Filter Dataframe with Multiple Conditions Name Matching in R dplyr
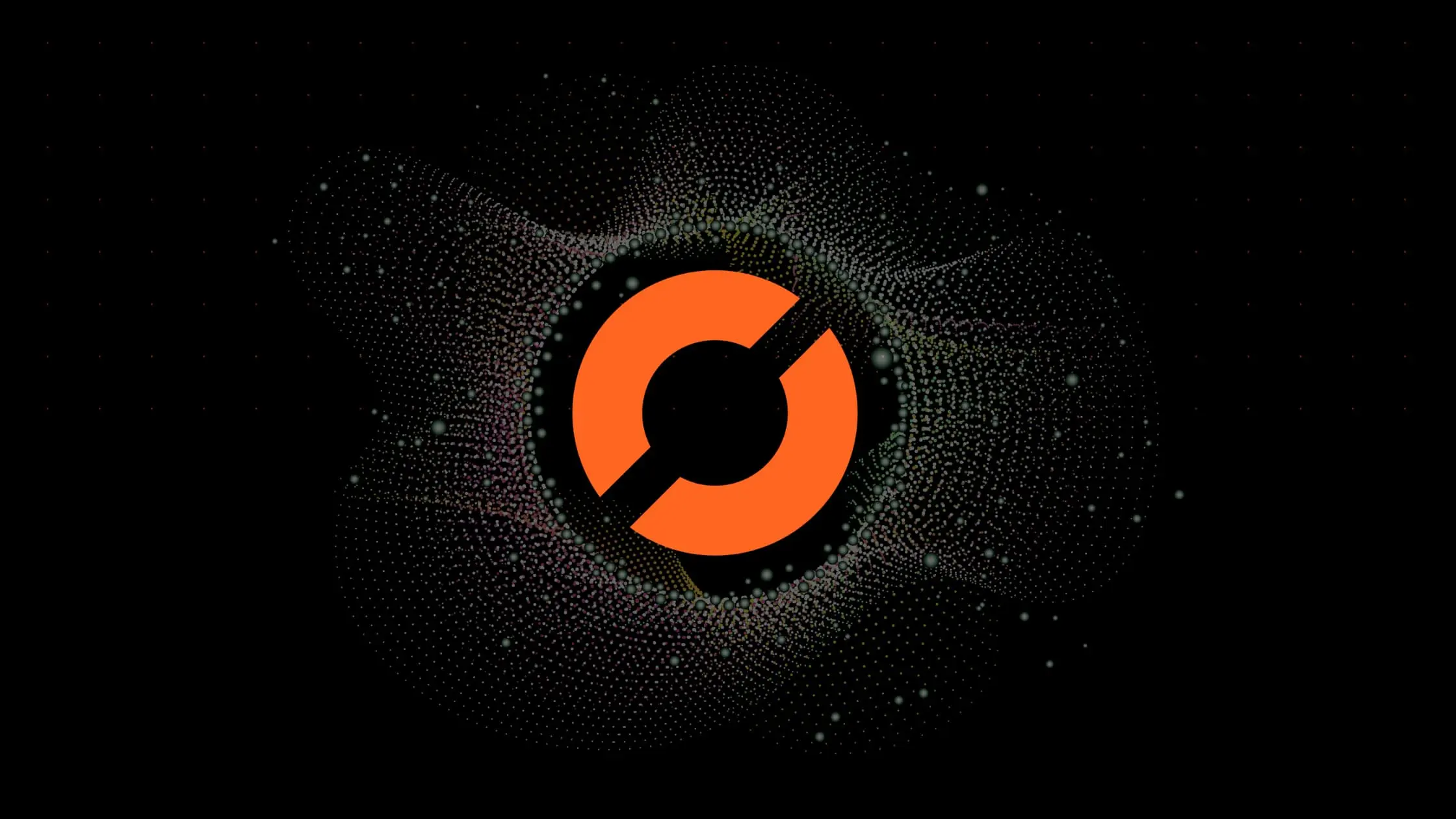
Table of Contents
- Introduction to dplyr
- Filtering Dataframes with dplyr
- Multiple Conditions Name Matching
- Combining Multiple Conditions
- Conclusion
Introduction to dplyr
Dplyr is a part of the tidyverse, a collection of R packages designed for data science. It provides a set of functions that perform common data manipulation operations, making it easier to read and write code. The key functions in dplyr are:
filter()
: Subset rows using column valuesselect()
: Subset columns using column namesmutate()
: Create new columns using existing onessummarise()
: Collapse multiple values down to a single summaryarrange()
: Reorder rows by column values
Filtering Dataframes with dplyr
Filtering is a common operation in data analysis. It involves selecting a subset of rows in a dataframe that meet certain conditions. In dplyr, the filter()
function is used for this purpose.
Let’s start with a simple example. Suppose we have a dataframe df
with columns x
, y
, and z
. We want to filter the dataframe to include only rows where x < 50
and z == TRUE
. Here’s how we can do it:
library(dplyr)
# sample data
df=data.frame(x=c(12,31,4,66,78),
y=c(22.1,44.5,6.1,43.1,99),
z=c(TRUE,TRUE,FALSE,TRUE,TRUE))
# condition
filter(df, x<50 & z==TRUE)
The filter()
function takes a logical condition and returns a dataframe with rows where the condition is TRUE.
Multiple Conditions Name Matching
Now, let’s say we want to filter the dataframe based on multiple conditions that involve matching names. For example, we want to include rows where x
is either 12, 4, or 66. We can use the %in%
operator for this:
filter(x %in% c(12, 4, 66))
The %in%
operator checks if a value is in a set of values. The c()
function combines its arguments into a vector.
Combining Multiple Conditions
We can combine multiple conditions using logical operators. For example, if we want to include rows where x
is ‘12’, ‘4’, or ‘66’ and y
is greater than 25, we can do:
filter(x %in% c(12, 4, 66) & y > 25)
Conclusion
The dplyr package in R provides a powerful and flexible way to manipulate data. The filter()
function, in particular, allows us to subset dataframes based on multiple conditions. By combining logical operators and the %in%
operator, we can filter dataframes based on multiple conditions name matching.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.