Converting from Numpy Array to PyTorch Tensor: A Guide
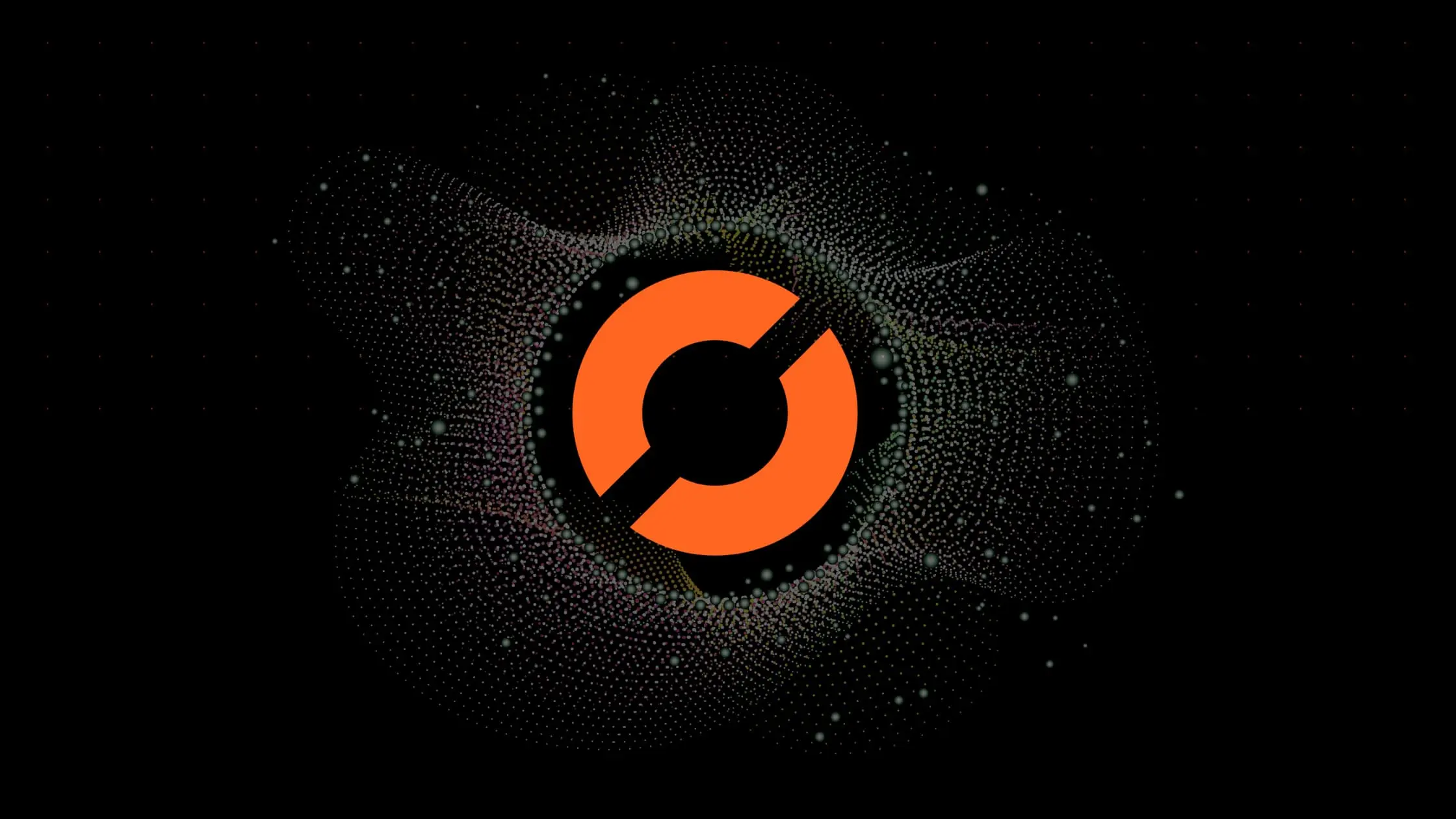
In the realm of data science, the ability to manipulate and convert data structures is a fundamental skill. Today, we’ll delve into the process of converting Numpy arrays to PyTorch tensors, a common requirement for deep learning tasks.
Table of Contents
- Introduction to Numpy and PyTorch
- Why Convert Numpy Arrays to PyTorch Tensors?
- Converting Numpy Arrays to PyTorch Tensors
- Things to Keep in Mind
- Conclusion
Introduction to Numpy and PyTorch
Numpy is a powerful Python library that provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. It’s a staple in the data science community for its efficiency and ease of use.
PyTorch, on the other hand, is an open-source machine learning library based on the Torch library. It’s known for its two high-level features: tensor computation with strong GPU acceleration and deep neural networks built on a tape-based autograd system.
Why Convert Numpy Arrays to PyTorch Tensors?
While Numpy is excellent for mathematical operations and data manipulation, it doesn’t natively support GPU acceleration, a significant disadvantage when dealing with large datasets and complex computations. PyTorch tensors, however, can utilize GPUs to accelerate their numeric computations. This feature is crucial for deep learning tasks, where computations are heavy and data is large.
Converting Numpy Arrays to PyTorch Tensors
Converting a Numpy array to a PyTorch tensor is straightforward, thanks to PyTorch’s built-in functions. Here’s a step-by-step guide:
Step 1: Import the Necessary Libraries
First, we need to import Numpy and PyTorch:
import numpy as np
import torch
Step 2: Create a Numpy Array
Next, let’s create a simple Numpy array:
numpy_array = np.array([1, 2, 3, 4, 5])
print(numpy_array)
Output:
[1 2 3 4 5]
Step 3: Convert to PyTorch Tensor
Now, we can convert the Numpy array to a PyTorch tensor using the from_numpy()
function:
pytorch_tensor = torch.from_numpy(numpy_array)
print(pytorch_tensor)
Output:
tensor([1, 2, 3, 4, 5])
And that’s it! You’ve successfully converted a Numpy array to a PyTorch tensor.
Things to Keep in Mind
While the conversion process is simple, there are a few things to keep in mind:
Data Type Consistency: PyTorch tensors and Numpy arrays will share their underlying memory locations, and changing one will change the other. However, they may not always have the same data type. Ensure to set the correct data type when creating your PyTorch tensor.
GPU Support: To leverage GPU acceleration, you need to move your tensor to the GPU memory using
.cuda()
. For example:
pytorch_tensor = pytorch_tensor.cuda()
- Backward Compatibility: You can convert PyTorch tensors back to Numpy arrays using the
.numpy()
method. But remember, the returned Numpy array and the original tensor share the same memory. Changes to one affect the other.
Conclusion
Converting Numpy arrays to PyTorch tensors is a simple yet powerful technique that allows data scientists to leverage the computational power of GPUs. With this guide, you’re now equipped to perform this conversion and optimize your deep learning tasks.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.