Creating Custom Loss Functions in Keras/TensorFlow
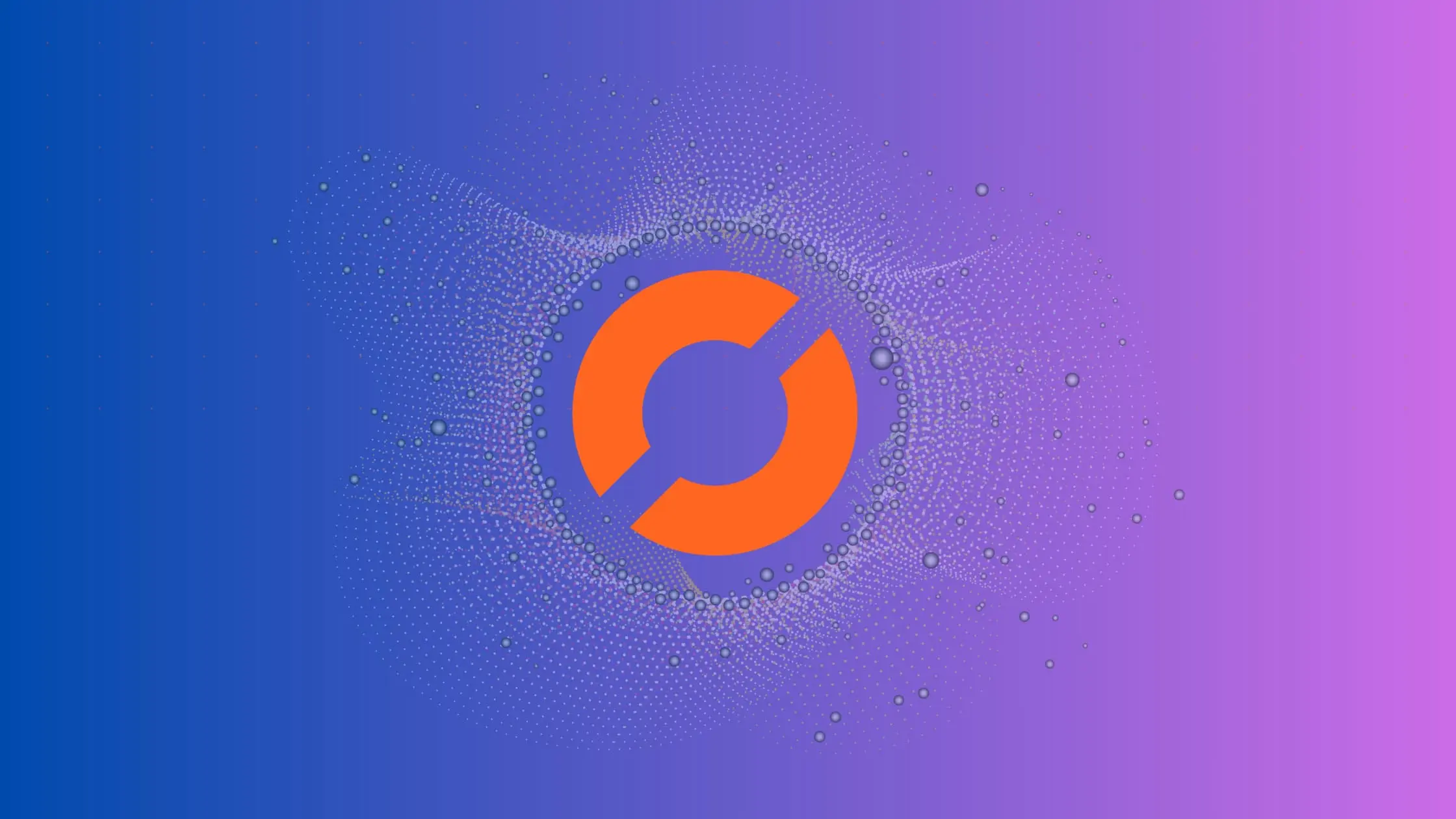
In the world of machine learning, loss functions play a pivotal role. They measure the inconsistency between predicted and actual outcomes, guiding the model towards accuracy. While Keras and TensorFlow offer a variety of pre-defined loss functions, sometimes, you may need to design your own to cater to specific project needs. This blog post will guide you through the process of creating custom loss functions in Keras/TensorFlow.
Table of Contents
- Understanding Loss Functions
- Why Custom Loss Functions?
- Creating a Custom Loss Function
- Implementing Custom Loss Function in Your Model
- A More Complex Example
- Common Errors and Solutions
- Conclusion
Understanding Loss Functions
Before we dive into creating custom loss functions, let’s briefly understand what they are. A loss function, also known as a cost function, quantifies how well your model’s predictions align with the actual data. The objective of any machine learning model is to minimize this loss value.
Keras and TensorFlow provide several built-in loss functions like MeanSquaredError
, BinaryCrossentropy
, CategoricalCrossentropy
, etc. However, these may not always be suitable for your unique problem sets.
Why Custom Loss Functions?
Custom loss functions allow you to incorporate domain knowledge and specific characteristics of your data into your model. They can help improve your model’s performance when standard loss functions fall short.
Creating a Custom Loss Function
Creating a custom loss function in Keras/TensorFlow involves defining a new function using TensorFlow operations. This function should take two arguments: the true values (y_true
) and the model’s predictions (y_pred
).
def custom_loss(y_true, y_pred):
return tf.reduce_mean(tf.square(y_true - y_pred))
In this example, we’ve created a simple custom loss function equivalent to Mean Squared Error. The tf.reduce_mean
function computes the mean of elements across dimensions of a tensor, and tf.square
computes the square of its input.
Implementing Custom Loss Function in Your Model
Once you’ve defined your custom loss function, you can use it in your model by passing it as an argument to the compile
method.
model.compile(optimizer='adam', loss=custom_loss)
A More Complex Example
Let’s create a more complex custom loss function. Suppose we want to penalize false negatives more than false positives in a binary classification problem. We can create a custom loss function to reflect this.
def custom_loss(y_true, y_pred):
# Define weights
false_positive_weight = 1.0
false_negative_weight = 2.0
# Calculate binary cross entropy
bce = tf.keras.losses.BinaryCrossentropy()
# Calculate loss
loss = bce(y_true, y_pred)
# Calculate weighted loss
weighted_loss = tf.where(tf.greater(y_true, y_pred), false_negative_weight * loss, false_positive_weight * loss)
return tf.reduce_mean(weighted_loss)
In this example, we’ve used the tf.where
function to apply different weights to the loss depending on whether the prediction is a false positive or a false negative.
Common Errors and Solutions
Error 1: Shape Mismatch
Description: The shapes of y_true
and y_pred
do not align, causing a shape mismatch error.
Solution: Ensure that the shapes of y_true
and y_pred
match, and consider using functions like tf.reduce_mean
or tf.reduce_sum
to obtain scalar values.
def custom_loss(y_true, y_pred):
# Check and handle shape mismatch
if y_true.shape != y_pred.shape:
y_true = tf.reshape(y_true, y_pred.shape)
# Rest of the loss computation
# ...
Error 2: Undefined Tensors
Description: The loss function involves undefined tensors or operations, leading to runtime errors.
Solution: Explicitly handle edge cases and ensure that all tensors used in the loss computation are well-defined. Consider using functions like tf.where
to handle conditionals.
def custom_loss(y_true, y_pred):
# Check and handle undefined tensors
y_true = tf.where(tf.math.is_finite(y_true), y_true, tf.zeros_like(y_true))
# Rest of the loss computation
# ...
Error 3: Vanishing or Exploding Gradients
Description: The loss function results in vanishing or exploding gradients during training.
Solution: Normalize the gradients by using techniques like gradient clipping or choosing appropriate activation functions to prevent extreme values.
optimizer = tf.keras.optimizers.Adam(clipvalue=1.0) # Example of gradient clipping
Conclusion
Creating custom loss functions in Keras/TensorFlow can be a powerful tool to improve your model’s performance. It allows you to incorporate domain-specific knowledge and cater to the unique characteristics of your data. Remember, the key to a good loss function is that it should be differentiable and should accurately represent the cost associated with an incorrect prediction.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.