Converting PySpark DataFrame Column to List: A Guide
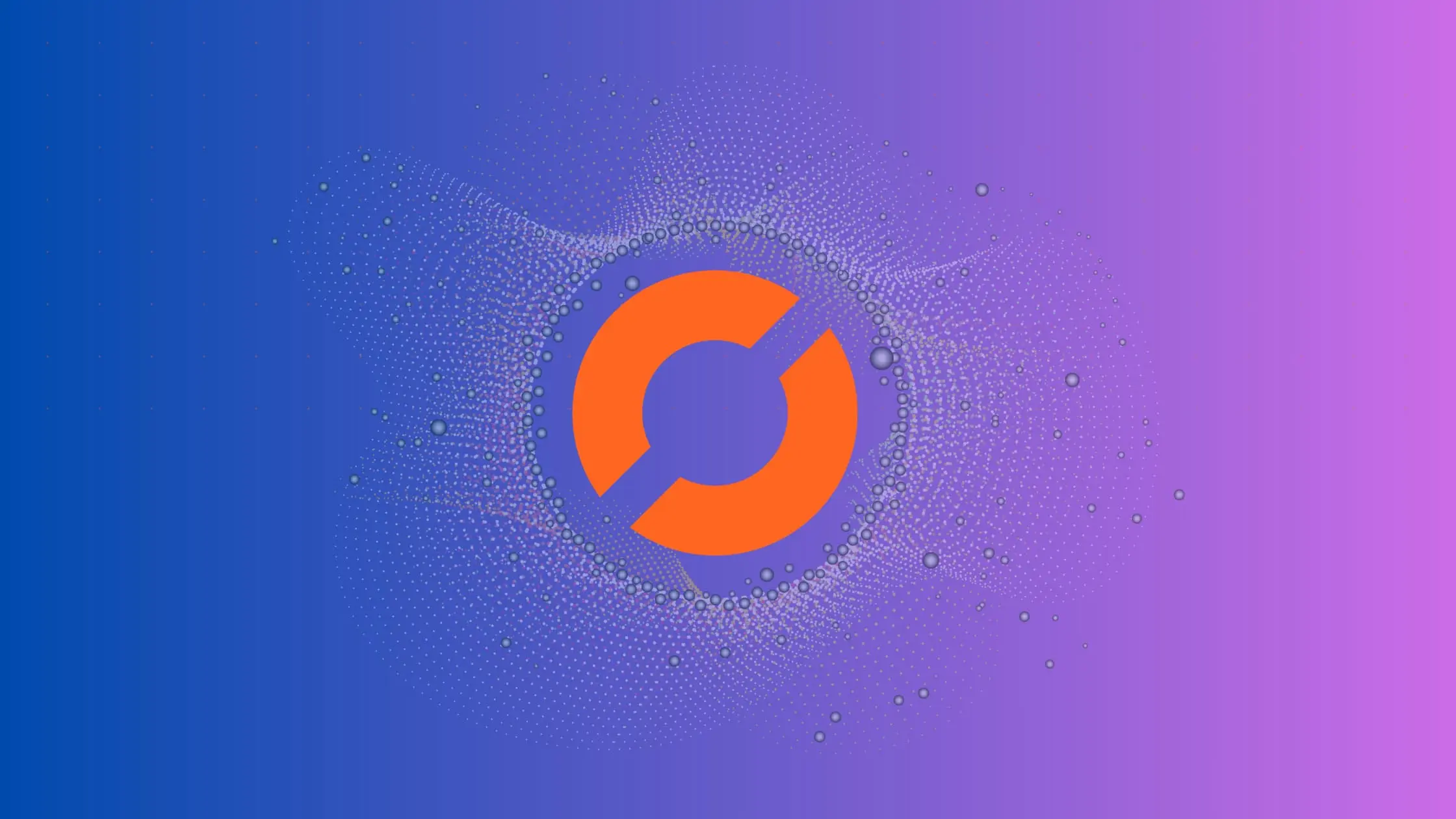
Data scientists often need to convert DataFrame columns to lists for various reasons, such as data manipulation, feature engineering, or even visualization. In this blog post, we’ll explore how to convert a PySpark DataFrame column to a list.
PySpark, the Python library for Apache Spark, is a powerful tool for large-scale data processing. It provides an interface for programming Spark with the Python programming language. With PySpark, you can create DataFrames, which are distributed collections of data organized into named columns.
Table of Contents
- Prerequisites
- Step 1: Importing Necessary Libraries
- Step 2: Creating a SparkSession
- Step 3: Creating a DataFrame
- Step 4: Converting DataFrame Column to List
- Best Practices
- Common Errors and How to Handle Them
- Conclusion
Prerequisites
Before we dive in, make sure you have the following:
- Apache Spark and PySpark installed on your system.
- A basic understanding of Python and PySpark DataFrames.
Step 1: Importing Necessary Libraries
First, we need to import the necessary libraries. We’ll need PySpark and its SQL functions.
from pyspark.sql import SparkSession
from pyspark.sql.functions import *
Step 2: Creating a SparkSession
Next, we create a SparkSession, which is the entry point to any PySpark functionality.
spark = SparkSession.builder.appName('PySparkTutorial').getOrCreate()
Step 3: Creating a DataFrame
For this tutorial, let’s create a simple DataFrame with two columns: ‘id’ and ‘value’.
data = [("1", "apple"), ("2", "banana"), ("3", "cherry")]
df = spark.createDataFrame(data, ["id", "value"])
df.show()
Output:
+---+------+
| id| value|
+---+------+
| 1| apple|
| 2|banana|
| 3|cherry|
+---+------+
Step 4: Converting DataFrame Column to List
Method 1: Using collect()
Now, let’s convert the ‘value’ column to a list. We can use the collect()
function to achieve this.
list_values = df.select("value").rdd.flatMap(lambda x: x).collect()
print(list_values)
Output:
['apple', 'banana', 'cherry']
The select()
function is used to select the column we want to convert to a list. The rdd
function converts the DataFrame to an RDD, and flatMap()
is a transformation operation that returns multiple output elements for each input element. The collect()
action operation returns all the elements of the RDD as an array to the driver program.
Method 2: Using select()
and rdd
Another approach involves using select()
to extract the desired column and then applying the rdd transformation. This method is more memory-efficient than collect()
.
column_list = df.select("your_column").rdd.map(lambda x: x[0]).collect()
print(list_values)
Output:
['apple', 'banana', 'cherry']
Best Practices
Memory Management: Be cautious with the use of collect(), especially on large datasets, as it can lead to memory overflow issues.
Select Only What You Need: Use the select() method to extract only the necessary columns, reducing the amount of data transferred.
Common Errors and How to Handle Them
Error 1: Memory Overflow
When dealing with large datasets, calling collect()
can lead to memory overflow. To handle this, consider using methods that distribute the computation across the Spark cluster, such as rdd.
Error 2: Null Values in the Column
If the column contains null values, you might encounter issues during conversion. Handle null values appropriately using PySpark functions like na.fill()
or filtering them out before conversion.
Conclusion
And there you have it! You’ve successfully converted a PySpark DataFrame column to a list. This technique is incredibly useful in many data processing tasks, and mastering it will make your data science journey with PySpark much smoother.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.