How to apply a function to a specific column of a pandas DataFrame
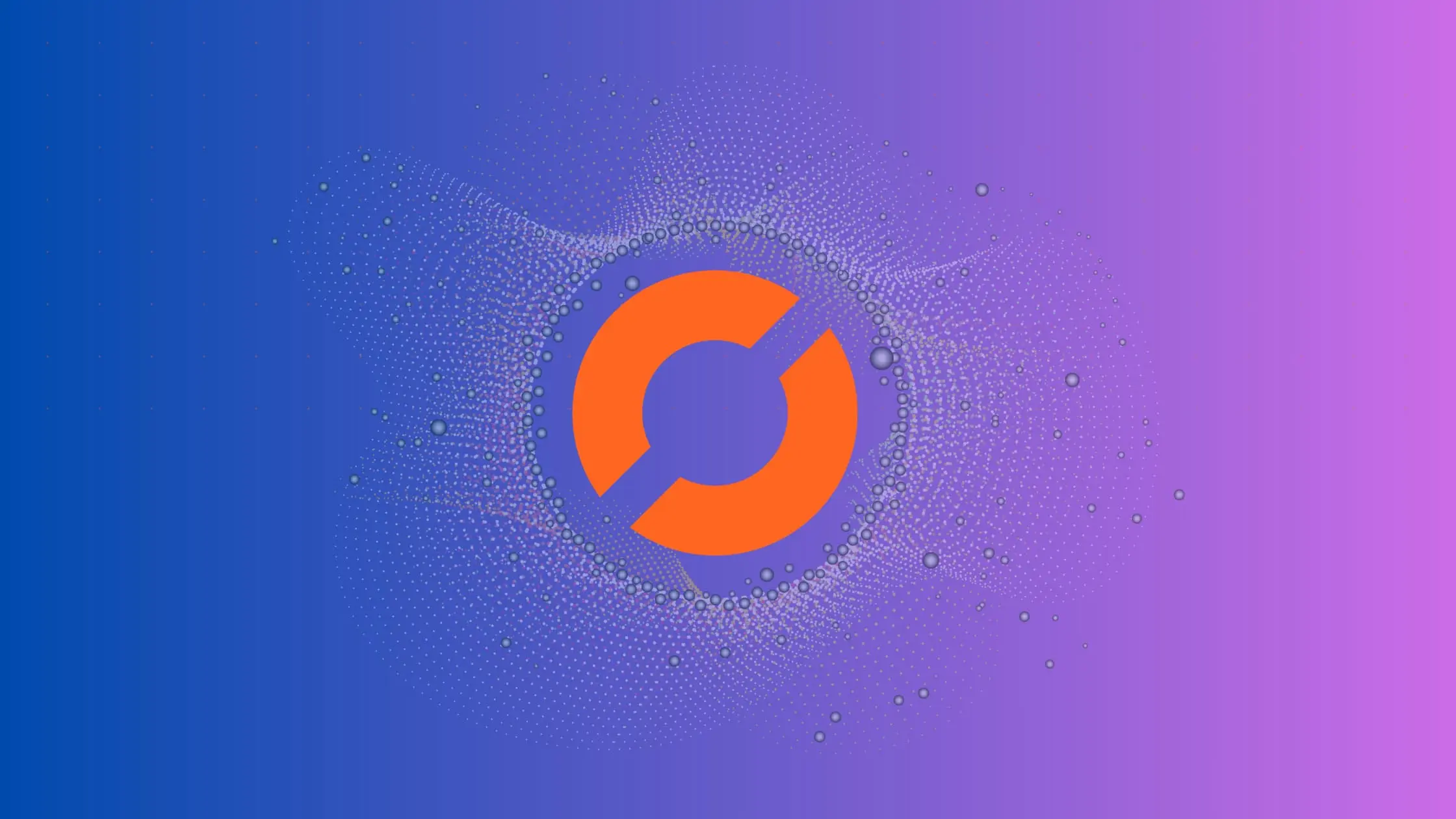
As a data scientist or software engineer, you are likely familiar with pandas, the Python library for data manipulation and analysis. One of the most commonly used data structures in pandas is the DataFrame, which is a two-dimensional table-like data structure with labeled rows and columns. In this article, we will explore how to apply a function to a specific column of a pandas DataFrame.
Table of Contents
- Introduction
- What is a pandas DataFrame?
- How to apply a function to a specific column in a pandas DataFrame
- Conclusion
What is a pandas DataFrame?
Before we dive into the topic of applying a function to a specific column in a pandas DataFrame, let’s first review what a pandas DataFrame is. As mentioned earlier, a DataFrame is a two-dimensional table-like data structure with labeled rows and columns. Each column in a DataFrame can have a different data type (e.g., integer, string, boolean, etc.), and each row represents a unique observation or record.
A pandas DataFrame can be created from a variety of sources, including CSV files, Excel spreadsheets, SQL databases, and more. Once a DataFrame is created, you can perform a wide range of data manipulation and analysis operations on it, such as filtering, grouping, sorting, and more.
How to apply a function to a specific column in a pandas DataFrame
Now that we have a basic understanding of what a pandas DataFrame is, let’s explore how to apply a function to a specific column in a DataFrame. There are several ways to accomplish this task, but one of the most common methods is to use the apply()
method in pandas.
The apply()
method in pandas allows you to apply a function to each element in a DataFrame or to each column or row in a DataFrame. To apply a function to a specific column in a DataFrame, you can pass the name of the column as an argument to the apply()
method.
Here’s an example of how to use the apply()
method to apply a function to a specific column in a pandas DataFrame:
import pandas as pd
# create a sample DataFrame
df = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie'],
'age': [25, 30, 35],
'salary': [50000, 60000, 70000]
})
# define a function to apply to the salary column
def salary_increase(salary):
return salary * 1.1
# apply the function to the salary column using apply()
df['salary'] = df['salary'].apply(salary_increase)
# print the updated DataFrame
print(df)
Output:
name age salary
0 Alice 25 55000.0
1 Bob 30 66000.0
2 Charlie 35 77000.0
In this example, we first create a sample DataFrame with three columns: name
, age
, and salary
. We then define a function called salary_increase
that takes a salary as input and returns the salary multiplied by 1.1 (to represent a 10% salary increase).
Next, we use the apply()
method to apply the salary_increase
function to the salary
column in the DataFrame. We do this by selecting the salary
column using the syntax df['salary']
and then calling the apply()
method on it with the salary_increase
function as an argument.
Finally, we print the updated DataFrame, which shows the original name
and age
columns, but with the salary
column updated to reflect a 10% increase.
Conclusion
In this article, we explored how to apply a function to a specific column in a pandas DataFrame. We learned that the apply()
method in pandas is a powerful tool for applying custom functions to DataFrame columns, and we saw an example of how to use it to apply a 10% salary increase to a DataFrame of employee data.
By knowing how to apply functions to specific columns in a pandas DataFrame, you can more easily manipulate and analyze your data to gain valuable insights and make informed decisions. So the next time you need to apply a function to a specific column in a DataFrame, remember to use the apply()
method in pandas!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.