How to Calculate the Time Difference Between Two Consecutive Rows in Pandas
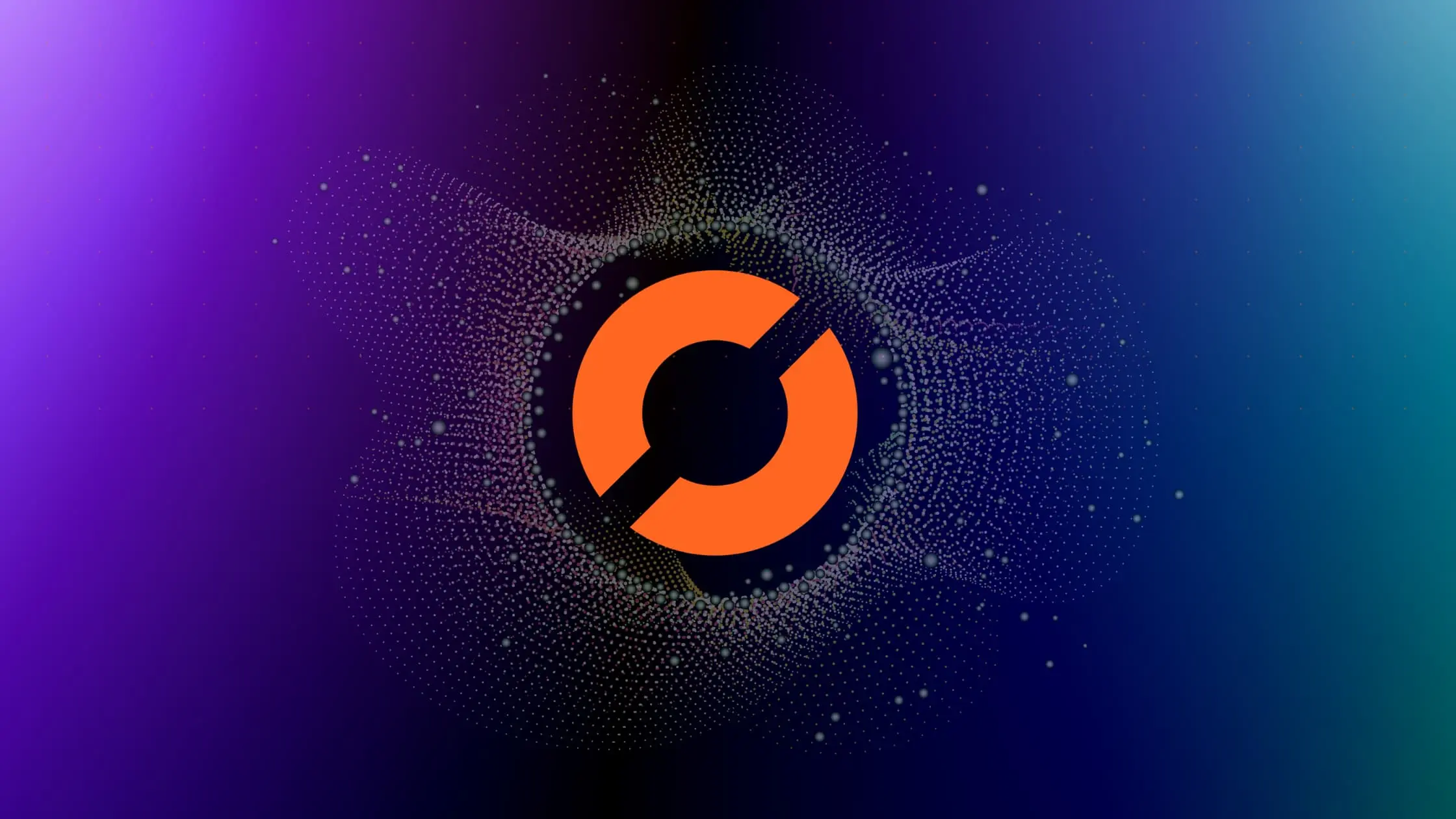
As a data scientist or software engineer, you may come across a situation where you need to calculate the time difference between two consecutive rows in a pandas DataFrame. This can be a challenging task, especially when dealing with large datasets. In this article, we will explore how to calculate the time difference between two consecutive rows in pandas.
What is Pandas?
Pandas is a popular open-source Python library used for data manipulation and analysis. It provides data structures for efficiently storing and manipulating large datasets, including data frames and series. Pandas is widely used in data science and machine learning applications.
The Problem
Suppose you have a pandas DataFrame that contains a timestamp column. You want to calculate the time difference between two consecutive rows in this DataFrame. For example, you have a DataFrame that contains the timestamps of user logins, and you want to calculate the time difference between each login.
The Solution
To solve this problem, we can use pandas diff()
function. The diff()
function calculates the difference between two consecutive rows in a DataFrame. We can use this function to calculate the time difference between consecutive timestamps in a DataFrame.
Here’s an example of how to use the diff()
function to calculate the time difference between two consecutive rows in a pandas DataFrame:
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({
'timestamp': ['2022-06-17 10:00:00', '2022-06-17 11:00:00', '2022-06-17 12:00:00', '2022-06-17 13:00:00'],
'value': [1, 2, 3, 4]
})
# convert the timestamp column to datetime format
df['timestamp'] = pd.to_datetime(df['timestamp'])
# calculate the time difference between consecutive rows
df['time_diff'] = df['timestamp'].diff()
print(df)
In this example, we create a sample DataFrame with a timestamp column and a value column. We convert the timestamp column to datetime format using pandas' to_datetime()
function. Then we use the diff()
function to calculate the time difference between consecutive timestamps and store the result in a new column called time_diff
.
The output of this code will be:
timestamp value time_diff
0 2022-06-17 10:00:00 1 NaT
1 2022-06-17 11:00:00 2 01:00:00
2 2022-06-17 12:00:00 3 01:00:00
3 2022-06-17 13:00:00 4 01:00:00
As you can see, the diff()
function calculates the time difference between consecutive timestamps and stores the result in the time_diff
column. The first row of the time_diff
column is NaT
(Not a Time), which indicates that there is no time difference between the first and second rows.
Dealing with Missing Values
In some cases, the diff()
function may return missing values (NaN) if there is a missing value in the original DataFrame. To deal with missing values, we can use the fillna()
function to replace them with a default value.
Here’s an example of how to use the fillna()
function to replace missing values with a default value:
import pandas as pd
# create a sample DataFrame with missing values
df = pd.DataFrame({
'timestamp': ['2022-06-17 10:00:00', '2022-06-17 11:00:00', '2022-06-17 12:00:00', '2022-06-17 13:00:00'],
'value': [1, 2, 3, 4]
})
# add a missing value to the timestamp column
df.loc[1, 'timestamp'] = None
# convert the timestamp column to datetime format
df['timestamp'] = pd.to_datetime(df['timestamp'])
# calculate the time difference between consecutive rows
df['time_diff'] = df['timestamp'].diff()
print(df)
Output:
timestamp value time_diff
0 2022-06-17 10:00:00 1 NaT
1 NaT 2 NaT
2 2022-06-17 12:00:00 3 NaT
3 2022-06-17 13:00:00 4 0 days 01:00:00
In this example, we create a sample DataFrame with a missing value in the timestamp column. After using pandas diff()
function, it yeilds NaT
values as show above. To solve this problem, we can use the fillna()
function to replace missing values with a default value of 0 seconds.
# replace missing values with a default value
df['time_diff'] = df['time_diff'].fillna(pd.Timedelta(seconds=0))
print(df)
Output:
timestamp value time_diff
0 2022-06-17 10:00:00 1 0 days 00:00:00
1 NaT 2 0 days 00:00:00
2 2022-06-17 12:00:00 3 0 days 00:00:00
3 2022-06-17 13:00:00 4 0 days 01:00:00
As you can see, the missing value in the timestamp column is replaced with a default value of 0 seconds.
Conclusion
In this article, we have explored how to calculate the time difference between two consecutive rows in a pandas DataFrame. We have learned how to use the diff()
function to calculate the time difference between consecutive timestamps and how to deal with missing values using the fillna()
function. By using these techniques, you can efficiently calculate the time difference between consecutive rows in a pandas DataFrame and perform various time-based analyses.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.