How to Append Rows to a Pandas DataFrame
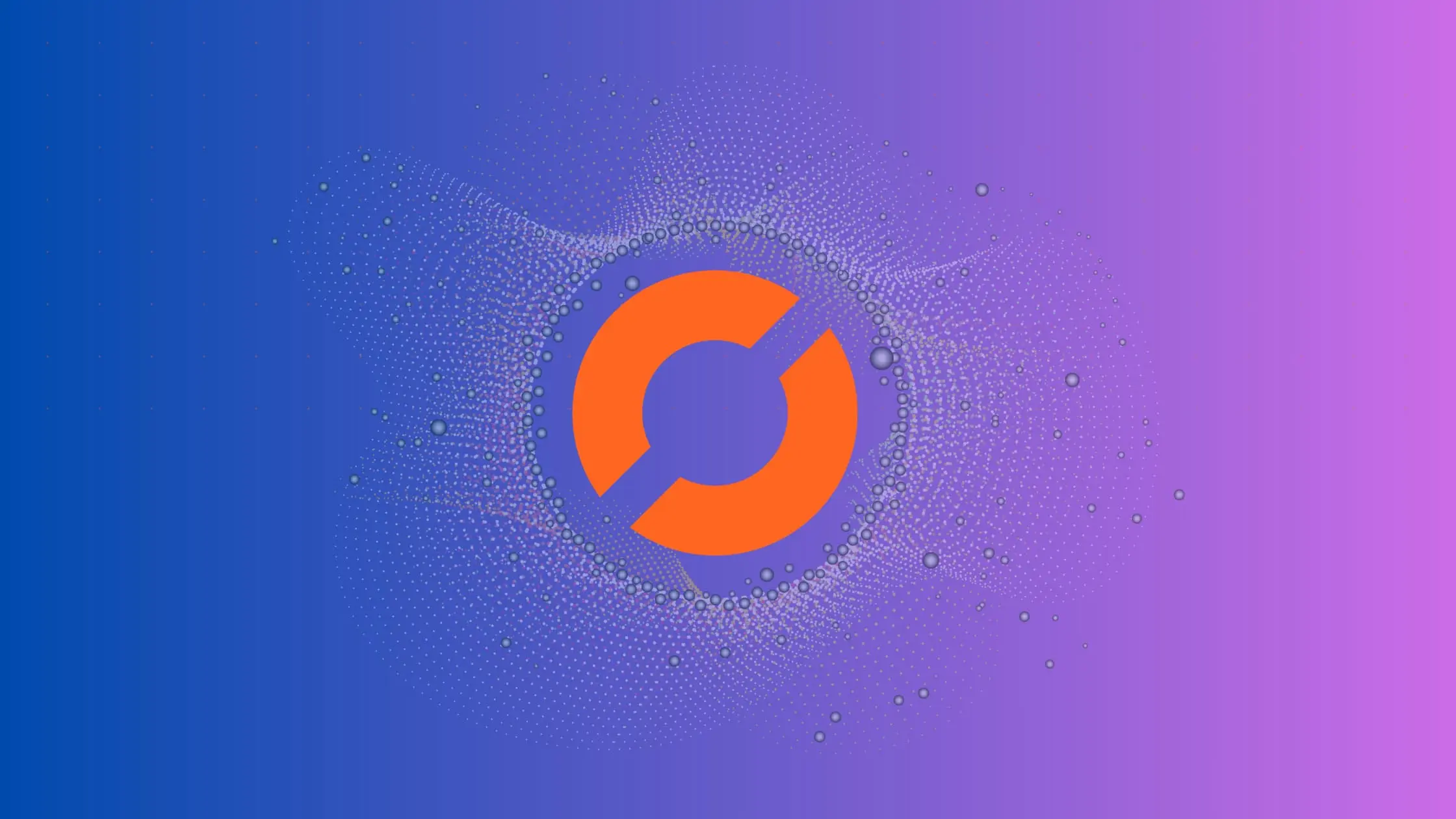
As a data scientist or software engineer, you’re likely familiar with Pandas, a popular data manipulation library in Python. One of the most fundamental tasks when working with data is appending rows to a DataFrame. In this article, we’ll cover how to do just that.
What is a Pandas DataFrame?
A Pandas DataFrame is a two-dimensional, size-mutable, tabular data structure with labeled axes (rows and columns). It is akin to a spreadsheet or SQL table, but with more powerful features. A DataFrame can hold data of different types, including integers, floats, strings, and more.
Why Append Rows to a Pandas DataFrame?
In data science, it’s common to receive new data over time. To keep our analyses up-to-date, we need to append new rows to our existing data. Appending rows is also useful when we want to combine multiple data sources.
How to Append Rows to a Pandas DataFrame
To append rows to a Pandas DataFrame, we can use the append()
method. This method returns a new DataFrame with the appended rows.
Here’s an example:
import pandas as pd
# create a DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# create a new row
new_row = {'A': 7, 'B': 8}
# append the new row to the DataFrame
df = df.append(new_row, ignore_index=True)
print(df)
Output:
A B
0 1 4
1 2 5
2 3 6
3 7 8
In this example, we first create a DataFrame with two columns A
and B
. We then create a new row new_row
with the same column names as the DataFrame. We use the append()
method to append the new row to the DataFrame and set the ignore_index
parameter to True
to reset the index of the resulting DataFrame.
Appending Multiple Rows to a Pandas DataFrame
We can also append multiple rows to a Pandas DataFrame using the append()
method. To do this, we first create a list of dictionaries, where each dictionary represents a row to append. We then pass this list to the append()
method.
Here’s an example:
import pandas as pd
# create a DataFrame
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# create a list of new rows
new_rows = [{'A': 7, 'B': 8}, {'A': 9, 'B': 10}]
# append the new rows to the DataFrame
df = df.append(new_rows, ignore_index=True)
print(df)
Output:
A B
0 1 4
1 2 5
2 3 6
3 7 8
4 9 10
In this example, we create a list of two dictionaries new_rows
, each representing a row to append. We pass this list to the append()
method to append the new rows to the DataFrame.
Conclusion
Appending rows to a Pandas DataFrame is a common and important task in data science. In this article, we covered how to append a single row and multiple rows to a DataFrame using the append()
method. Remember to set the ignore_index
parameter to True
to reset the index of the resulting DataFrame.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.