How do I convert a Pandas dataframe to a PyTorch tensor
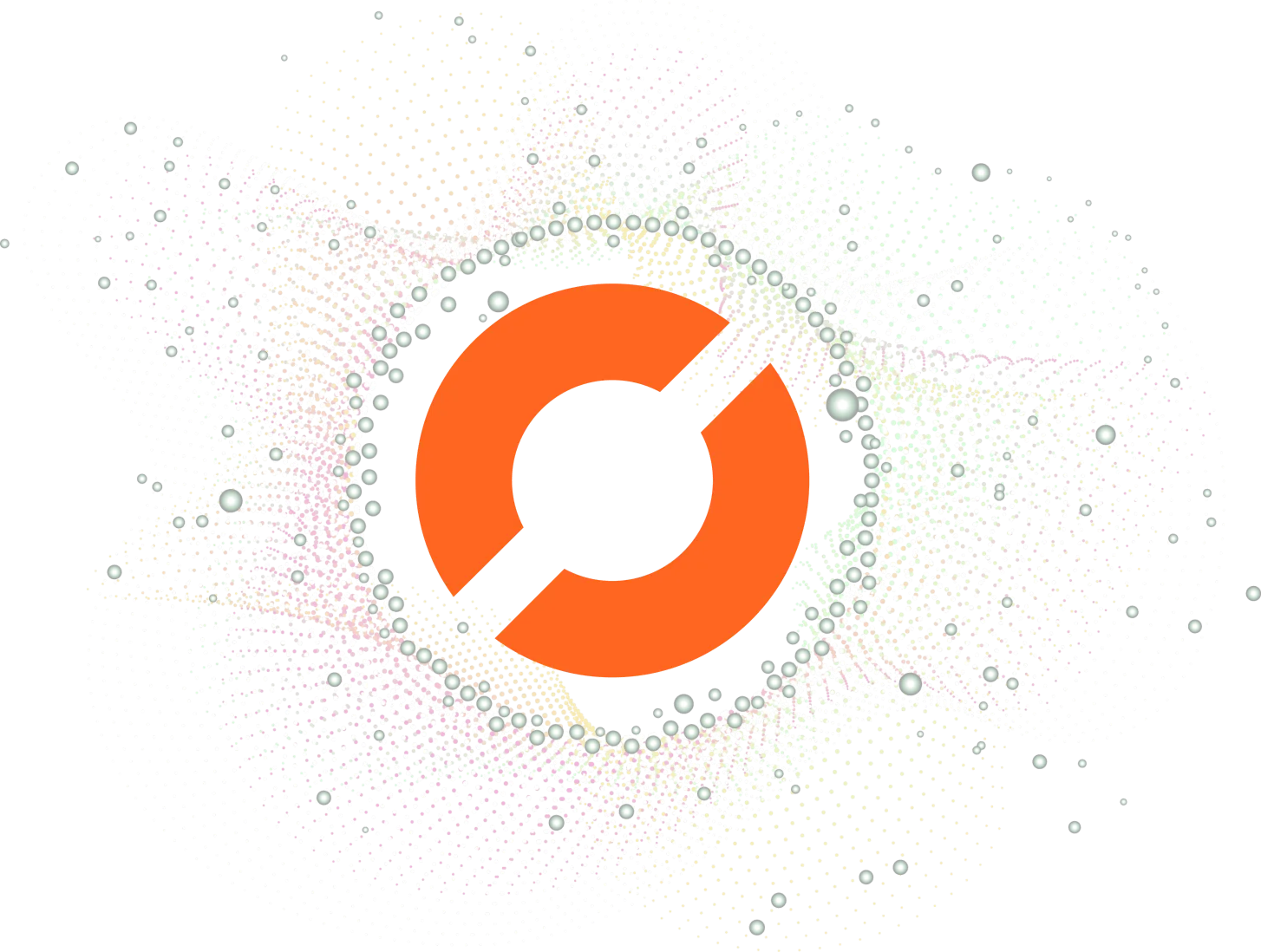
As a data scientist, you may often work with Pandas dataframes to manipulate and analyze data. However, when it comes to building machine learning models, you may need to convert your Pandas dataframe into a PyTorch tensor. In this blog post, we will explore how to do this conversion efficiently.
Understanding Pandas dataframes and PyTorch tensors
Before we dive into the conversion process, let’s first understand what Pandas dataframes and PyTorch tensors are.
A Pandas dataframe is a two-dimensional, size-mutable, tabular data structure with rows and columns. It is similar to an Excel spreadsheet or a SQL table. You can perform various operations on dataframes, such as filtering, grouping, and merging.
On the other hand, a PyTorch tensor is a multi-dimensional array that can hold numerical data. It is the fundamental data structure used in PyTorch for building machine learning models. You can perform various operations on tensors, such as matrix multiplication, addition, and subtraction.
Converting a Pandas dataframe to a PyTorch tensor
To convert a Pandas dataframe to a PyTorch tensor, we need to follow a few steps. Let’s explore each step in detail.
Step 1: Import the necessary libraries
First, we need to import the necessary libraries. We need Pandas to read the data from a CSV file and convert it into a dataframe. We also need PyTorch to convert the dataframe into a tensor.
import pandas as pd
import torch
Step 2: Read the data into a Pandas dataframe
Next, we need to read the data into a Pandas dataframe. We can use the read_csv
function of Pandas to read a CSV file.
df = pd.read_csv('data.csv')
Step 3: Convert the Pandas dataframe to a PyTorch tensor
Now that we have the data in a Pandas dataframe, we can convert it into a PyTorch tensor. We can use the tensor
function of PyTorch to convert the dataframe into a tensor.
tensor = torch.tensor(df.values)
Here, we are using the values
attribute of the dataframe to extract the data as a numpy array, which can then be converted into a tensor using the tensor
function.
Step 4: Convert the data type of the tensor (optional)
If the data in the dataframe is not of the correct data type, we may need to convert it before converting the dataframe to a tensor. For example, if the data is in string format, we may need to convert it to a float or an integer.
df['column_name'] = df['column_name'].astype(float)
Here, we are using the astype
function of Pandas to convert the data type of a specific column in the dataframe.
Step 5: Normalize the data (optional)
If the data in the dataframe has a large range of values, we may need to normalize it before converting the dataframe to a tensor. Normalization helps to scale the data to a smaller range, which can improve the performance of the machine learning model.
df['column_name'] = (df['column_name'] - df['column_name'].mean()) / df['column_name'].std()
Here, we are using the z-score normalization technique to normalize a specific column in the dataframe.
Step 6: Save the tensor (optional)
If we want to save the tensor for later use, we can do so using the save
function of PyTorch.
torch.save(tensor, 'data.pt')
Here, we are saving the tensor as a file named data.pt
.
Conclusion
In this blog post, we explored how to convert a Pandas dataframe to a PyTorch tensor. We learned that we need to import the necessary libraries, read the data into a Pandas dataframe, convert the dataframe into a PyTorch tensor, and optionally convert the data type and normalize the data. We also learned how to save the tensor for later use. By following these steps, we can efficiently convert our data into a format suitable for building machine learning models in PyTorch.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.