Efficiently Appending to a DataFrame within a For Loop in Python
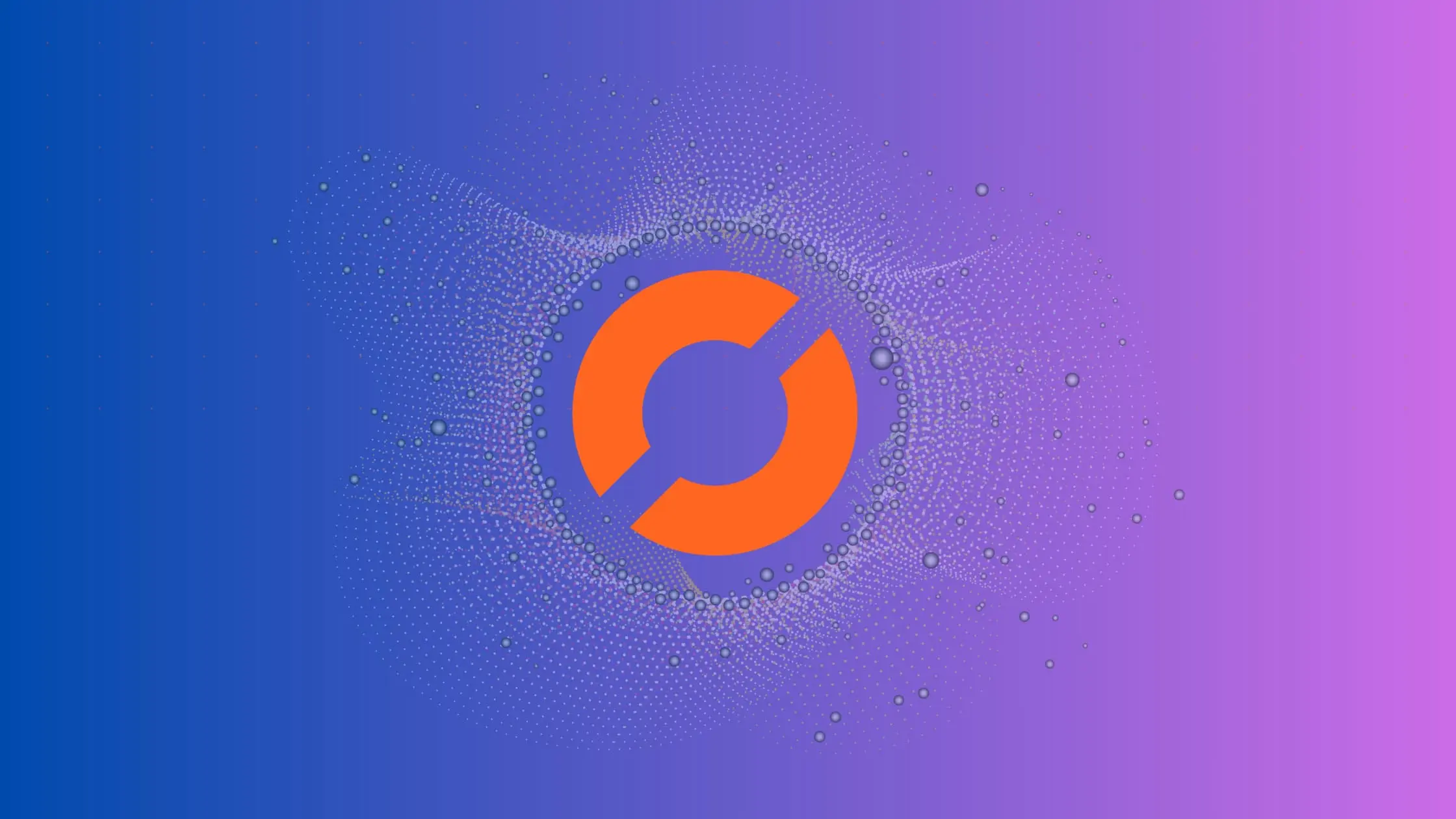
Note:
As of pandas 2.0, `append()` previously deprecated was removed.
You need to use `concat()` instead for most applications:
Understanding the Challenge
When working with large datasets, efficiency is key. A common pitfall is the misuse of the concat()
function within a for loop. This can lead to significant performance issues due to the way pandas handles DataFrame memory allocation. Each time concat()
is called, a new DataFrame is created, which can be very slow and memory-intensive for large datasets.
import pandas as pd
df = pd.DataFrame()
for i in range(10000):
df = pd.concat([df, pd.DataFrame({'A': [i]})], ignore_index=True)
This code will work, but it’s not efficient. Let’s explore a better way.
The Efficient Approach
Instead of appending to the DataFrame directly within the loop, a more efficient approach is to create a list of dictionaries within the loop, and then convert this list to a DataFrame outside the loop.
data = []
for i in range(10000):
data.append({'A': i})
df = pd.DataFrame(data)
This approach is much faster and more memory-efficient because it only creates one DataFrame, rather than creating a new DataFrame with each iteration.
Using List Comprehension
We can make our code even more concise and Pythonic by using list comprehension, a powerful feature in Python that allows us to generate lists in a single line of code.
data = [{'A': i} for i in range(10000)]
df = pd.DataFrame(data)
This code does exactly the same thing as the previous example, but in a more compact and readable way.
Benchmarking Performance
Let’s compare the performance of these methods using the timeit
module. We’ll use a smaller dataset for this test to avoid excessive computation time.
import timeit
# Inefficient method
start_time = timeit.default_timer()
df = pd.DataFrame()
for i in range(10000):
df = pd.concat([df, pd.DataFrame({'A': [i]})], ignore_index=True)
end_time = timeit.default_timer()
print(f"Inefficient method time: {end_time - start_time}")
# Efficient method
start_time = timeit.default_timer()
data = [{'A': i} for i in range(1000)]
df = pd.DataFrame(data)
end_time = timeit.default_timer()
print(f"Efficient method time: {end_time - start_time}")
You’ll find that the efficient method is significantly faster, especially as the size of the dataset increases.
Inefficient method time: 2.3888381000142545
Efficient method time: 0.0006947999354451895
Conclusion
Appending to a DataFrame within a for loop is a common task in data manipulation, but it can be computationally expensive if not done correctly. By creating a list of dictionaries within the loop and converting this list to a DataFrame outside the loop, we can significantly improve the performance of our code. This is a simple but powerful technique that can make a big difference in your data science projects.
Remember, efficient data manipulation is not just about writing code that works—it’s about writing code that works well. By understanding the underlying mechanics of pandas and Python, you can write code that is not only correct, but also fast and efficient.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.