Converting Numpy Array Values into Integers: A Guide
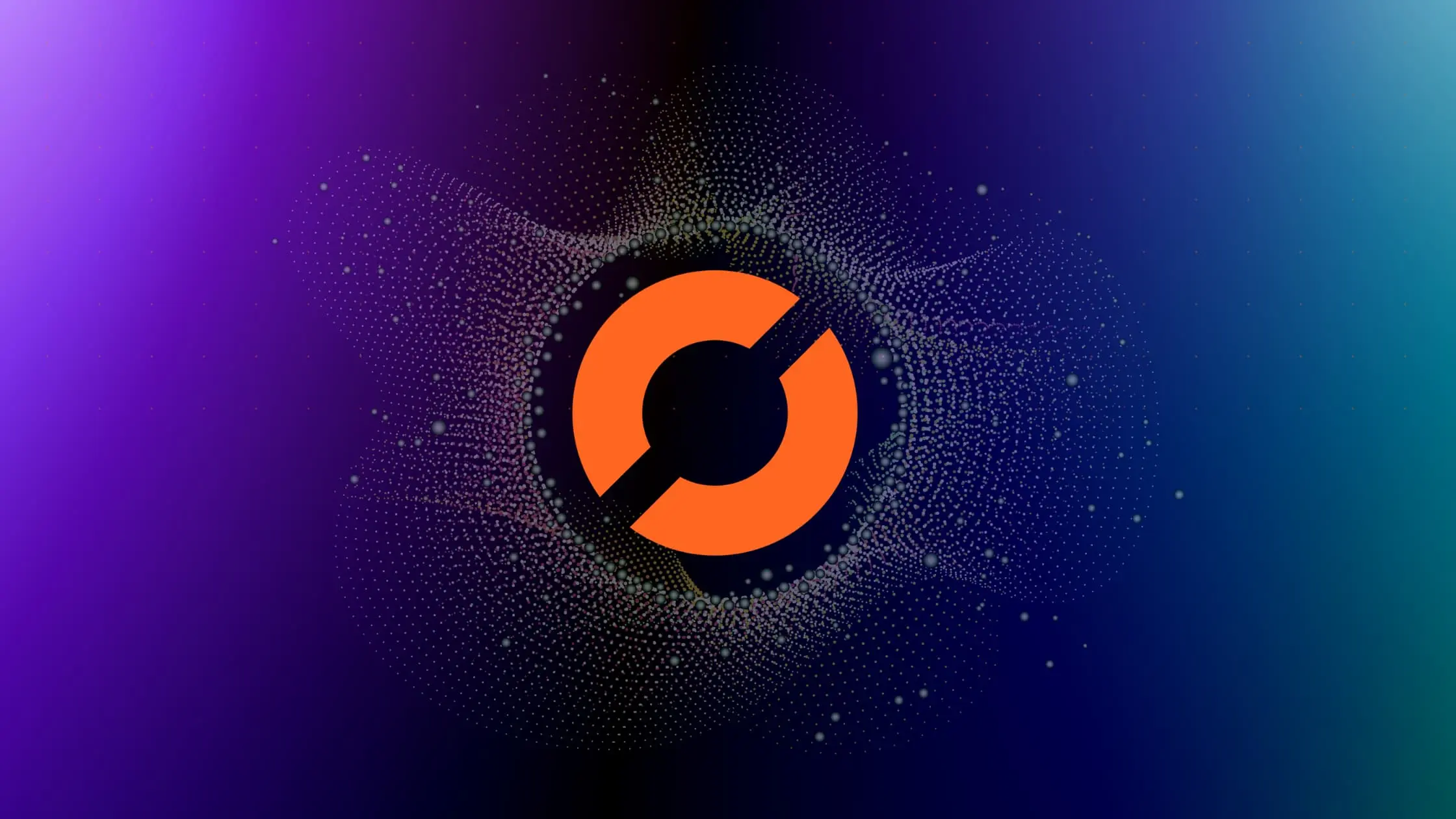
Data scientists often deal with a wide range of data types. One of the most common tasks is converting data types in Numpy arrays, specifically converting array values into integers. This blog post will guide you through the process, providing a step-by-step tutorial on how to convert Numpy array values into integers.
Table of Contents
- Introduction to Numpy
- Why Convert Numpy Array Values to Integers?
- Converting Numpy Array Values to Integers
- Rounding Before Converting to Integers
- Common Errors and How to Handle Them
- Conclusion
Introduction to Numpy
Numpy, short for Numerical Python, is a fundamental package for scientific computing in Python. It provides a high-performance multidimensional array object and tools for working with these arrays. Numpy is a powerful library that allows you to perform complex mathematical operations with ease.
Why Convert Numpy Array Values to Integers?
There are several reasons why you might want to convert the values in a Numpy array to integers:
- Data Preprocessing: In data science, preprocessing is a crucial step. Converting floating-point numbers to integers can help reduce the complexity of the data and make computations faster and more efficient.
- Memory Efficiency: Integer data types consume less memory compared to floating-point numbers. By converting your data to integers, you can optimize memory usage, especially when working with large datasets.
- Data Visualization: Some visualization libraries or functions may require integer inputs. Converting your data to integers ensures compatibility with these libraries.
Converting Numpy Array Values to Integers
Numpy astype()
Method:
Numpy provides several functions to convert the data types of numbers. The astype()
function is one of the most commonly used functions for this purpose. Let’s dive into how you can use this function to convert Numpy array values into integers.
import numpy as np
# Creating a sample array with float values
float_array = np.array([1.23, 4.56, 7.89])
# Converting float_array to integers
int_array = float_array.astype(int)
print("Original Array:", float_array)
print("Converted Array:", int_array)
When you run this code, you’ll get the following output:
Original Array: [1.23 4.56 7.89]
Converted Array: [1 4 7]
The astype(int)
function converts the floating-point numbers in the array to integers. Note that this function truncates the decimal part of the number, rather than rounding to the nearest integer.
Rounding Before Converting to Integers
If you want to round the numbers to the nearest integer before converting, you can use the round()
function before astype(int)
.
import numpy as np
# Creating a sample array with float values
float_array = np.array([1.23, 4.56, 7.89])
# Rounding and converting to integers
rounded_int_array = np.round(float_array).astype(int)
print("Original Array:", float_array)
print("Rounded and Converted Array:", rounded_int_array)
This code will output:
Original Array: [1.23 4.56 7.89]
Rounded and Converted Array: [1 5 8]
In this case, the number 4.56 and 7.89 are rounded up to 5 and 8 before being converted to an integer.
Common Errors and How to Handle Them
Error 1: Type Conversion Issues
If the array contains non-convertible elements, a ValueError will be raised. To handle this, you can use numpy.isnan or try-except blocks.
Error 2: Rounding Errors
When rounding, be cautious about precision issues that might arise. Adjust the precision parameter in numpy.round accordingly.
Leveraging Vectorized Operations:
Instead of iterating through each element individually, vectorized operations allow us to perform computations on entire arrays at once. For instance, multiplying a Numpy array by a scalar is a vectorized operation.
array = np.array([1.1, 2.2, 3.3, 4.4, 5.5])
# Convert the array values to integers
integer_array = (array * 1).astype(int)
In this snippet, the multiplication operation is applied element-wise, effectively converting each float value to an integer. The ‘leveraging’ here lies in the fact that the multiplication is broadcasted across the entire array, eliminating the need for explicit iteration.
Common Errors:
Precision Loss During Conversion:
Precision loss can occur when converting Numpy array values to integers, emphasizing the need for meticulous precision management. For example, converting a floating-point array with decimal values to integers might lead to rounding discrepancies. Consider the following code snippet:
import numpy as np
original_array = np.array([1.234, 2.567, 3.891])
integer_array = original_array.astype(int)
In this case, the precision of the original floating-point values is compromised during conversion, potentially resulting in unexpected discrepancies in the integer representation.
Handling Non-Numeric Data Challenges:
Dealing with non-numeric data during the conversion process presents unique challenges that demand careful consideration. For instance, attempting to convert an array containing strings or other non-numeric types using the astype() method can result in errors. Here’s an illustrative example:
import numpy as np
mixed_array = np.array([1, 'two', 3])
numeric_array = mixed_array.astype(int)
In this scenario, the attempt to convert a mixed array with string values into integers will raise a ValueError, highlighting the importance of pre-processing non-numeric data appropriately.
Mitigating Overflow and Underflow:
Mitigating overflow and underflow issues is crucial when working with large array values, ensuring the integrity of the conversion process. For instance, converting a Numpy array with excessively large or small values to integers might lead to overflow or underflow. Consider the following example:
import numpy as np
large_array = np.array([1e20, 2e30, 3e40])
integer_array = large_array.astype(int)
In this case, attempting to convert an array with values exceeding the integer range may result in overflow, leading to unexpected outcomes. Mitigating these issues involves careful scaling or utilizing alternative methods to handle extreme values appropriately.
Conclusion
Converting Numpy array values into integers is a common task in data science and machine learning. It helps in data preprocessing, memory efficiency, and data visualization. The astype(int)
function in Numpy makes this task straightforward and efficient. Remember to use the round()
function if you want to round the numbers to the nearest integer before converting.
We hope this guide has been helpful in understanding how to convert Numpy array values into integers. Stay tuned for more tutorials on data science and Numpy!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.