Converting Complex XML Files to Pandas DataFrame/CSV in Python
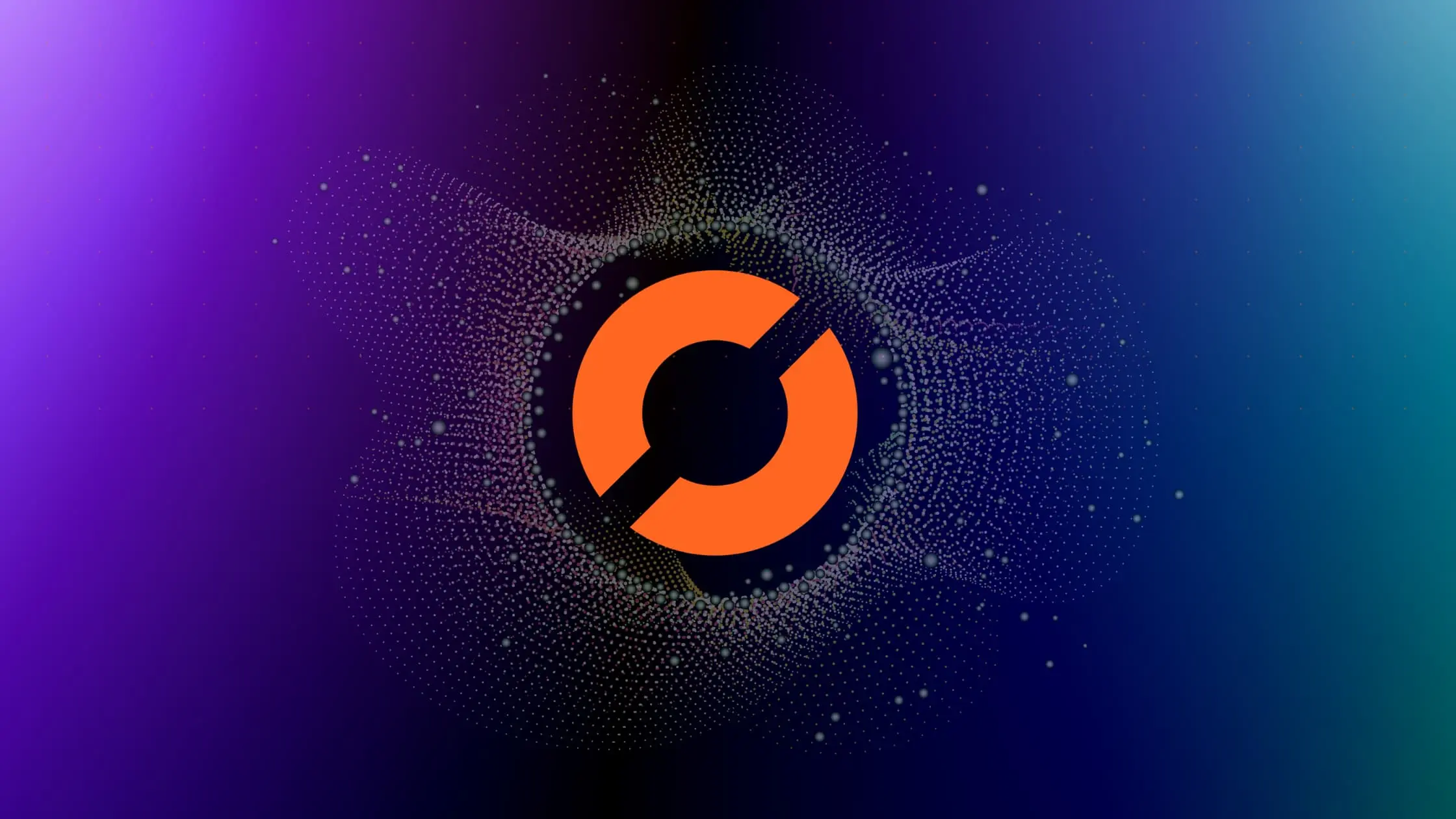
Table of Contents
- Prerequisites
- Step 1: Parsing the XML File
- Step 2: Extracting Data
- Step 3: Converting to DataFrame
- Step 4: Exporting to CSV
- Common Errors
- Conclusion
Prerequisites
Before we start, ensure you have the following Python libraries installed:
pandas
xml.etree.ElementTree
If not, you can install them using pip:
pip install pandas
pip install elementpath
Step 1: Parsing the XML File
The first step in converting an XML file to a DataFrame or CSV is parsing the XML file. We’ll use the xml.etree.ElementTree
module in Python, which provides a lightweight and efficient API for parsing and creating XML data.
import xml.etree.ElementTree as ET
def parse_xml(xml_file):
tree = ET.parse(xml_file)
root = tree.getroot()
return root
Step 2: Extracting Data
Once we have the root of the XML tree, we can extract the data we need. This process will vary depending on the structure of your XML file. For this example, let’s assume we have an XML file with a structure like this:
<data>
<record>
<field1>value1</field1>
<field2>value2</field2>
...
</record>
...
</data>
We can extract the data with the following function:
def extract_data(root):
data = []
for record in root.findall('record'):
row = {}
for field in record:
row[field.tag] = field.text
data.append(row)
return data
If you have a more complex structure with records of different variables you might need to customize which variable you want to extract from the XML file. Let’s look at the structure below:
<Root>
<Customer ...>
<field1>value1</field1>
<field2>value2</field2>
...
</Customers>
<Order ...>
<field1>value1</field1>
<field2>value2</field2>
...
</Orders>
</Root>
You would need to specify which variable you are trying to extract, whether Customer
or Order
def extract_data_custom(root, element_name):
data = []
for record in root.findall(f'.//{element_name}'):
row = {}
for field in record:
row[field.tag] = field.text
data.append(row)
return data
Step 3: Converting to DataFrame
With our data extracted from the XML file, we can now convert it into a Pandas DataFrame. This is as simple as passing our list of dictionaries to the pandas.DataFrame
constructor:
import pandas as pd
def to_dataframe(data):
df = pd.DataFrame(data)
return df
Step 4: Exporting to CSV
Finally, we can export our DataFrame to a CSV file using the to_csv
method:
def to_csv(df, filename):
df.to_csv(filename, index=False)
Putting It All Together
Here’s the complete code:
import xml.etree.ElementTree as ET
import pandas as pd
def parse_xml(xml_file):
tree = ET.parse(xml_file)
root = tree.getroot()
return root
def extract_data(root):
data = []
for record in root.findall('record'):
row = {}
for field in record:
row[field.tag] = field.text
data.append(row)
return data
def to_dataframe(data):
df = pd.DataFrame(data)
return df
def to_csv(df, filename):
df.to_csv(filename, index=False)
def main(xml_file, csv_file):
root = parse_xml(xml_file)
data = extract_data(root) # OR extract_data_custom(root, 'Customer') or `Order`
df = to_dataframe(data)
to_csv(df, csv_file)
if __name__ == "__main__":
main('data.xml', 'data.csv')
Common Errors
While working with XML files and converting them to DataFrames, you might encounter certain common errors. Here are a few notable ones and tips to address them:
- Parsing Errors:
- Missing File: Ensure the file path is correct and the file exists.
- Invalid File Format: Verify that the XML file has a valid structure and syntax. Use tools or online validators to check the XML format’s integrity.
- Data Extraction Errors:
- Non-existent Tags: Double-check the XML structure and confirm the tags being accessed exist.
- Type Mismatch: Handle data types appropriately during extraction. Use type conversion functions or conditional logic to ensure compatibility.
- Conversion Errors:
- Empty Data: Implement checks for empty data and handle them gracefully. Consider providing informative messages or default values.
- Duplicate Columns: Avoid creating duplicate keys in the DataFrame construction process. Use techniques to merge or handle duplicate column names.
- Saving Errors:
- Permission Issues: Verify write permissions for the output file’s location.
- Disk Space Issues: Ensure sufficient disk space is available for CSV creation.
General Tips:
- Use
try-except
blocks to catch potential errors and provide informative messages. - Validate data types during extraction and conversion.
- Thoroughly inspect the XML structure to understand its complexities.
- Test your code with diverse XML files to uncover potential issues early in development.
By understanding these common errors and implementing appropriate safeguards, you can enhance the robustness and reliability of your XML to DataFrame conversion process.
Conclusion
With this script, you can easily convert any complex XML file into a Pandas DataFrame or CSV file. This will make your data easier to work with and allow you to leverage the powerful data analysis capabilities of Python and Pandas.
Remember, the key to working with XML data is understanding its structure. Once you understand the structure of your XML file, you can easily extract the data you need and convert it into a more manageable format.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.