Creating a Pandas DataFrame from a Numpy array How do I specify the index column and column headers
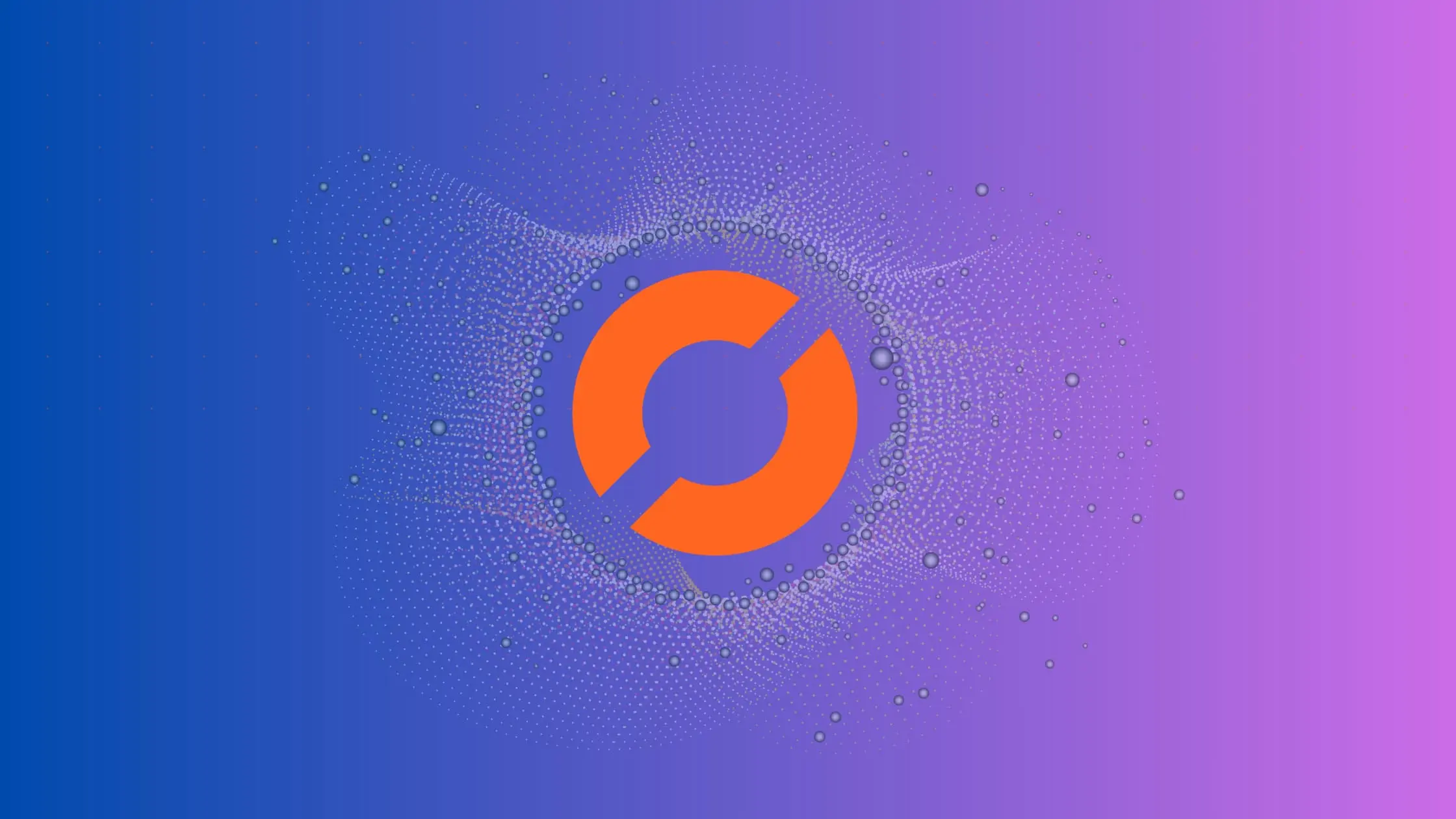
As a data scientist or software engineer, you may often find yourself working with large datasets that require efficient manipulation and analysis. One of the most popular tools for data analysis in Python is the Pandas library, which provides a powerful set of data structures and functions for working with tabular data. In this article, we will discuss how to create a Pandas DataFrame from a Numpy array and how to specify the index column and column headers.
Table of Contents
- What is a Pandas DataFrame?
- Creating a Pandas DataFrame from a Numpy array
- Specifying the index column
- Common Errors and How to Handle Them
- Conclusion
What is a Pandas DataFrame?
In Pandas, a DataFrame is a two-dimensional labeled data structure with columns of potentially different types. It is similar to a spreadsheet or SQL table, but with more powerful features for data manipulation and analysis. A DataFrame can be thought of as a dictionary of Series objects, where each Series represents a column of data.
Creating a Pandas DataFrame from a Numpy array
Numpy is a popular library for numerical computing in Python, and it provides a powerful array data structure for storing and manipulating large arrays of numerical data. To create a Pandas DataFrame from a Numpy array, you can use the pd.DataFrame()
function, which takes a Numpy array as input.
For example, let’s say we have the following Numpy array:
import numpy as np
data = np.array([[1, 2], [3, 4], [5, 6]])
To create a Pandas DataFrame from this array, we simply pass it to the pd.DataFrame()
function:
import pandas as pd
df = pd.DataFrame(data)
This creates a DataFrame with the same shape and data as the Numpy array:
>>> print(df)
0 1
0 1 2
1 3 4
2 5 6
By default, the DataFrame is created with integer column headers starting from 0. However, we can specify our own column headers by passing a list of column names to the columns
parameter:
df = pd.DataFrame(data, columns=['A', 'B'])
This creates a DataFrame with column headers ‘A’ and ‘B’:
>>> print(df)
A B
0 1 2
1 3 4
2 5 6
Specifying the index column
In addition to column headers, a Pandas DataFrame also has an index column, which identifies each row of data. By default, the index is created as a range of integers starting from 0, but we can specify our own index column by passing a list of index values to the index
parameter.
For example, let’s say we want to create a DataFrame with the same data as before, but with row labels ‘a’, ‘b’, and ‘c’:
df = pd.DataFrame(data, columns=['A', 'B'], index=['a', 'b', 'c'])
This creates a DataFrame with row labels ‘a’, ‘b’, and ‘c’:
>>> print(df)
A B
a 1 2
b 3 4
c 5 6
We can also specify the index column after creating the DataFrame by assigning a list of index values to the index
attribute:
df.index = ['x', 'y', 'z']
This changes the index column to ‘x’, ‘y’, and ‘z’:
>>> print(df)
A B
x 1 2
y 3 4
z 5 6
Common Errors and How to Handle Them
Error 1: Shape Mismatch
If the shape of the Numpy array doesn’t match the desired DataFrame shape, a ValueError will be raised. Double-check your array dimensions.
# Incorrect shape
invalid_array = np.array([[1, 2], [3, 4, 5]])
# Handling error
try:
df_invalid_shape = pd.DataFrame(invalid_array)
except ValueError as e:
print(f"ValueError: {e}")
Error 2: Incorrect Index Specification
Ensure that the index specified has the correct length and format. A mismatch will result in an IndexError.
# Incorrect index length
invalid_index = ['row1', 'row2']
# Handling error
try:
df_invalid_index = pd.DataFrame(data_array, index=invalid_index)
except IndexError as e:
print(f"IndexError: {e}")
Error 3: Duplicate Column Names
If your column headers contain duplicates, Pandas will throw a ValueError. Make sure each column has a unique name.
# Duplicate column headers
invalid_headers = ['col1', 'col2', 'col1']
# Handling error
try:
df_invalid_headers = pd.DataFrame(data_array, columns=invalid_headers)
except ValueError as e:
print(f"ValueError: {e}")
Conclusion
In summary, creating a Pandas DataFrame from a Numpy array is a straightforward process that can be done using the pd.DataFrame()
function. We can specify our own column headers and index column by passing lists of column names and index values to the columns
and index
parameters, respectively. By using these techniques, we can create customized DataFrames that are optimized for our specific data analysis needs.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.