How to Access the Last Element in a Pandas Series
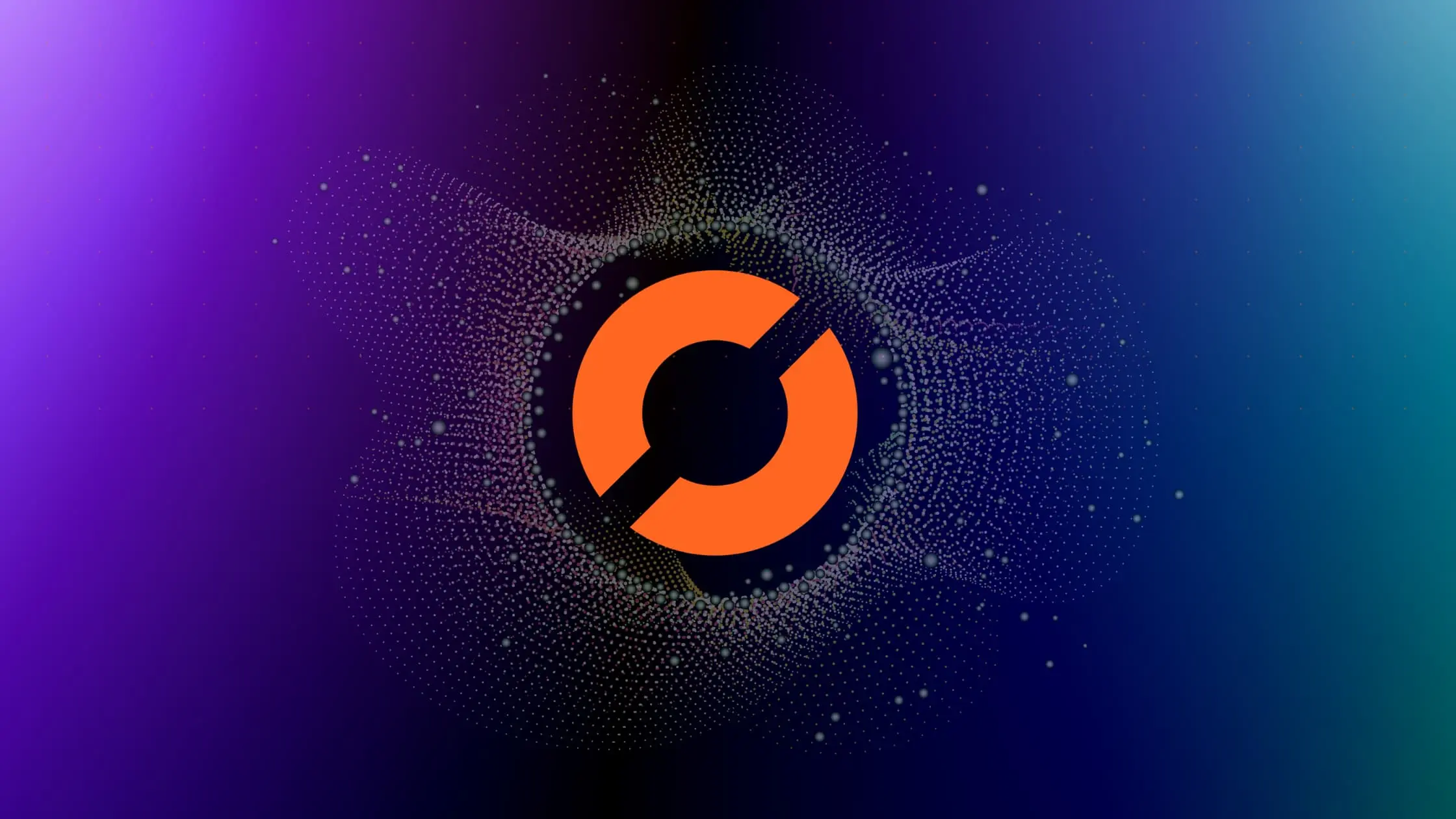
As a data scientist or software engineer, working with data is a daily task. One common task is accessing the last element in a Pandas series. Pandas is a powerful library in Python used for data manipulation and analysis. In this article, we will explore different ways to access the last element in a Pandas series.
Table of Contents
- What is a Pandas Series?
- Accessing the Last Element in a Pandas Series
- Pros and Cons Comparison
- Common Errors and How to Handle Them
- Common Errors and How to Handle Them
What is a Pandas Series?
A Pandas series is a one-dimensional labeled array that can hold any data type. It is similar to a column in a spreadsheet or a SQL table. A series can be created from a list, dictionary, or array.
import pandas as pd
# Creating a series from a list
my_list = [10, 20, 30, 40, 50]
my_series = pd.Series(my_list)
print(my_series)
Output:
0 10
1 20
2 30
3 40
4 50
dtype: int64
Accessing the Last Element in a Pandas Series
There are several ways to access the last element in a Pandas series. We will explore three different methods:
- Using the
.iloc
method - Using the
.tail()
method - Using negative indexing
1. Using the .iloc Method
The .iloc
method is used to access a specific location in the series using integer-based indexing. To access the last element, we can use the index position -1
.
import pandas as pd
# Creating a series from a list
my_list = [10, 20, 30, 40, 50]
my_series = pd.Series(my_list)
# Accessing the last element using the .iloc method
last_element = my_series.iloc[-1]
print(last_element)
Output:
50
2. Using the .tail() Method
The .tail()
method is used to display the last n
elements of a series. By default, n=5
. To access only the last element, we can set n=1
.
import pandas as pd
# Creating a series from a list
my_list = [10, 20, 30, 40, 50]
my_series = pd.Series(my_list)
# Accessing the last element using the .tail() method
last_element = my_series.tail(1).iloc[0]
print(last_element)
Output:
50
3. Using Negative Indexing
Negative indexing starts from the end of the series. To access the last element, we can use the index position -1
.
import pandas as pd
# Creating a series from a list
my_list = [10, 20, 30, 40, 50]
my_series = pd.Series(my_list)
# Accessing the last element using negative indexing
last_element = my_series[-1]
print(last_element)
Output:
50
Pros and Cons Comparison
Method | Pros | Cons |
---|---|---|
.iloc[] | - Explicit integer-based indexing | - Requires knowledge of index positions |
.tail() | - Cleaner syntax | - Involves additional function call |
Negative indexing | - Simple and intuitive | - Limited to basic indexing operations |
Common Errors and How to Handle Them
Error 1: Index Error
import pandas as pd
data = pd.Series([10, 20, 30, 40, 50])
try:
# Trying to access an out-of-range index
last_element = data.iloc[10]
except IndexError as e:
print("Index Error:", e)
Handle this error by ensuring the index is within the valid range.
Error 2: Empty Series
import pandas as pd
data = pd.Series([])
try:
# Trying to access the last element in an empty Series
last_element = data.iloc[-1]
except IndexError as e:
print("Index Error:", e)
Handle this error by checking if the Series is empty before attempting to access the last element.
Conclusion
Accessing the last element in a Pandas series is a common task in data manipulation and analysis. In this article, we explored three different methods to access the last element in a Pandas series: using the .iloc
method, using the .tail()
method, and using negative indexing. These methods are simple, efficient, and can be easily implemented in your code.
As a data scientist or software engineer, it is important to have a good understanding of Pandas and its functionalities. Pandas is a powerful library that can simplify your data manipulation and analysis tasks.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.