How to Append Two Data Frames with Pandas
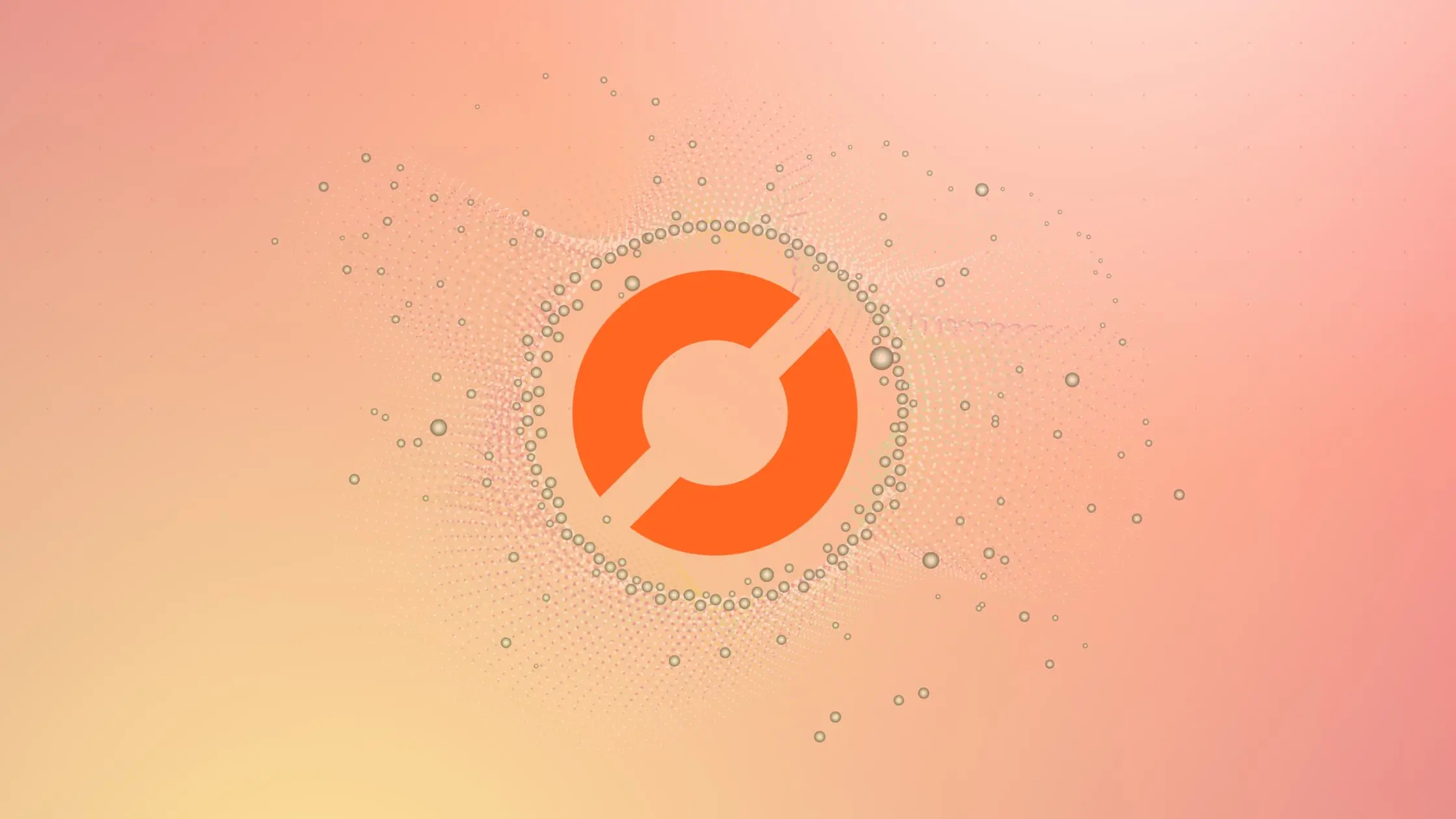
As a data scientist or software engineer, working with data is an essential part of our job. We often need to combine data from different sources to extract insights and make informed decisions. Pandas is a popular Python library that provides powerful tools for data manipulation and analysis. In this article, we will discuss how to append two data frames with Pandas.
What is a data frame?
A data frame is a two-dimensional table that stores data in rows and columns. In Pandas, a data frame is a primary data structure for data manipulation and analysis. It is a powerful tool that allows you to perform complex data operations such as filtering, sorting, and aggregating.
What is appending?
Appending is the process of adding rows from one data frame to another. It is a useful operation when you have two data frames with similar structures and want to combine them into a single data frame.
How to append two data frames with Pandas
To append two data frames with Pandas, you can use the concat()
or append()
function.
The concat()
function
The concat()
function takes a list of data frames as an argument and concatenates them along a specified axis. By default, it concatenates them along the rows (axis=0).
Here’s an example of how to use the concat()
function to append two data frames with Pandas:
import pandas as pd
# create the first data frame
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# create the second data frame
df2 = pd.DataFrame({'A': [7, 8, 9], 'B': [10, 11, 12]})
# append the second data frame to the first data frame
df = pd.concat([df1, df2])
# print the result
print(df)
Output:
A B
0 1 4
1 2 5
2 3 6
0 7 10
1 8 11
2 9 12
In this example, we created two data frames (df1
and df2
) with identical structures. We then used the concat()
function to append df2
to df1
along the rows. The result is a new data frame df
that contains all the rows from df1
and df2
.
Note that the indices of the two data frames are retained in the concatenated data frame. To reset the index, you can use the reset_index()
function:
df = pd.concat([df1, df2]).reset_index(drop=True)
Output:
A B
0 1 4
1 2 5
2 3 6
3 7 10
4 8 11
5 9 12
The reset_index()
function resets the index of the concatenated data frame and drops the old index.
The append
function:
The append
method is a convenient way to append one DataFrame to another, similar to how we might append a row to a list.
import pandas as pd
# Creating two sample DataFrames
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = pd.DataFrame({'A': [7, 8, 9], 'B': [10, 11, 12]})
# Appending DataFrames using append method
result = df1.append(df2, ignore_index=True)
print(result)
The ignore_index=True
argument is used to reindex the resulting DataFrame.
Output:
A B
0 1 4
1 2 5
2 3 6
3 7 10
4 8 11
5 9 12
Conclusion
In conclusion, appending two data frames with Pandas is a useful operation when you want to combine data from different sources. The concat()
and append()
function are powerfuls tool that allow you to append data frames along the rows or columns. By following the steps and examples provided in this article, you can easily append two data frames with Pandas in your data science or software engineering projects.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.