Converting Object Column in Pandas Dataframe to Datetime: A Comprehensive Guide
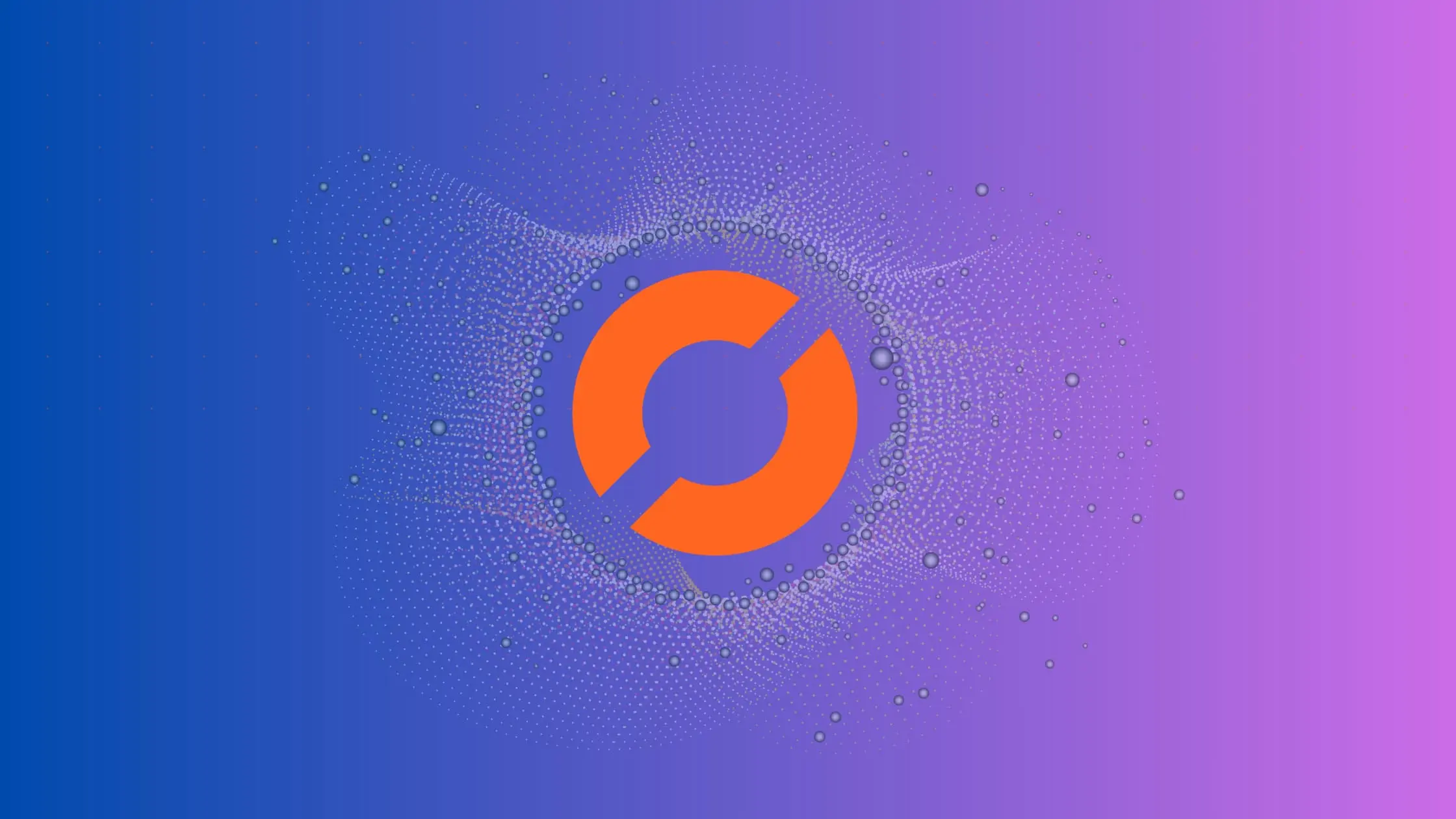
As a data scientist, one of the most common tasks you will encounter is working with dates and times. Often, you will need to convert date/time data stored in an object column in a pandas dataframe to a datetime format, which is much easier to work with. In this article, we will discuss why datetime format is necessary, how to convert object columns to datetime format, and some common challenges you may encounter during this process.
Why Use Datetime Format in Pandas Dataframe?
Before we dive into the conversion process, let’s first understand why datetime format is necessary in pandas dataframes. When you work with dates and times, you often need to perform calculations, filtering, and sorting based on specific time periods. Working with dates in their string format (object column) can be challenging and time-consuming. For example, if you want to sort a dataframe based on date, you may need to convert the dates to datetime format before sorting.
Datetime format is essential because it allows you to perform various operations on dates and times, such as addition, subtraction, sorting, and filtering, with ease. Therefore, converting object columns to datetime format is a crucial step in preparing your data for analysis.
How to Convert Object Column to Datetime in Pandas Dataframe
To convert an object column to datetime format in pandas, you can use the pd.to_datetime()
method. Let’s take an example dataframe with an object column containing date strings.
import pandas as pd
df = pd.DataFrame({'date': ['2022-05-01', '2022-05-02', '2022-05-03']})
# convert to datetime using pd.to_datetime
df['date'] = pd.to_datetime(df['date'])
print(df)
Output:
date
0 2022-05-01
1 2022-05-02
2 2022-05-03
As you can see, the date
column is now in datetime format. The pd.to_datetime()
method automatically detects the date format and converts it to datetime format.
Alternative Approaches:
1. Custom Parsing:
For more control over date format parsing, a custom parsing function can be implemented using the datetime.strptime() method from the datetime module:
from datetime import datetime
df['date'] = df['date'].apply(lambda x: datetime.strptime(x, '%Y-%m-%d'))
2. Using infer_datetime_format
:
df['date'] = pd.to_datetime(df['date'], infer_datetime_format=True)
This option attempts to infer the datetime format, reducing the need for specifying the format manually.
Common Challenges and Solutions
1. Non-Standard Date Formats
One common challenge you may face when converting object columns to datetime format is that the date strings may not be in the standard format (YYYY-MM-DD). In this case, the pd.to_datetime()
method may not be able to detect the date format automatically.
For example, let’s take the following dataframe with a date column in a non-standard format:
df = pd.DataFrame({'date': ['May 1, 2022', 'May 2, 2022', 'May 3, 2022']})
print(df)
Output:
date
0 May 1, 2022
1 May 2, 2022
2 May 3, 2022
To convert this column to datetime format, we need to specify the date format using the format
parameter of the pd.to_datetime()
method.
# convert to datetime using pd.to_datetime with predefined format
df['date'] = pd.to_datetime(df['date'], format='%B %d, %Y')
print(df)
Output:
date
0 2022-05-01
1 2022-05-02
2 2022-05-03
In this example, we used the format
parameter to specify the date format as Month Day, Year ('%B %d, %Y'
), and the pd.to_datetime()
method was able to convert the column to datetime format successfully.
2. Missing or Invalid Dates
Another challenge you may face when converting object columns to datetime format is missing or invalid dates. For example, let’s take the following dataframe:
df = pd.DataFrame({'date': ['2022-05-01', '2022-05-02', '2022-05-xx']})
print(df)
Output:
date
0 2022-05-01
1 2022-05-02
2 2022-05-xx
As you can see, the third row contains an invalid date (2022-05-xx
). When you try to convert this column to datetime format using the pd.to_datetime()
method, it will raise a ValueError
:
df['date'] = pd.to_datetime(df['date'])
Output:
ValueError: Unknown string format: 2022-05-xx
To handle missing or invalid dates, you can set the errors
parameter of the pd.to_datetime()
method to 'coerce'
. This will convert the missing or invalid dates to NaT
(Not a Time) values.
# convert to datetime using pd.to_datetime and handle missing datetime data
df['date'] = pd.to_datetime(df['date'], errors='coerce')
print(df)
Output:
date
0 2022-05-01
1 2022-05-02
2 NaT
As you can see, the third row has been converted to a NaT
value, indicating that the date is missing or invalid.
Conclusion
In this article, we discussed why datetime format is necessary in pandas dataframes and how to convert object columns to datetime format using the pd.to_datetime()
method. We also discussed some common challenges you may face during this process, such as non-standard date formats and missing or invalid dates, and their solutions. Converting object columns to datetime format is a crucial step in preparing your data for analysis, and by following the tips and tricks discussed in this article, you can do it with ease.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.