Efficiently Checking if Arbitrary Object is NaN in Python Numpy and Pandas
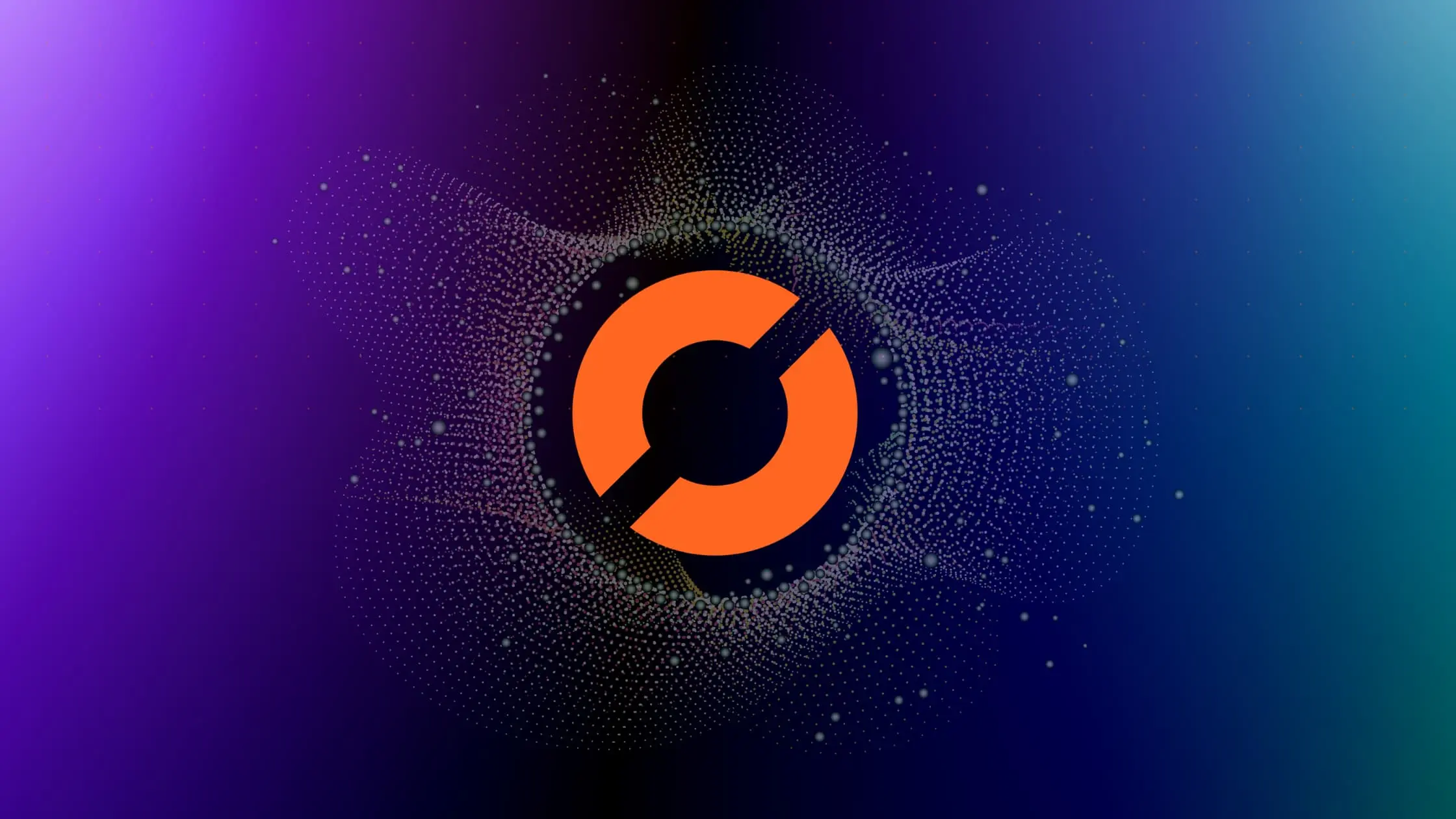
As a data scientist or software engineer, a common task in working with data is checking whether a value is NaN (Not a Number) or not. NaN values can arise in many ways, such as missing data or undefined mathematical operations. In Python, NumPy, and Pandas, there are several ways to efficiently check if an arbitrary object is NaN.
Table of Contents
- Checking for NaN in Python
- Checking for NaN in NumPy
- Checking for NaN in Pandas
- mon Errors and Solutions
- Conclusion
Checking for NaN in Python
In Python, the built-in math
module provides a function called isnan()
that can be used to check if a value is NaN. However, this function only works for floating-point numbers, so it cannot be used to check for NaN in other data types.
import math
value = float('nan')
if math.isnan(value):
print('Value is NaN')
else:
print('Value is not NaN')
Alternatively, you can use the numpy
library’s isnan()
function, which can handle NaN values for different data types, including floating-point, integer, and boolean values.
import numpy as np
value = np.nan
if np.isnan(value):
print('Value is NaN')
else:
print('Value is not NaN')
Checking for NaN in NumPy
In NumPy, you can use the isnan()
function to check for NaN values in an array. This function returns a Boolean array indicating which values in the input array are NaN.
import numpy as np
arr = np.array([1, 2, np.nan, 4])
is_nan = np.isnan(arr)
print(is_nan)
Output:
[False False True False]
You can also use the nan_to_num()
function to replace NaN values with a specified value, such as zero.
import numpy as np
arr = np.array([1, 2, np.nan, 4])
arr = np.nan_to_num(arr, nan=0)
print(arr)
Output:
[1. 2. 0. 4.]
Checking for NaN in Pandas
In Pandas, you can use the isna()
function to check for NaN values in a DataFrame or Series. This function returns a Boolean DataFrame or Series indicating which values in the input DataFrame or Series are NaN.
import pandas as pd
df = pd.DataFrame({'A': [1, 2, np.nan], 'B': [4, np.nan, 6]})
is_nan = df.isna()
print(is_nan)
Output:
A B
0 False False
1 False True
2 True False
You can also use the fillna()
function to replace NaN values with a specified value, such as the mean or median of the non-NaN values in the DataFrame or Series.
import pandas as pd
df = pd.DataFrame({'A': [1, 2, np.nan], 'B': [4, np.nan, 6]})
df = df.fillna(df.mean())
print(df)
Output:
A B
0 1.0 4.0
1 2.0 5.0
2 1.5 6.0
Common Errors and Solutions
Incorrect Application of
np.nan_to_num()
without Understanding Consequences:- Error: Blindly using
np.nan_to_num()
to replace NaN values without considering the impact on data may lead to distorted results, especially if zero is not an appropriate replacement value. - Solution: Before applying
np.nan_to_num()
, carefully consider whether replacing NaN with zero is appropriate for your specific use case. If not, explore other methods, such as using interpolation or domain-specific strategies.
import numpy as np arr = np.array([1, 2, np.nan, 4]) arr = np.nan_to_num(arr, nan=0) # Replacing NaN with zero print(arr)
- Error: Blindly using
Inconsistent Handling of NaN Values Across Multiple Libraries:
- Error: Mixing and matching methods from different libraries (e.g., using
math.isnan()
alongsidenp.isnan()
) may lead to inconsistencies and unexpected results. - Solution: Stick to one library’s conventions for consistency. For example, if you are working with NumPy arrays, use
np.isnan()
consistently throughout your code.
import numpy as np value = np.nan if np.isnan(value): # Consistent use of NumPy's isnan() print('Value is NaN') else: print('Value is not NaN')
- Error: Mixing and matching methods from different libraries (e.g., using
Conclusion
In conclusion, checking for NaN values is a common task in data science and software engineering. In Python, the math
module’s isnan()
function can be used for floating-point numbers, while the numpy
library’s isnan()
function can handle NaN values for different data types. In NumPy, the nan_to_num()
function can be used to replace NaN values with a specified value. In Pandas, the isna()
function can be used to check for NaN values in a DataFrame or Series, and the fillna()
function can be used to replace NaN values with a specified value. By using these functions efficiently, you can ensure that your data analysis and computations are accurate and reliable.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.