How to Add Calculated Columns to a Dataframe in Pandas
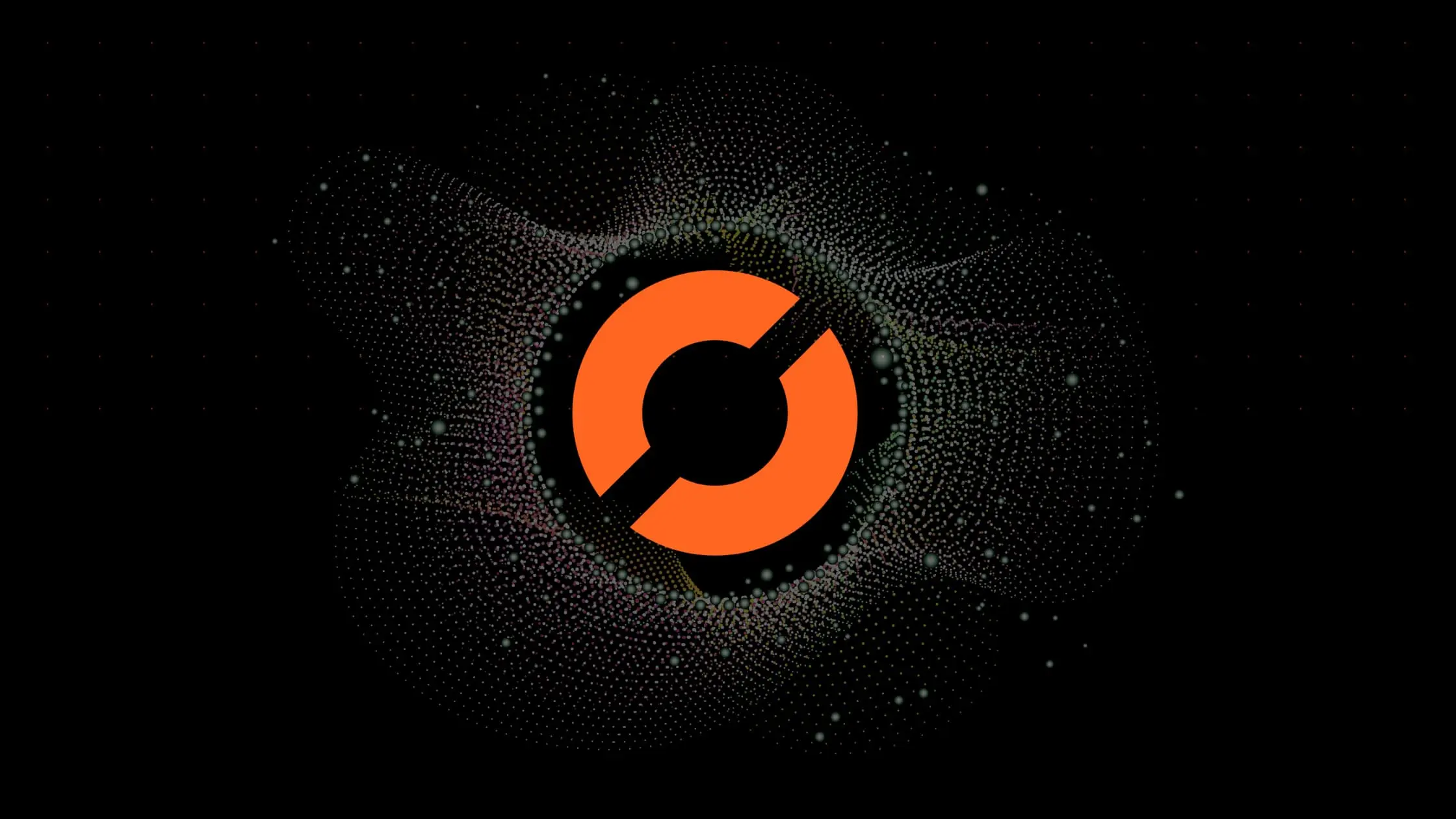
As a data scientist or software engineer, you might have come across situations where you need to add calculated columns to a dataframe in pandas. Pandas is a popular data manipulation library in Python that provides a powerful and flexible way to work with structured data. In this article, we will explore how to add calculated columns to a dataframe in pandas.
What is a Dataframe in Pandas?
A dataframe is a two-dimensional labeled data structure in pandas, where the columns can be of different data types (e.g., numerical, categorical, or text). It is similar to a spreadsheet or SQL table, where each row represents an observation, and each column represents a variable. Dataframes are useful for data cleaning, manipulation, and analysis.
Adding a Calculated Column to a Dataframe
Adding a calculated column to a dataframe involves performing some computation based on the values of existing columns and storing the result in a new column. The new column can be added to the existing dataframe or create a new one. There are several ways to add calculated columns to a dataframe in pandas.
Method 1: Using Basic Arithmetic Operators
The easiest way to add a calculated column to a dataframe is to use basic arithmetic operators (+, -, *, /) on the existing columns. For example, suppose we have a dataframe with two columns, A
and B
, and we want to add a new column C
that is the sum of A
and B
.
import pandas as pd
# Creating a dataframe with columns A and B
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# Adding a new column C using basic arithmetic operators
df['C'] = df['A'] + df['B']
# Printing the updated dataframe
print(df)
Output:
A B C
0 1 4 5
1 2 5 7
2 3 6 9
In this example, we create a new dataframe df
with two columns A
and B
. We then add a new column C
to the dataframe, which is the sum of columns A
and B
.
Method 2: Using the apply Method
Another way to add a calculated column to a dataframe is to use the apply
method. The apply
method applies a function to each row or column of a dataframe and returns the result as a new dataframe or series. For example, suppose we have a dataframe with two columns, A
and B
, and we want to add a new column C
that is the product of A
and B
.
import pandas as pd
# Creating a dataframe with columns A and B
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# Adding a new column C using the apply method
df['C'] = df.apply(lambda row: row['A'] * row['B'], axis=1)
# Printing the updated dataframe
print(df)
Output:
A B C
0 1 4 4
1 2 5 10
2 3 6 18
In this example, we create a new dataframe df
with two columns A
and B
. We then use the apply
method to calculate the product of columns A
and B
for each row and store the result in a new column C
.
Method 3: Using the eval Method
The eval
method in pandas allows us to evaluate a string containing a Python expression and return the result as a new dataframe or series. This method is useful for adding calculated columns to a dataframe based on the values of existing columns. For example, suppose we have a dataframe with two columns, A
and B
, and we want to add a new column C
that is the sum of A
and B
squared.
import pandas as pd
# Creating a dataframe with columns A and B
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# Adding a new column C using the eval method
df.eval('C = A**2 + B**2', inplace=True)
# Printing the updated dataframe
print(df)
Output:
A B C
0 1 4 17
1 2 5 29
2 3 6 45
In this example, we create a new dataframe df
with two columns A
and B
. We then use the eval
method to calculate the sum of A
and B
squared for each row and store the result in a new column C
.
Conclusion
In this article, we have explored three methods to add calculated columns to a dataframe in pandas. The first method involves using basic arithmetic operators on the existing columns, the second method involves using the apply
method to apply a function to each row or column of a dataframe, and the third method involves using the eval
method to evaluate a string containing a Python expression. These methods provide a flexible and efficient way to manipulate and analyze data in pandas.
When adding calculated columns to a dataframe, it is important to consider the data types of the existing columns and the type of computation to be performed. It is also important to ensure that the new column is added to the correct location in the dataframe and has a meaningful name. With these considerations in mind, you can effectively add calculated columns to a pandas dataframe and perform complex data manipulation tasks.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.