Converting Numpy Arrays to Images using CV2 and PIL
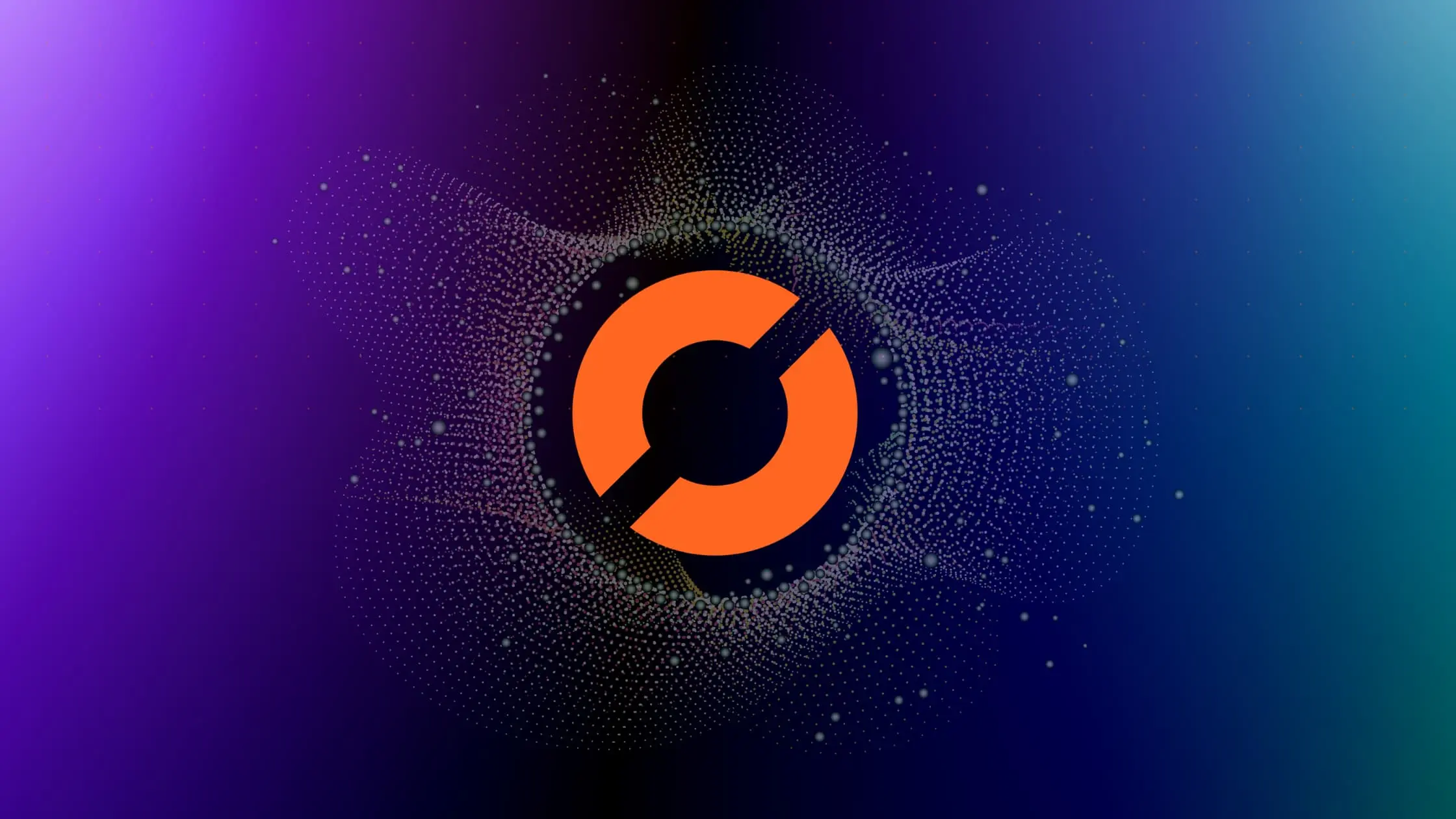
Data scientists often need to convert Numpy arrays to images for various tasks, such as image processing, machine learning, and computer vision. In this tutorial, we’ll explore how to accomplish this using two popular Python libraries: OpenCV (CV2) and Python Imaging Library (PIL).
Table of Contents
- Introduction
- Converting Numpy Arrays to Images with CV2
- Converting Numpy Arrays to Images with PIL
- Conclusion
Introduction
Numpy is a powerful library for numerical computing in Python. It provides support for arrays, which are often used to store image data. However, to visualize or save these arrays as images, we need to convert them into a format that image viewers understand. This is where OpenCV and PIL come in.
Converting Numpy Arrays to Images with CV2
OpenCV (Open Source Computer Vision Library) is an open-source computer vision and machine learning software library. It provides a function cv2.imwrite()
to save an image. The function takes two arguments: the image file path and the Numpy array containing the pixel values.
Here’s a simple example:
import cv2
import numpy as np
# Create a Numpy array
array = np.zeros([100, 200, 3], dtype=np.uint8)
array[:,:100] = [255, 128, 0] # Orange left side
array[:,100:] = [0, 0, 255] # Blue right side
# Convert array to image
cv2.imwrite('output.png', array)
In this code, we first create a 100x200 Numpy array with three channels (for RGB). We then fill the left half of the array with orange and the right half with blue. Finally, we use cv2.imwrite()
to save the array as an image.
Converting Numpy Arrays to Images with PIL
The Python Imaging Library (PIL) is another powerful library for opening, manipulating, and saving many different image file formats. To convert a Numpy array to an image with PIL, we use the Image.fromarray()
function.
Here’s how to do it:
from PIL import Image
import numpy as np
# Create a Numpy array
array = np.zeros([100, 200, 3], dtype=np.uint8)
array[:,:100] = [255, 128, 0] # Orange left side
array[:,100:] = [0, 0, 255] # Blue right side
# Convert array to image
img = Image.fromarray(array)
img.save('output.png')
In this code, we create the same Numpy array as before. However, this time we use Image.fromarray()
to convert the array to a PIL Image object. We then save the image with img.save()
.
Output:
Conclusion
In this tutorial, we’ve learned how to convert Numpy arrays to images using CV2 and PIL. Both libraries provide simple and effective ways to accomplish this task. The choice between them depends on your specific needs and which library you’re more comfortable with.
Remember, when working with images in Python, it’s crucial to understand the data structure you’re dealing with. Images are typically represented as Numpy arrays, with dimensions for height, width, and color channels. By mastering the conversion between Numpy arrays and images, you’ll be well-equipped to tackle a wide range of image processing tasks.
Stay tuned for more tutorials on data science and Python!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.