How to Avoid PythonPandas Creating an Index in a Saved CSV
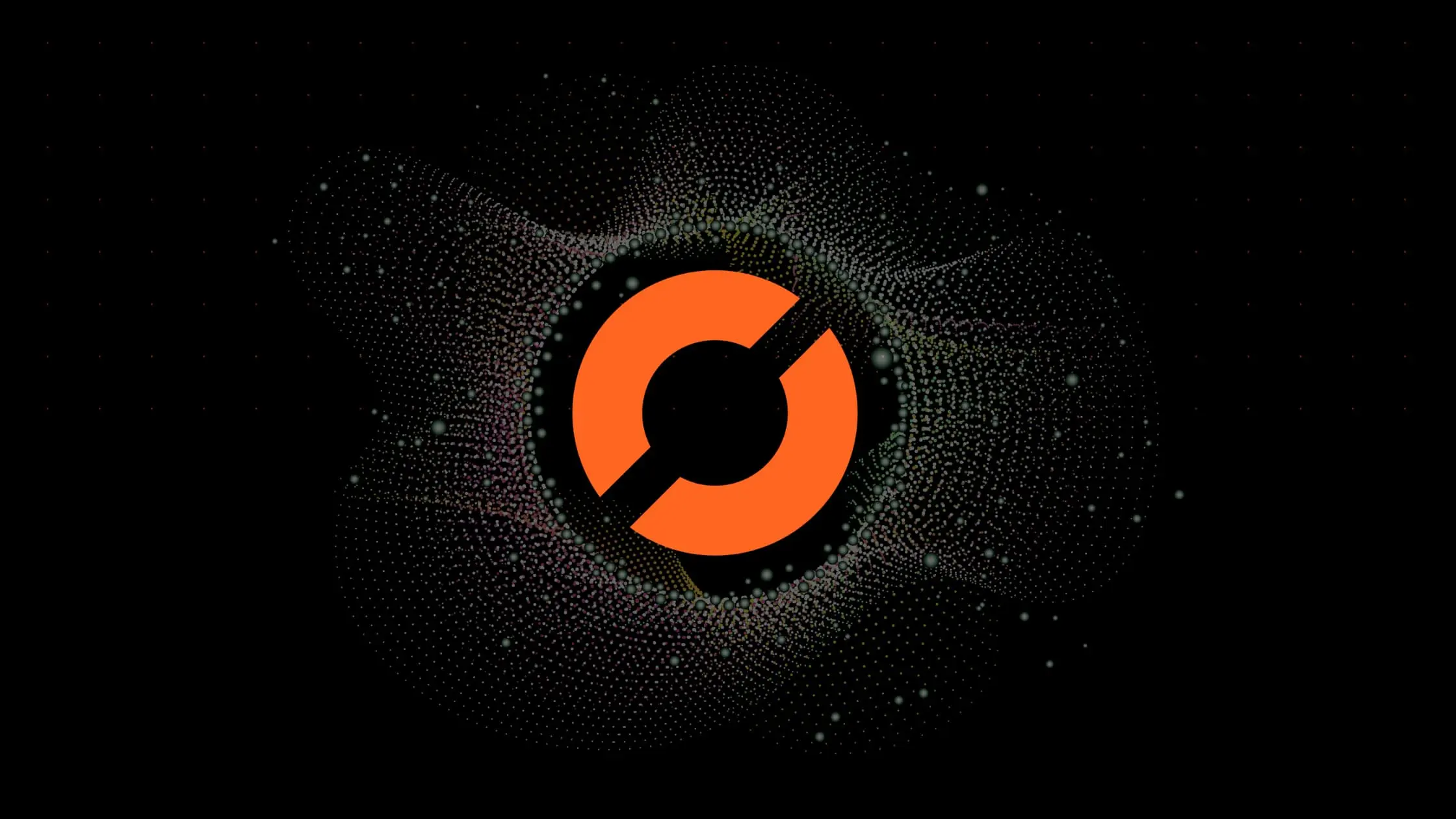
As a data scientist or software engineer, you might have encountered a situation where you need to save a Pandas DataFrame to a CSV file without the index. Pandas is a powerful library for data manipulation, but sometimes it can be frustrating when it automatically creates an index when saving a DataFrame to a CSV file. In this blog post, we will explore how to avoid Python/Pandas creating an index in a saved CSV.
What is an Index in Pandas?
An index in Pandas is a way to uniquely identify each row in a DataFrame. By default, Pandas creates an index with integers starting from 0. You can also set a column as an index if it provides a unique identifier for each row. An index is useful when you need to select, filter, or merge rows based on their position or label.
Why Avoid an Index in a Saved CSV?
When you save a Pandas DataFrame to a CSV file, the index is also saved by default. While this might be useful in some cases, it can cause problems in others. For example, if you have a large DataFrame with a complex index, the saved CSV file can become bloated and slow to load. Moreover, if you later read the CSV file back into a DataFrame, the index might not be useful or even invalid.
Therefore, it is often a good idea to save a DataFrame to a CSV file without the index, especially if you only need to store the data and not the index.
How to Save a DataFrame to CSV Without an Index
To save a Pandas DataFrame to a CSV file without the index, you can use the to_csv()
method with the index
parameter set to False
. Here is an example:
import pandas as pd
# create a DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]}, index=['x', 'y', 'z'])
# save to CSV without the index
df.to_csv('data.csv', index=False)
In this example, we create a DataFrame with two columns and a custom index. Then, we save the DataFrame to a CSV file called data.csv
without the index by setting the index
parameter to False
.
How to Remove an Index from an Existing CSV File
If you have an existing CSV file with an index that you want to remove, you can use the read_csv()
method with the index_col
parameter to read the CSV file into a DataFrame with a specific column as the index. Then, you can save the DataFrame to a new CSV file without the index.
Here is an example:
import pandas as pd
# read CSV file with index
df = pd.read_csv('data.csv', index_col=0)
# save to new CSV without index
df.to_csv('data_no_index.csv', index=False)
In this example, we read a CSV file called data.csv
into a DataFrame with the first column as the index. Then, we save the DataFrame to a new CSV file called data_no_index.csv
without the index by setting the index
parameter to False
.
Conclusion
In this blog post, we have learned how to avoid Python/Pandas creating an index in a saved CSV. We have seen that an index in Pandas is useful for identifying rows, but it can cause problems when saving a DataFrame to a CSV file. To save a DataFrame to a CSV file without the index, we can use the to_csv()
method with the index
parameter set to False
. If we have an existing CSV file with an index, we can remove the index by reading the CSV file into a DataFrame with a specific column as the index and then saving the DataFrame to a new CSV file without the index.
By following these simple steps, we can avoid the frustration of having Python/Pandas create an index in a saved CSV file and ensure that our data is stored efficiently and effectively.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.