Custom Loss Function in PyTorch: A Guide
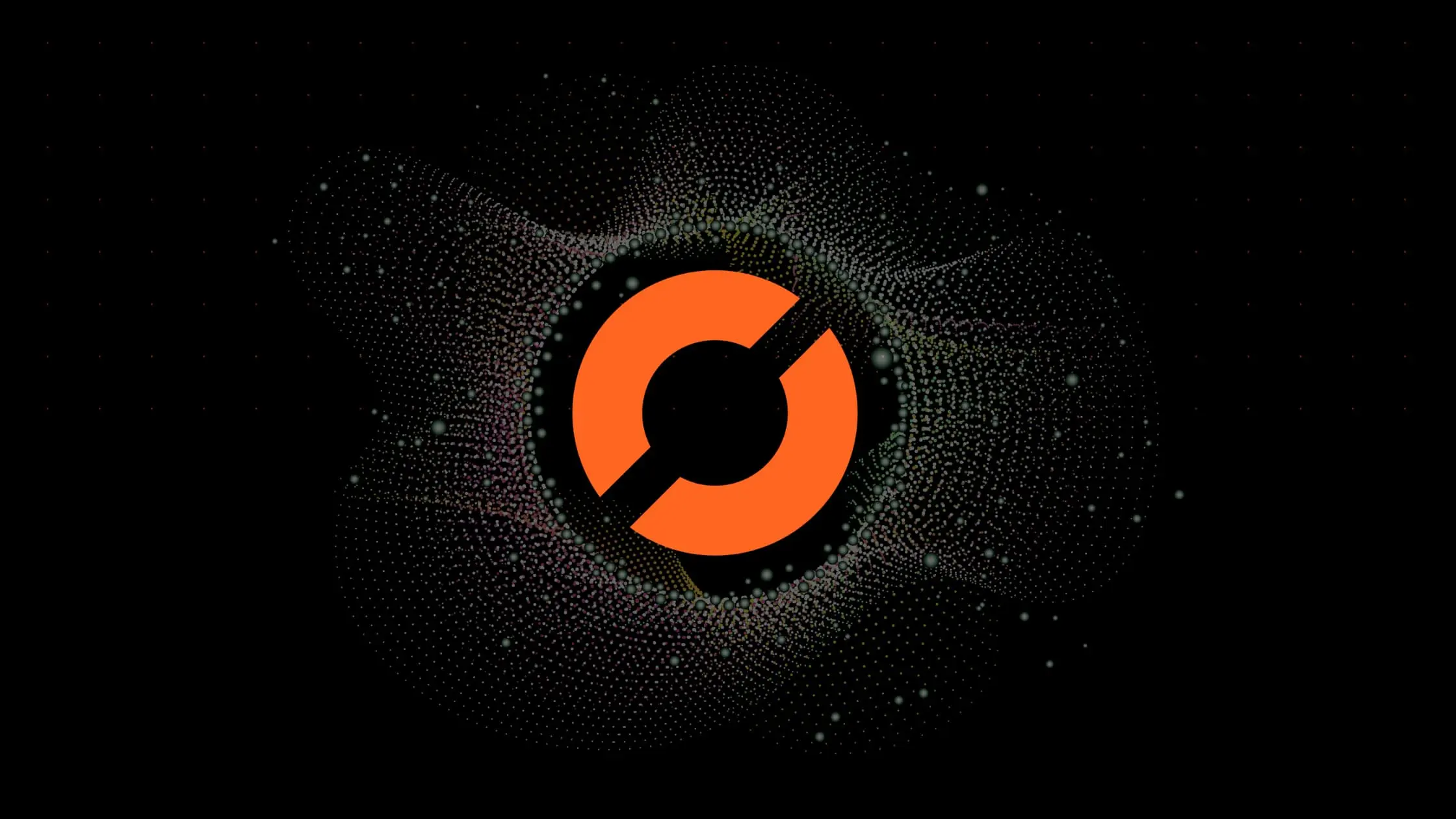
Custom Loss Function in PyTorch: A Guide
As a data scientist or software engineer, you might have come across situations where the standard loss functions available in PyTorch are not enough to capture the nuances of your problem statement. In such cases, you can create custom loss functions in PyTorch to optimize your model’s performance.
In this blog post, we will be discussing how to create custom loss functions in PyTorch and integrate them into your neural network model.
What is a Loss Function?
A loss function, also known as a cost function or objective function, is used to quantify the difference between the predicted and actual output of a machine learning model. The goal of training a machine learning model is to minimize the value of the loss function, which indicates that the model is making accurate predictions.
PyTorch offers a wide range of loss functions for different problem statements, such as Mean Squared Error (MSE) for regression problems and Cross-Entropy Loss for classification problems. However, there are situations where these standard loss functions are not suitable for your problem statement.
Custom Loss Function in PyTorch
A custom loss function in PyTorch is a user-defined function that measures the difference between the predicted output of the neural network and the actual output. You can create custom loss functions in PyTorch by inheriting the nn.Module
class and implementing the forward
method.
Here’s an example of a custom loss function for a binary classification problem:
import torch.nn as nn
class CustomLoss(nn.Module):
def __init__(self):
super(CustomLoss, self).__init__()
def forward(self, inputs, targets):
loss = -1 * (targets * torch.log(inputs) + (1 - targets) * torch.log(1 - inputs))
return loss.mean()
In this example, inputs
are the predicted outputs of the neural network, and targets
are the actual outputs. The loss calculation is performed using the binary cross-entropy loss formula, which penalizes the model for making incorrect predictions.
Once you have defined your custom loss function, you can integrate it into your neural network model by passing it as an argument to the loss
parameter of the optimizer.
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
loss_fn = CustomLoss()
for epoch in range(num_epochs):
# Forward pass
outputs = model(inputs)
loss = loss_fn(outputs, targets)
# Backward and optimize
optimizer.zero_grad()
loss.backward()
optimizer.step()
Advantages of Custom Loss Functions
Custom loss functions can offer several advantages over standard loss functions in PyTorch.
Flexibility
With custom loss functions, you have complete control over the loss calculation process. You can define the loss function in a way that suits your problem statement the best.
Improved Performance
In some cases, using a custom loss function can lead to improved model performance. This is because the custom loss function can capture the nuances of the problem statement better than the standard loss functions.
Compatibility with Complex Models
Custom loss functions can be used with complex neural network models, such as GANs and LSTMs, where standard loss functions may not be sufficient.
Conclusion
In this blog post, we discussed how to create custom loss functions in PyTorch. Custom loss functions can offer several advantages over standard loss functions, such as flexibility, improved performance, and compatibility with complex models.
When creating a custom loss function, it is essential to ensure that the function is differentiable, as PyTorch uses automatic differentiation to optimize the model. Additionally, the custom loss function should be designed to suit your problem statement the best.
By implementing custom loss functions, you can enhance your machine learning models and achieve better results.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.