How to Access Pandas Columns with Spaces in Column Names
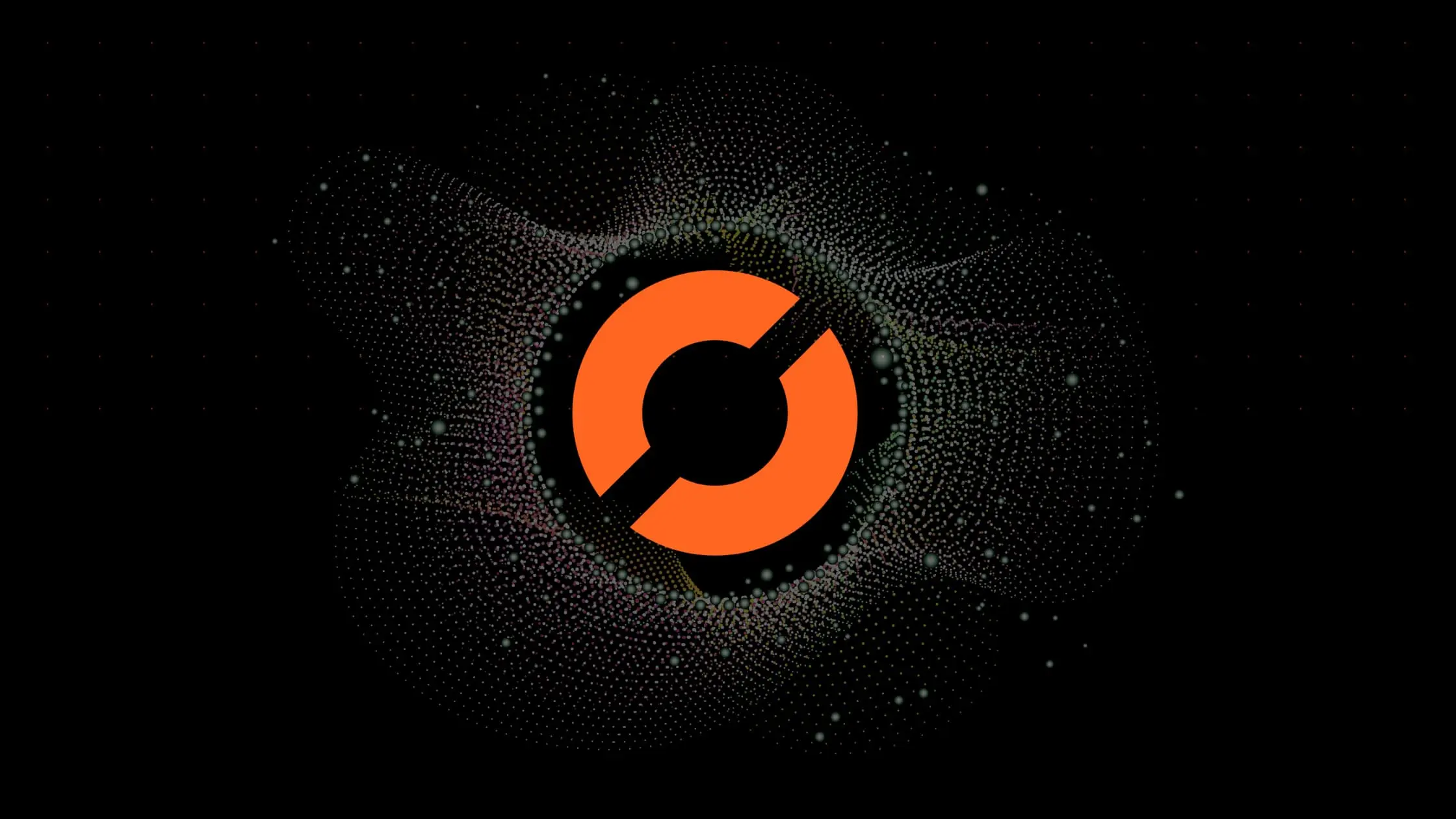
As a data scientist or software engineer, you’ve probably encountered a situation where you need to access columns in a Pandas dataframe that have spaces in their column names. This can be a frustrating experience, as the typical methods for accessing columns with regular column names won’t work. In this article, we’ll go over the different ways you can access columns with spaces in their names using Pandas.
Table of Contents
- Why are Column Names with Spaces a Problem?
- Methods To Access Column With a Space in Its Name
- Common Error and Solution
- Conclusion
Why are Column Names with Spaces a Problem?
In Pandas, column names are typically accessed using dot notation. For example, if you have a dataframe with a column named "age"
, you can access it using df.age
. However, if you have a column with a space in its name, like "first name"
, you can’t use dot notation to access it. Instead, you need to use a different method to access it.
Let’s consider the following DataFrame:
first name last name
0 Jane Smith
1 Dwayne Johnson
2 Jon Doe
Methods To Access Column With a Space in Its Name
Method 1: Using Bracket Notation
The simplest way to access a column with a space in its name is to use bracket notation. To do this, you simply enclose the column name in square brackets. For example, if you have a dataframe named df
with a column named "first name"
, you can access it using df['first name']
. This method works for all columns, regardless of their names.
print(df["first name"])
Output:
0 Jane
1 Dwayne
2 Jon
Name: first name, dtype: object
Method 2: Using the getattr()
Function
Another way to access a column with a space in its name is to use the getattr()
function. This function takes two arguments: the object you want to access the attribute from, and the name of the attribute you want to access. For example, if you have a dataframe named df
with a column named “first name”, you can access it using getattr(df, 'first name')
.
print(getattr(df, 'first name'))
Output:
0 Jane
1 Dwayne
2 Jon
Name: first name, dtype: object
Method 3: Renaming Columns
If you find yourself accessing a column with a space in its name frequently, you might want to consider renaming the column to something without spaces. This can make your code easier to read and less error-prone. To rename a column in Pandas, you can use the rename()
method. For example, if you have a dataframe named df
with a column named “first name”, you can rename it to “first_name” using the following code:
df = df.rename(columns={'first name': 'first_name'})
print(df.first_name)
After running this code, you can access the column using dot notation like this: df.first_name
.
Output:
0 Jane
1 Dwayne
2 Jon
Name: first name, dtype: object
Method 4: Using the query() Method
The query()
method in Pandas allows you to filter a dataframe based on a specified condition. It can also be used to select specific columns, including those with spaces in their names. To select a column with a space in its name using the query()
method, you can enclose the column name in backticks. For example, if you have a dataframe named df
with a column named “first name”, you can select it using the following code:
df_filtered = df.query('`first name` == "Jon"')
print(df_filtered)
This code will create a new dataframe containing only the rows where the "first name"
column is equal to "Jon"
.
Output:
first_name last name
2 Jon Doe
Common Error and Solution
1. SyntaxError when Using Dot Notation
Error Description: Attempting to access a column with a space in its name using dot notation results in a SyntaxError.
# Creating a dataframe with a column having a space in its name
import pandas as pd
data = {'first name': ['Jon', 'Jane', 'Mike'], 'age': [25, 30, 35]}
df = pd.DataFrame(data)
# Trying to access the column with dot notation
try:
df.first name
except Exception as e:
print(f"Error: {type(e).__name__}: {e}")
Solution:
# Accessing the column with bracket notation
column_data = df['first name']
print(column_data)
Conclusion
Accessing columns with spaces in their names can be a frustrating experience in Pandas, but there are several methods you can use to make it easier. The simplest way is to use bracket notation, but you can also use the getattr()
function, rename your columns, or use the query()
method. By using these methods, you can avoid errors and make your code more readable.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.