How can I validate an email address using a regular expression
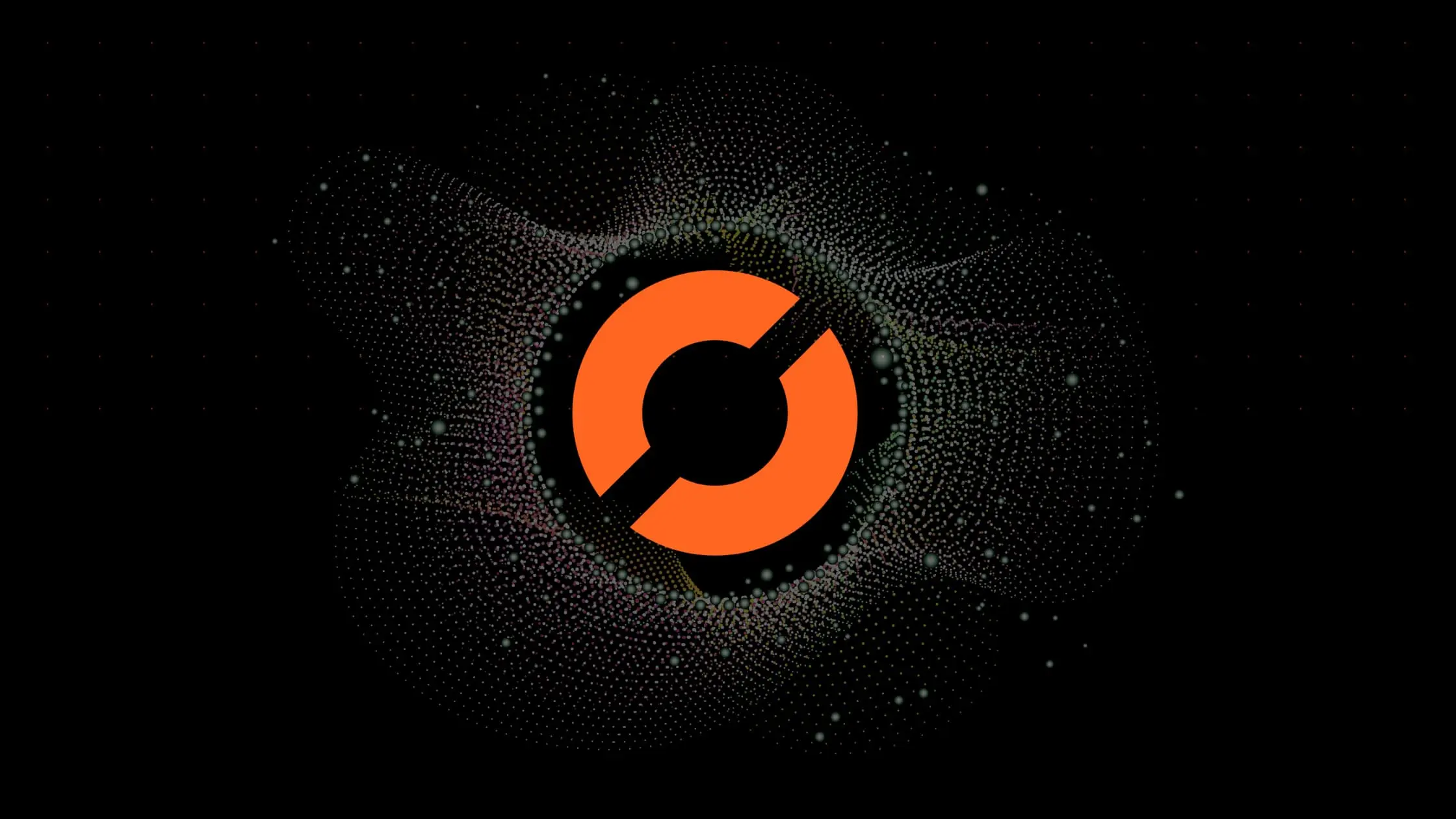
As a software engineer, one of the most common tasks you may encounter is validating user input. In particular, validating email addresses is a crucial step in ensuring that your application is secure and user-friendly. In this post, we’ll explore how to validate email addresses using regular expressions in order to help you develop more robust and secure software.
Table of Contents
- Why validate email addresses?
- What is a regular expression?
- The anatomy of an email address
- Building a regular expression for email validation
- Using the regular expression in your code
- Common Errors and How to Handle Them
- Conclusion
Why validate email addresses?
Before we dive into the specifics of email validation, let’s first discuss why it’s important to validate email addresses in the first place. First and foremost, email validation helps to ensure that the email address entered by the user is formatted correctly. This can help prevent typos and other mistakes that could lead to delivery failures or other issues down the line.
Additionally, email validation can help prevent malicious attacks such as email spoofing or phishing. By validating that the email address entered by the user is legitimate, you can help prevent attackers from sending fake emails that appear to come from legitimate sources.
What is a regular expression?
A regular expression, also known as a regex, is a pattern that describes a set of strings. Regular expressions are commonly used in programming to search for, match, and manipulate text.
In the context of email validation, a regular expression can be used to check whether an email address is properly formatted. By defining a pattern that matches valid email addresses, we can use a regular expression to quickly and efficiently validate user input.
The anatomy of an email address
Before we can create a regular expression to validate email addresses, it’s important to understand the basic structure of an email address. At a high level, an email address consists of two parts: the local part and the domain part.
The local part is the portion of the email address that comes before the “@” symbol. This part can contain a variety of characters, including letters, numbers, and special characters such as "."
, "-"
, and "_"
.
The domain part, on the other hand, is the portion of the email address that comes after the "@"
symbol. This part typically consists of a domain name, which can contain letters, numbers, and hyphens.
Building a regular expression for email validation
Now that we understand the basic structure of an email address, we can begin to build a regular expression to validate email addresses. There are many different regular expressions that can be used for email validation, but we’ll focus on one that is commonly used and fairly robust.
Here’s the regular expression we’ll be using:
/^([a-zA-Z0-9._%-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,})$/
Let’s break down this regular expression piece by piece:
^
- The beginning of the string.(
- Start of a capturing group.[a-zA-Z0-9._%-]+
- Matches one or more of the following characters: letters (both uppercase and lowercase), numbers, periods, underscores, percent signs, and hyphens.@
- Matches the “@” symbol.[a-zA-Z0-9.-]+
- Matches one or more of the following characters: letters (both uppercase and lowercase), numbers, periods, and hyphens.\.
- Matches a literal period character.[a-zA-Z]{2,}
- Matches two or more letters (both uppercase and lowercase).)
- End of the capturing group.$
- The end of the string.
This regular expression matches email addresses that are properly formatted according to the rules we discussed earlier. Specifically, it matches email addresses that have a valid local part and a valid domain part, separated by an "@"
symbol.
Using the regular expression in your code
Now that we have a regular expression for email validation, let’s discuss how to use it in your code. The specific implementation will depend on the programming language you’re using, but the general process will be the same.
Here’s an example of how to use the regular expression in Python:
import re
email_pattern = re.compile(r'^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$')
def validate_email(email):
if email_pattern.match(email):
print(f"{email} is a valid email address.")
else:
print(f"{email} is not a valid email address.")
validate_email("john.doe@saturncloud.io") # Valid email address
validate_email("invalid_email") # Invalid email address
Output:
john.doe@saturncloud.io is a valid email address.
invalid_email is not a valid email address.
In this example, we define a regular expression for email validation and wrap it in a function called validate_email
. This function takes an email address as an argument and returns a boolean value indicating whether the email address is valid according to the regular expression.
We then call the validate_email
function with an example email address and log the result to the console.
Common Errors and How to Handle Them:
False Negatives: If the regex pattern rejects a valid email, ensure that the pattern is up-to-date with the latest email standards.
False Positives: If the regex pattern accepts an invalid email, review and adjust the pattern to enhance accuracy.
Conclusion
Validating email addresses is a critical step in ensuring the security and user-friendliness of your applications. This comprehensive guide has explored the use of regular expressions for efficient email address validation, covering the anatomy of an email address, building a robust regex pattern, and implementing it in your code. Armed with this knowledge, you can develop software that effectively validates user input, enhancing the overall security of your applications.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.