Converting a List of Dictionaries to a Pandas DataFrame: A Comprehensive Guide
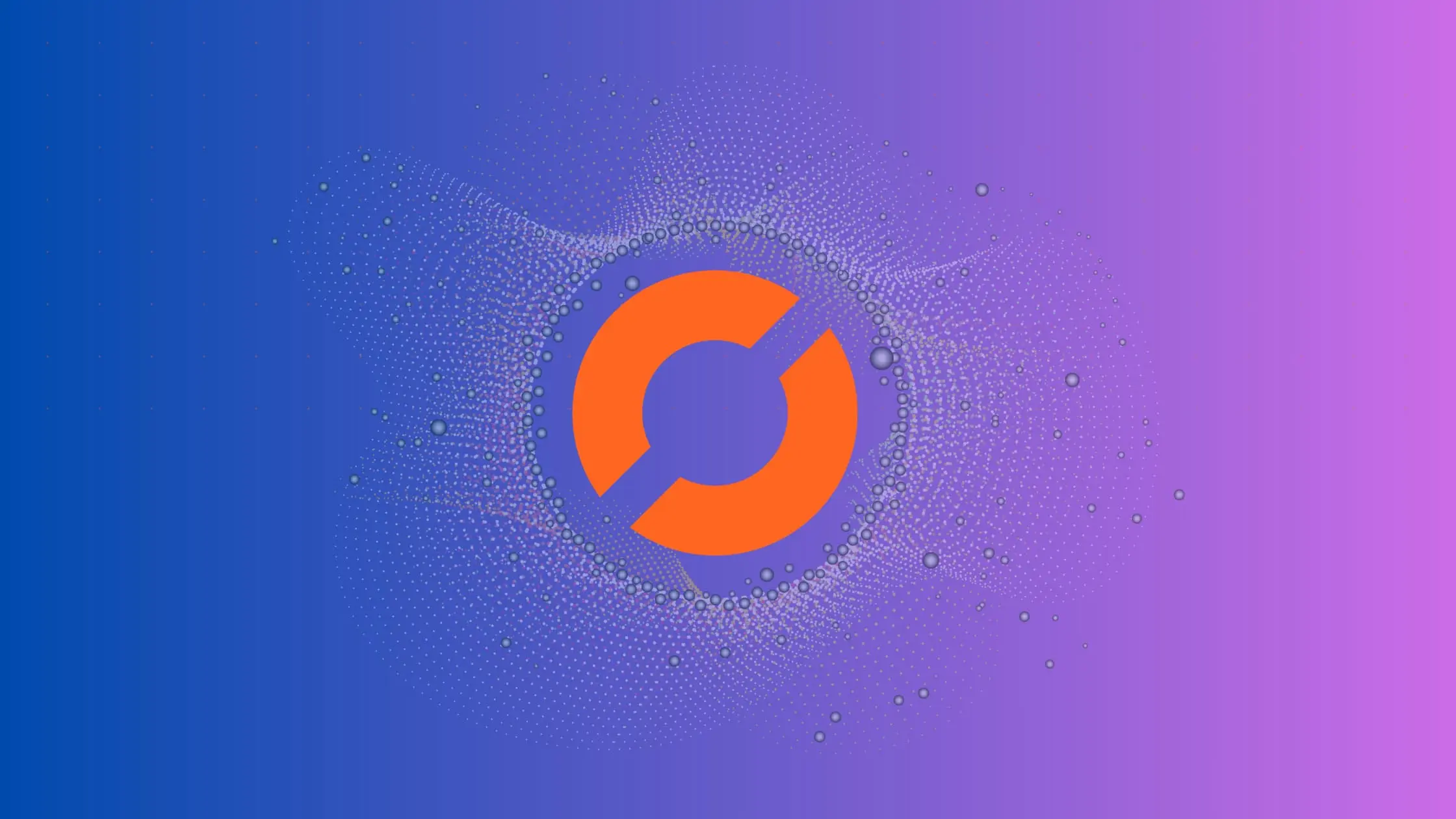
In the realm of data science, data manipulation is a fundamental skill. One common task is converting a list of dictionaries into a Pandas DataFrame. This comprehensive guide will walk you through the process, emphasizing the importance of setting one of the dictionary values as the column name for effective data analysis.
Why Convert a List of Dictionaries to a DataFrame?
Before we dive into the how, let’s discuss the why. While lists of dictionaries are common in Python, especially when handling JSON data, the Pandas DataFrame emerges as a more robust and flexible tool for data analysis and manipulation. With built-in functions for data cleaning, manipulation, and analysis, Pandas simplifies the entire process.
Step-by-Step Guide to Converting a List of Dictionaries to a DataFrame
Step 1: Import the Necessary Libraries
First, we need to import the Pandas library. If you haven’t installed it yet, you can do so using pip:
pip install pandas
Then, import it in your Python script:
import pandas as pd
Step 2: Define Your List of Dictionaries
For this guide, we’ll use a simple list of dictionaries. Each dictionary represents a person, with keys for ‘name’, ‘age’, and ‘city’:
people = [
{'name': 'Alice', 'age': 25, 'city': 'New York'},
{'name': 'Bob', 'age': 30, 'city': 'Chicago'},
{'name': 'Charlie', 'age': 35, 'city': 'Los Angeles'}
]
Step 3: Convert the List to a DataFrame
Converting the list to a DataFrame is as simple as passing it to the pd.DataFrame()
function:
df = pd.DataFrame(people)
This will create a DataFrame where the dictionary keys become column names, and the values become the rows of the DataFrame.
Step 4: Set a Dictionary Value as the Column Name
To set one of the dictionary values as the column name, we can use the set_index()
function. For example, to set ‘name’ as the column name:
df.set_index('name', inplace=True)
The inplace=True
argument modifies the original DataFrame, rather than creating a new one.
Output:
age city
name
Alice 25 New York
Bob 30 Chicago
Charlie 35 Los Angeles
Common Errors and Solutions:
Error 1: Inconsistent Dictionary Keys
Ensure that all dictionaries in the list have consistent keys. Inconsistent keys can lead to a DataFrame with missing or mislabeled columns.
people = [
{'name': 'Alice', 'age': 25, 'city': 'New York'},
{'name': 'Bob', 'age': 30, 'location': 'Chicago'},
{'name': 'Charlie', 'age': 35, 'city': 'Los Angeles'}
]
Notice that the second dictionary has a key named 'location'
instead of 'city'
. When attempting to convert this list to a Pandas DataFrame, you might encounter the following error:
ValueError: arrays must all be same length
Ensure that all dictionaries within the list have consistent keys. In this case, either update the 'location'
key to 'city'
or vice versa to maintain consistency.
Error 2: Missing Values
Handle missing or inconsistent values gracefully using Pandas functions like fillna()
or dropna()
.
people = [
{'name': 'Alice', 'age': 25, 'city': 'New York'},
{'name': 'Bob', 'age': 30},
{'name': 'Charlie', 'age': 35, 'city': 'Los Angeles'}
]
Handle missing or inconsistent values gracefully using Pandas functions. For instance, you can use the fillna()
function to replace NaN values with a default value or use dropna()
to remove rows with missing values.
df = pd.DataFrame(people).fillna('N/A')
# OR
df = pd.DataFrame(people).dropna()
Error 3: Data Type Mismatch
Address any data type mismatches, as Pandas attempts to infer data types during DataFrame creation.
people = [
{'name': 'Alice', 'age': '25', 'city': 'New York'},
{'name': 'Bob', 'age': '30', 'city': 'Chicago'},
{'name': 'Charlie', 'age': '35', 'city': 'Los Angeles'}
]
This may result in unexpected behavior or errors when performing numerical operations on the 'age'
column.
Ensure that the data types are consistent. Convert the 'age'
values to integers using the astype()
function:
df = pd.DataFrame(people)
df['age'] = df['age'].astype(int)
Conclusion
And there you have it! You’ve successfully converted a list of dictionaries into a Pandas DataFrame, with one of the dictionary values as the column name. This process is a fundamental part of data manipulation in Python, and mastering it will make your data analysis tasks much smoother.
Remember, the power of Pandas lies in its flexibility and functionality. Don’t hesitate to explore the Pandas documentation to learn more about what you can do with DataFrames.
Key Takeaways
- Lists of dictionaries are common in Python, but Pandas DataFrames offer more powerful data manipulation tools.
- Converting a list of dictionaries to a DataFrame is as simple as passing the list to
pd.DataFrame()
. - You can set a dictionary value as the column name using the
set_index()
function.
Next Steps
Now that you’ve mastered this process, why not explore more of what Pandas has to offer? Check out our other guides on topics like merging DataFrames, grouping and aggregating data, and handling missing data.
Happy data wrangling!
This blog post is part of our series on Python data manipulation. Stay tuned for more content on leveraging the power of Python for data science.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.