Feature Selection in PySpark: A Guide for Data Scientists
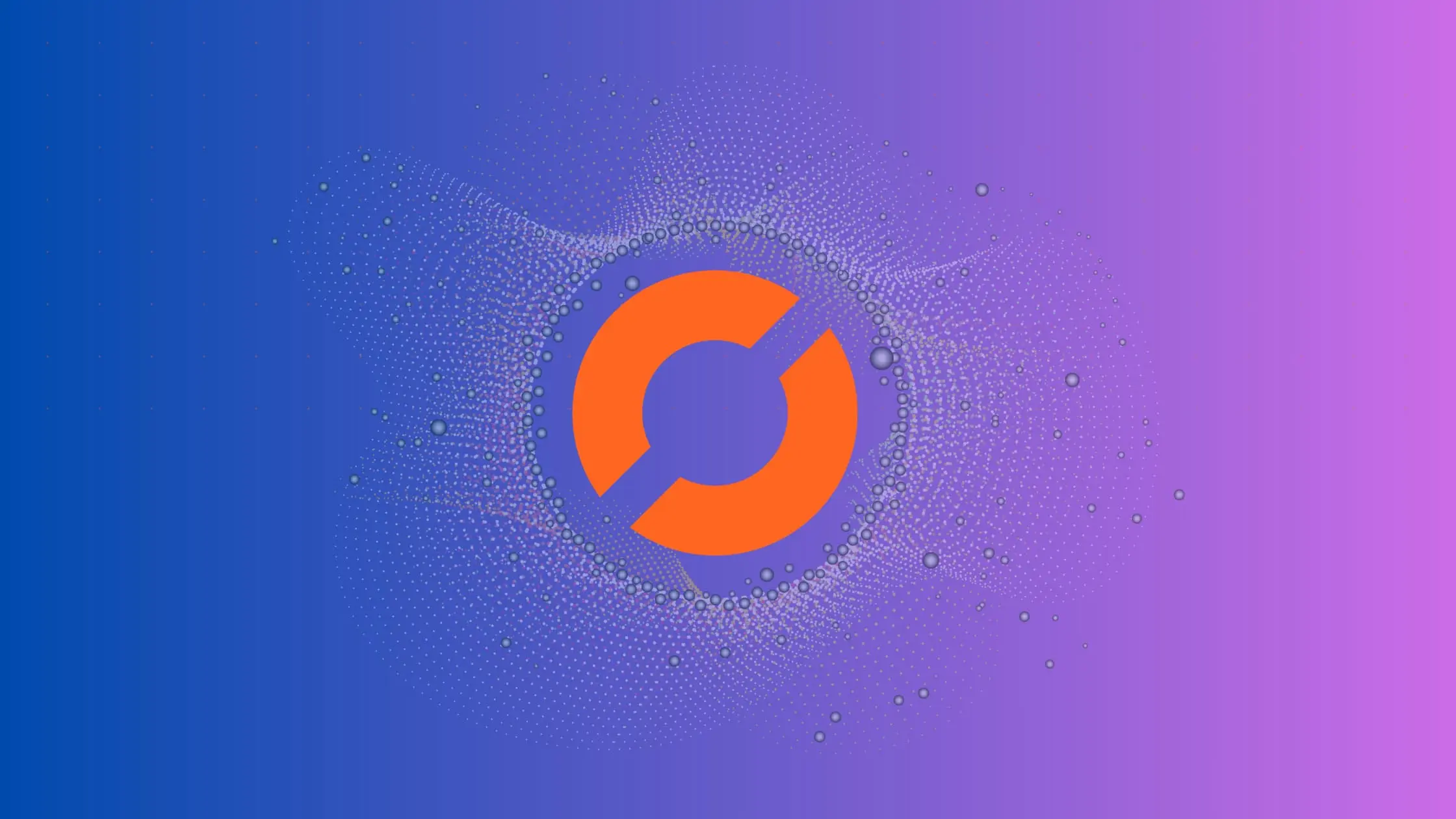
In the world of data science, feature selection is a critical step that can significantly impact the performance of your models. PySpark, the Python library for Apache Spark, offers a variety of tools for this process. This blog post will guide you through the steps of feature selection in PySpark, helping you to optimize your machine learning models.
What is Feature Selection?
Feature selection, also known as variable selection or attribute selection, is the process of selecting a subset of relevant features for use in model construction. The goal is to remove irrelevant or redundant features to improve the model’s performance, reduce overfitting, and enhance interpretability.
Why PySpark?
Apache Spark is a powerful open-source, distributed computing system that’s well-suited for big data processing and analytics. PySpark is the Python API for Spark, which allows Python programmers to leverage the power of Spark. PySpark is particularly useful when dealing with large datasets that can’t fit into memory, as it can process data in a distributed and parallelized manner.
Feature Selection Techniques in PySpark
PySpark provides several methods for feature selection, including:
- Chi-Squared Selector
- Variance Threshold Selector
- Correlation-based Feature Selection
Let’s dive into each of these methods.
Chi-Squared Selector
The Chi-Squared selector is a filter method used for categorical target variables. It measures the dependence between each feature and the target variable. The features with the highest chi-squared statistics are selected.
from pyspark.ml.feature import ChiSqSelector
from pyspark.ml.linalg import Vectors
from pyspark.sql import SparkSession
# Create a Spark session
spark = SparkSession.builder.appName("ChiSqSelectorExample").getOrCreate()
# Sample data
data = [(Vectors.dense([0.0, 0.0, 18.0, 1.0]), 1.0),
(Vectors.dense([0.0, 1.0, 12.0, 0.0]), 0.0),
(Vectors.dense([1.0, 0.0, 15.0, 0.1]), 0.0)]
df = spark.createDataFrame(data, ["features", "label"])
selector = ChiSqSelector(numTopFeatures=1, featuresCol="features",
outputCol="selectedFeatures", labelCol="label")
result = selector.fit(df).transform(df)
print("ChiSqSelector output with top %d features selected" % selector.getNumTopFeatures())
result.show()
Output:
ChiSqSelector output with top 1 features selected
+------------------+-----+----------------+
| features|label|selectedFeatures|
+------------------+-----+----------------+
|[0.0,0.0,18.0,1.0]| 1.0| [18.0]|
|[0.0,1.0,12.0,0.0]| 0.0| [12.0]|
|[1.0,0.0,15.0,0.1]| 0.0| [15.0]|
+------------------+-----+----------------+
Variance Threshold Selector
The Variance Threshold selector is a simple baseline approach to feature selection. It removes all features whose variance doesn’t meet a certain threshold. By default, it removes all zero-variance features, i.e., features that have the same value in all samples.
from pyspark.ml.feature import VarianceThresholdSelector
from pyspark.ml.linalg import Vectors
from pyspark.sql import SparkSession
# Create a Spark session
spark = SparkSession.builder.appName("VarianceThresholdSelector").getOrCreate()
# Sample data
data = [(Vectors.dense([0.0, 0.0, 18.0, 1.0]),),
(Vectors.dense([0.0, 1.0, 12.0, 0.0]),),
(Vectors.dense([1.0, 0.0, 15.0, 0.1]),)]
df = spark.createDataFrame(data, ["features"])
selector = VarianceThresholdSelector(varianceThreshold=0.5, outputCol="selectedFeatures")
result = selector.fit(df).transform(df)
print("Features selected by VarianceThresholdSelector:")
result.show()
Output:
Features selected by VarianceThresholdSelector:
+------------------+----------------+
| features|selectedFeatures|
+------------------+----------------+
|[0.0,0.0,18.0,1.0]| [18.0]|
|[0.0,1.0,12.0,0.0]| [12.0]|
|[1.0,0.0,15.0,0.1]| [15.0]|
+------------------+----------------+
Correlation-based Feature Selection
Correlation-based feature selection is a wrapper method that ranks features based on their correlation with the target variable. Features with high correlation are more likely to be selected.
from pyspark.ml.stat import Correlation
from pyspark.ml.linalg import Vectors
from pyspark.sql import SparkSession
# Create a Spark session
spark = SparkSession.builder.appName("Pearson").getOrCreate()
# Sample data
data = [(Vectors.dense([0.0, 0.0, 18.0, 1.0]),),
(Vectors.dense([0.0, 1.0, 12.0, 0.0]),),
(Vectors.dense([1.0, 0.0, 15.0, 0.1]),)]
df = spark.createDataFrame(data, ["features"])
r1 = Correlation.corr(df, "features").head()
print("Pearson correlation matrix:\n" + str(r1[0]))
Output:
Pearson correlation matrix:
DenseMatrix([[ 1. , -0.5 , 0. , -0.41931393],
[-0.5 , 1. , -0.8660254 , -0.57655666],
[ 0. , -0.8660254 , 1. , 0.9078413 ],
[-0.41931393, -0.57655666, 0.9078413 , 1. ]])
Pros and Cons
Technique | Pros | Cons |
---|---|---|
Chi-Squared Selector | - Handles categorical features well | - Assumes independence of features |
Variance Threshold Selector | - Simple and computationally efficient | - May eliminate useful features with low variance |
Correlation-based Feature Selection | - Removes multicollinearity | - Only captures linear relationships |
Common Errors and Solutions
- Memory Issues: If you encounter memory issues, consider increasing the cluster size or optimizing your feature selection parameters.
- Incorrect Column Names: Ensure that the column names used in the feature selection methods match your DataFrame’s column names.
Conclusion
Feature selection is a crucial step in the data preprocessing pipeline. It can significantly improve the performance of your machine learning models by reducing overfitting, improving accuracy, and reducing training time. PySpark provides several methods for feature selection, making it a powerful tool for data scientists working with large datasets.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.