How to Calculate Weighted Average Using Pandas DataFrame
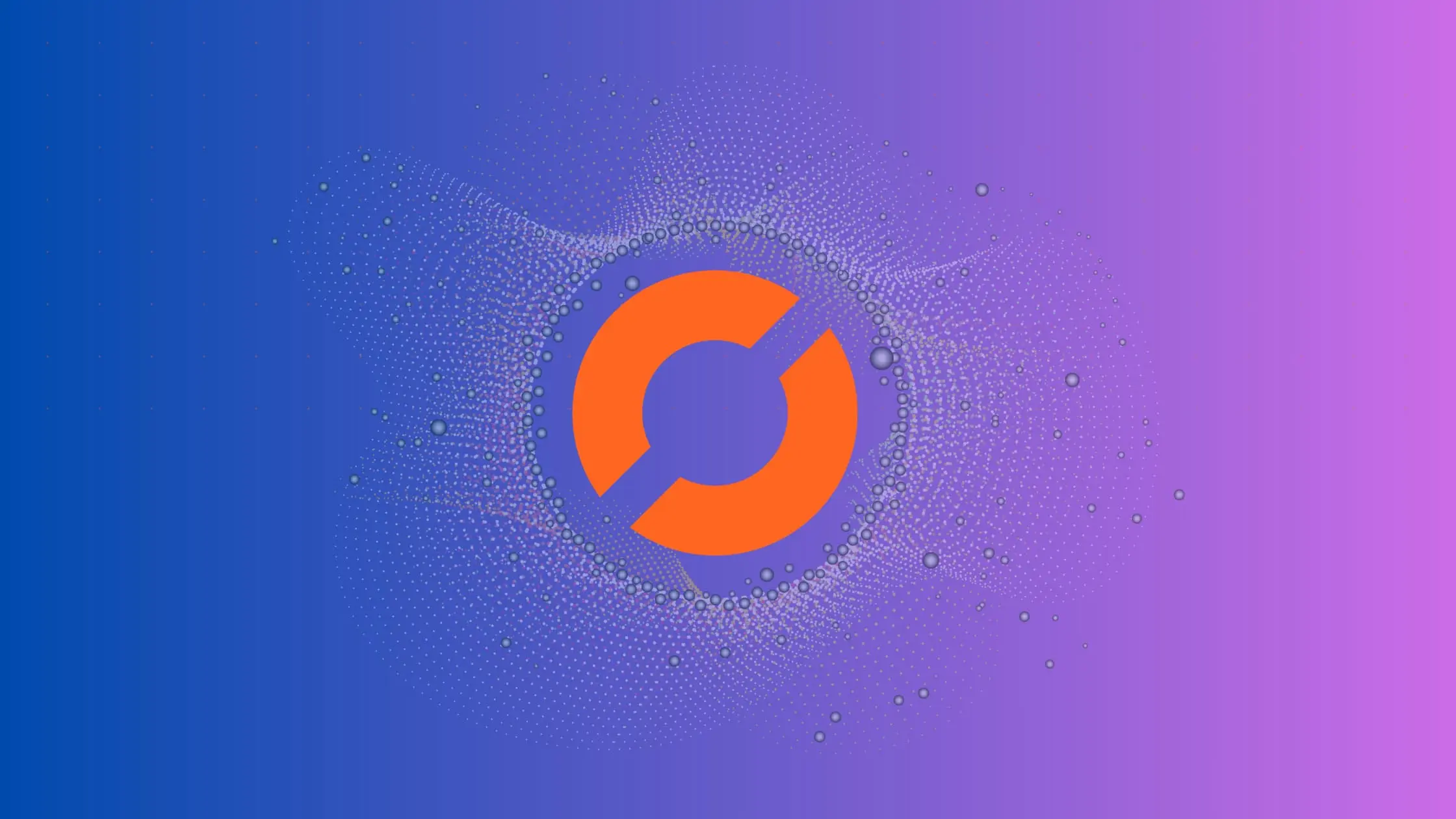
As a data scientist or software engineer, you may encounter situations where you need to calculate a weighted average of a dataset. Weighted average is a type of average where each data point is multiplied by a weight factor, and the sum of all these products is divided by the sum of the weights.
In this blog post, we will discuss how to calculate a weighted average using Pandas DataFrame. Pandas is a widely-used data manipulation library in Python that provides functionality to work with tabular data.
Table of Contents
- Introduction
- What Is Pandas DataFrame?
- How to Calculate Weighted Average Using Pandas DataFrame?
- Practical Applications of Weighted Averages
- Pros and Cons of Calculating Weighted Averages Using Pandas DataFrame
- Error Handling
- Conclusion
What Is Pandas DataFrame?
A Pandas DataFrame is a two-dimensional, size-mutable, and heterogeneously-typed tabular data structure with labeled axes (rows and columns). It is similar to a spreadsheet or a SQL table, where each column can have a different data type (e.g., integer, float, string, etc.).
How to Calculate Weighted Average Using Pandas DataFrame?
Let’s assume that we have a DataFrame with two columns: “Values” and “Weights”. The “Values” column contains the data points, and the “Weights” column contains the corresponding weight factors for each data point.
Here’s an example DataFrame:
import pandas as pd
data = {'Values': [10, 20, 30, 40, 50],
'Weights': [0.1, 0.2, 0.3, 0.2, 0.2]}
df = pd.DataFrame(data)
To calculate the weighted average of this DataFrame, we can use the following formula:
weighted_average = sum(df['Values'] * df['Weights']) / sum(df['Weights'])
Let’s break down this formula:
df['Values'] * df['Weights']
multiplies each value in the “Values” column by the corresponding weight factor in the “Weights” column, resulting in a new Series.sum(df['Values'] * df['Weights'])
calculates the sum of all the products in the new Series, which gives us the numerator of the weighted average formula.sum(df['Weights'])
calculates the sum of all the weight factors in the “Weights” column, which gives us the denominator of the weighted average formula.sum(df['Values'] * df['Weights']) / sum(df['Weights'])
divides the numerator by the denominator, giving us the weighted average.
In our example DataFrame, the weighted average would be:
weighted_average = sum(df['Values'] * df['Weights']) / sum(df['Weights'])
print(weighted_average)
Output:
32.0
Therefore, the weighted average of the “Values” column with corresponding weights in the “Weights” column is 32.0.
Practical Applications of Weighted Averages
Calculating a weighted average is useful in various scenarios, especially when dealing with datasets where certain data points contribute more or less to the overall average based on their significance or relevance. Here are some common scenarios where calculating a weighted average is beneficial:
Financial Data: When calculating the average return on investment, where each investment may have a different weight based on its initial amount or the time it was held. In portfolio management, where assets with different weights contribute to the overall performance.
Survey Responses: When analyzing survey data where each response category may have a different weight based on its significance or relevance to the research question.
Product Reviews and Ratings: When calculating a weighted average user rating for a product, where each review has a different weight, perhaps based on the credibility of the reviewer or the number of reviews.
Employee Performance Evaluations: When calculating the overall performance score for employees, considering different performance metrics with varying importance, and each metric having a different weight.
Supply Chain and Inventory Management: In scenarios where different products have different economic impacts, such as calculating a weighted average cost of goods sold (COGS) or average inventory cost.
Health and Biomedical Research: When analyzing health data, such as calculating a weighted average patient recovery rate, where the weight may represent the severity of the condition.
Statistical Aggregations: When summarizing data for different regions, departments, or segments, where each segment has a different weight based on its size or significance.
Population Demographics: In demographic studies, when calculating a weighted average age, income, or other demographic factors where each subgroup has a different weight.
Resource Allocation: When distributing resources or budgets among different departments or projects, where the importance or priority of each area determines its weight in the overall allocation.
In essence, the need for calculating a weighted average arises when individual data points contribute differently to the overall average based on their relative importance or relevance in a specific context. This allows for a more nuanced and accurate representation of the underlying data, taking into account the varying impacts of different elements within a dataset.
Pros and Cons of Calculating Weighted Averages Using Pandas DataFrame
Pros
Precision and Accuracy: Weighted averages provide a more accurate representation of the dataset by accounting for the varying importance of each data point.
Flexibility in Data Analysis: Pandas allows for flexibility in handling complex weighting schemes, enabling data scientists to adapt to diverse scenarios.
Efficiency with Large Datasets: Pandas' optimized implementation results in efficient calculations, making it suitable for handling large datasets without sacrificing performance.
Integration with Data Manipulation Tools: Seamless integration with other Python libraries allows for a comprehensive data analysis workflow, incorporating data cleaning, transformation, and exploration.
Reproducibility and Documentation: The use of Pandas enhances transparency, making it easier to create reproducible data analysis pipelines and document the entire process.
Interactivity in Jupyter Notebooks: Pandas integrates well with Jupyter Notebooks, providing an interactive environment for exploring intermediate results and iterating through analyses.
Cons
Learning Curve for Beginners: Beginners may experience a learning curve when getting acquainted with Pandas syntax and functionality.
Potential for Code Complexity: Complex weighting scenarios may lead to intricate code, potentially making it harder to maintain and understand.
Resource Intensity: While Pandas is efficient, extremely large datasets may still require substantial computational resources.
Dependence on Data Quality: Weighted averages are sensitive to the quality of input data, and anomalies or errors in the data can impact the accuracy of the results.
Error Handling
Handling Missing Values: Before calculating a weighted average, ensure that missing values in the relevant columns are appropriately addressed, as they can lead to inaccurate results.
Verification of Data Types: Verify that the data types of the columns involved in the calculation are compatible to avoid unexpected errors.
Check for Division by Zero: Implement checks to handle situations where the denominator (sum of weights) becomes zero, preventing division errors.
Validation of Input Columns: Validate that the DataFrame contains the necessary columns (“Values” and “Weights”) to avoid key errors during the calculation.
Handling Negative Weights: Depending on the context, consider implementing logic to handle scenarios where negative weights might lead to unintended results.
Testing and Validation: Before deploying the code in a production environment, thoroughly test the weighted average calculation with diverse datasets to catch any potential issues.
Conclusion
In conclusion, calculating a weighted average using Pandas DataFrame is a simple process that can be performed using a few lines of code. By multiplying each data point with its corresponding weight factor and dividing the sum of these products by the sum of the weights, we can obtain the weighted average of a dataset.
Pandas DataFrame provides a powerful and flexible way to manipulate and analyze tabular data. As a data scientist or software engineer, it is essential to be familiar with the various functionalities provided by Pandas, including calculating weighted averages.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.