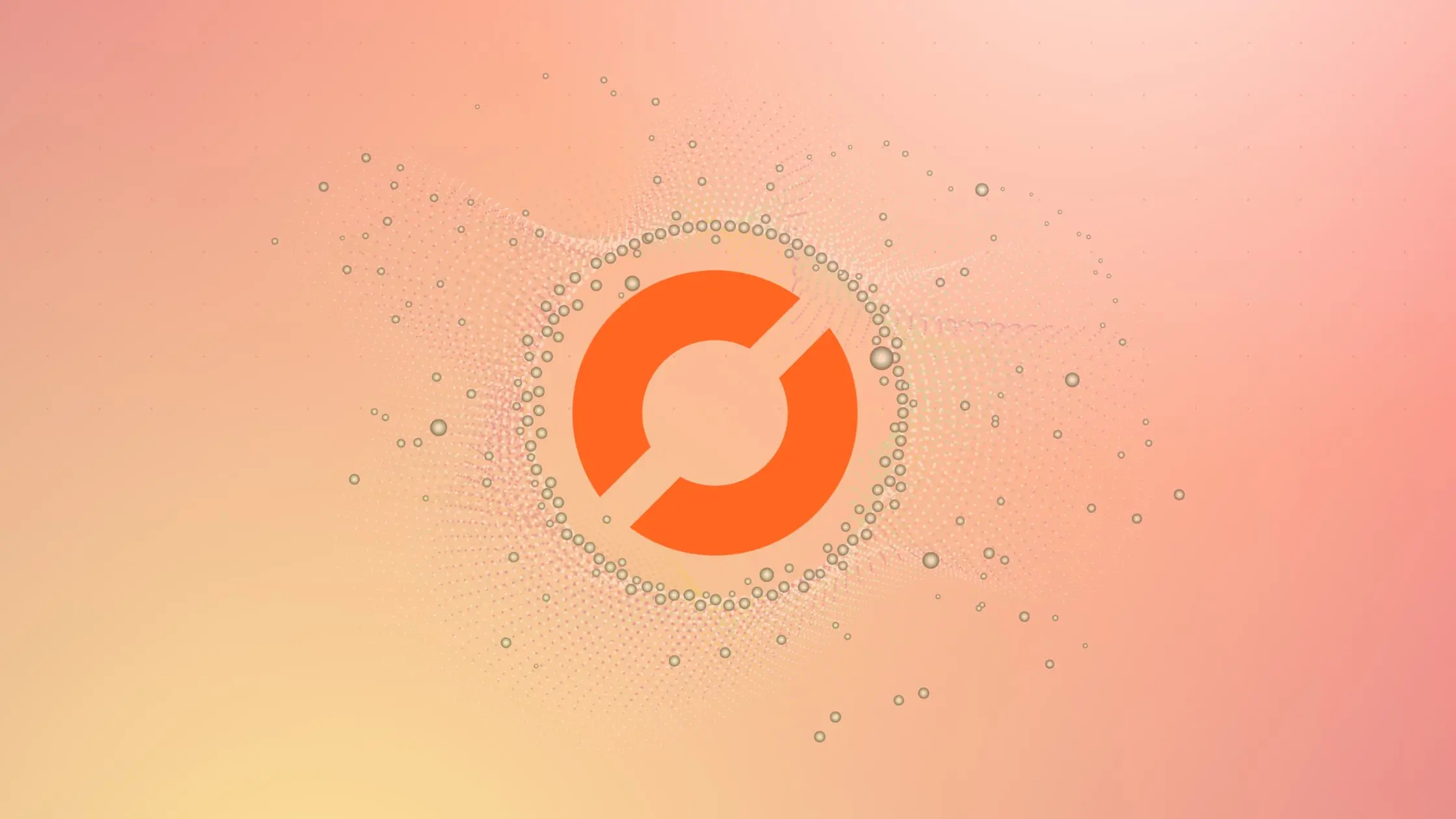
As a data scientist or software engineer, working with data is a common task, and Pandas is a powerful and popular tool for data manipulation in Python. One of the common operations in Pandas is changing the index value of a dataframe. In this article, we will explore the different methods to change the index value in Pandas dataframe.
Table of Contents
- What is a Pandas Dataframe?
- Why Change Index Value in Pandas Dataframe?
- How to Change Index Value in Pandas Dataframe
- Common Errors and Solutions
- Conclusion
What is a Pandas Dataframe?
A Pandas dataframe is a 2-dimensional labeled data structure with columns of potentially different types. In other words, it is a table with rows and columns, where each column can have a different datatype. The dataframe has an index, which is a unique identifier for each row.
Why Change Index Value in Pandas Dataframe?
The index value can be used to select, filter, and join data in Pandas. Sometimes the index value might not be suitable for our analysis, or we might need to change it to make it more meaningful. For example, we might need to change the index value to a date-time format, or we might need to change it to a categorical variable.
How to Change Index Value in Pandas Dataframe
There are different methods to change the index value in Pandas dataframe. We will explore them one by one.
Use set_index()
Method
The set_index()
method is used to set the index of a Pandas dataframe. We can pass the name of the column to be used as the index to the set_index()
method. The following code demonstrates how to use the set_index()
method to change the index value:
import pandas as pd
# create a dataframe
df = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 30, 35, 40],
'city': ['New York', 'Paris', 'London', 'Tokyo']
})
# set the index to the 'name' column
df.set_index('name', inplace=True)
print(df)
In the above code, we created a dataframe with three columns. We then used the set_index()
method to set the index to the name
column. The inplace=True
parameter is used to modify the dataframe in place, without creating a new dataframe.
Output:
age city
name
Alice 25 New York
Bob 30 Paris
Charlie 35 London
David 40 Tokyo
Use reset_index()
Method
The reset_index()
method is used to reset the index of a Pandas dataframe to the default integer index. The following code demonstrates how to use the reset_index()
method to reset the index value:
import pandas as pd
# create a dataframe
df = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 30, 35, 40],
'city': ['New York', 'Paris', 'London', 'Tokyo']
})
# set the index to the 'name' column
df.set_index('name', inplace=True)
print(df)
print("------------")
# reset the index
df.reset_index(inplace=True)
print(df)
In the above code, we first set the index to the name
column using the set_index()
method. We then used the reset_index()
method to reset the index to the default integer index. The inplace=True
parameter is used to modify the dataframe in place, without creating a new dataframe.
Output:
age city
name
Alice 25 New York
Bob 30 Paris
Charlie 35 London
David 40 Tokyo
------------
name age city
0 Alice 25 New York
1 Bob 30 Paris
2 Charlie 35 London
3 David 40 Tokyo
Use rename()
Method
The rename()
method is used to rename the index labels of a Pandas dataframe. We can pass a dictionary with the old index label as the key and the new index label as the value to the rename()
method. The following code demonstrates how to use the rename()
method to change the index value:
import pandas as pd
# create a dataframe
df = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [25, 30, 35, 40],
'city': ['New York', 'Paris', 'London', 'Tokyo']
})
# set the index to the 'name' column
df.set_index('name', inplace=True)
# rename the index label
df.rename(index={'Alice': 'Alicia'}, inplace=True)
print(df)
In the above code, we first set the index to the name
column using the set_index()
method. We then used the rename()
method to rename the index label from Alice
to Alicia
. The inplace=True
parameter is used to modify the dataframe in place, without creating a new dataframe.
Output:
age city
name
Alicia 25 New York
Bob 30 Paris
Charlie 35 London
David 40 Tokyo
Common Errors and Solutions
Error 1: “Index not found."
This error occurs when the specified index column is not present in the DataFrame.
- Solution: Ensure the column name is spelled correctly and exists in the DataFrame.
# Example Solution
df.set_index('Nonexistent_Column') # Replace 'Nonexistent_Column' with the correct column name
Error 2: “Cannot set a frame with no defined index and a value that cannot be converted to a DataFrame."
This error occurs when attempting to set an index with a value that cannot be converted to a DataFrame.
- Solution: Verify that the new index is a valid column or array and can be converted to a DataFrame.
# Example Solution
df.set_index([1, 2, 3]) # Replace [1, 2, 3] with a valid index
Error 3: “The new index length must be the same as the old index length."
This error occurs when the length of the new index does not match the length of the old index.
- Solution: Ensure the new index has the same length as the old index.
# Example Solution
df.set_index(['Alice', 'Bob']) # Replace ['Alice', 'Bob'] with an index of the same length
Conclusion
In this article, we explored the different methods to change the index value in Pandas dataframe. We learned that we can use the set_index()
method to set the index, the reset_index()
method to reset the index to the default integer index, and the rename()
method to rename the index label. These methods are useful for data manipulation and analysis, and can help us make our data more meaningful and useful.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.