How to Change Datetime Format in Pandas
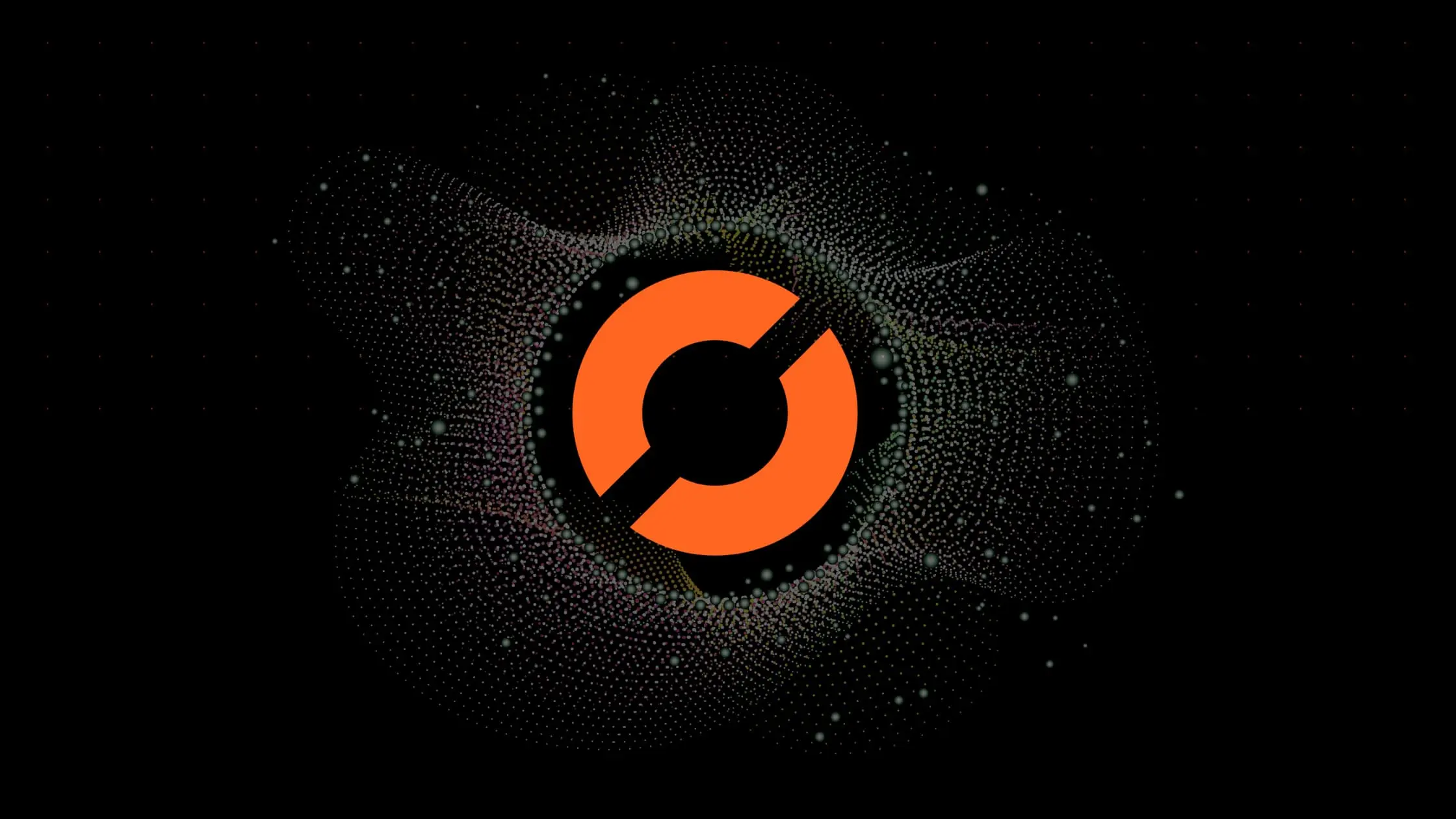
How to Change Datetime Format in Pandas
As a data scientist or software engineer, you may often find yourself working with date and time data in your projects. One of the most popular tools for working with such data in Python is the Pandas library. Pandas provides a powerful set of tools for manipulating and analyzing data, including the ability to easily change the format of datetime data.
In this article, we will show you how to change the datetime format in Pandas, including some common datetime formats that you may encounter in your data.
What is Datetime in Pandas?
Before diving into how to change the datetime format in Pandas, let’s first briefly discuss what datetime is in Pandas. In Pandas, datetime is a data type that represents dates and times. It is a combination of a date and a time, and can be represented in a variety of formats.
Pandas provides a number of tools for working with datetime data, including the ability to parse datetime strings, create datetime objects from scratch, and perform various calculations and manipulations on datetime data.
How to Change Datetime Format in Pandas
To change the datetime format in Pandas, you first need to have a Pandas DataFrame or Series that contains datetime data. Once you have your data loaded into Pandas, you can use the strftime()
method to format the datetime data in the desired format.
The strftime()
method takes a string argument that specifies the desired format for the datetime data. The string argument contains various format codes that are replaced with the corresponding values from the datetime data.
Here is an example of how to use strftime()
to change the datetime format in Pandas:
import pandas as pd
# create a DataFrame with datetime data
df = pd.DataFrame({'date': ['2022-06-01 12:00:00', '2022-06-02 13:00:00', '2022-06-03 14:00:00']})
# convert the date column to datetime format
df['date'] = pd.to_datetime(df['date'])
# change the datetime format
df['date_formatted'] = df['date'].dt.strftime('%Y/%m/%d %H:%M:%S')
print(df)
In this example, we first create a DataFrame with a column called “date” that contains datetime strings in the format “YYYY-MM-DD HH:MM:SS”. We then convert this column to datetime format using the pd.to_datetime()
method.
Finally, we use the dt.strftime()
method to change the datetime format to “YYYY/MM/DD HH:MM:SS” and store the result in a new column called “date_formatted”. The resulting DataFrame looks like this:
date date_formatted
0 2022-06-01 12:00:00 2022/06/01 12:00:00
1 2022-06-02 13:00:00 2022/06/02 13:00:00
2 2022-06-03 14:00:00 2022/06/03 14:00:00
Note that the format codes used in the strftime()
method are case-sensitive. For example, “Y” represents the year with a century, while “y” represents the year without a century. The full list of format codes can be found in the Python documentation.
Common Datetime Formats
Here are some common datetime formats that you may encounter in your data, along with their corresponding format codes for use with the strftime()
method:
Datetime Format | Format Code |
---|---|
YYYY-MM-DD HH:MM:SS | %Y-%m-%d %H:%M:%S |
YYYY/MM/DD HH:MM:SS | %Y/%m/%d %H:%M:%S |
MM/DD/YYYY HH:MM:SS | %m/%d/%Y %H:%M:%S |
DD/MM/YYYY HH:MM:SS | %d/%m/%Y %H:%M:%S |
YYYY-MM-DD | %Y-%m-%d |
YYYY/MM/DD | %Y/%m/%d |
MM/DD/YYYY | %m/%d/%Y |
DD/MM/YYYY | %d/%m/%Y |
These are just a few examples of the many datetime formats that you may encounter in your data. If you are unsure of the format of your datetime data, you can use the pd.to_datetime()
method with the infer_datetime_format=True
parameter to automatically infer the format.
Conclusion
In this article, we have shown you how to change the datetime format in Pandas using the strftime()
method. We have also provided some common datetime formats and their corresponding format codes.
Working with datetime data in Pandas can be a powerful tool for data analysis and manipulation. By understanding how to change the datetime format in Pandas, you can more easily work with datetime data in your projects.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.