Converting a 2D Numpy Array to DataFrame Rows: A Guide
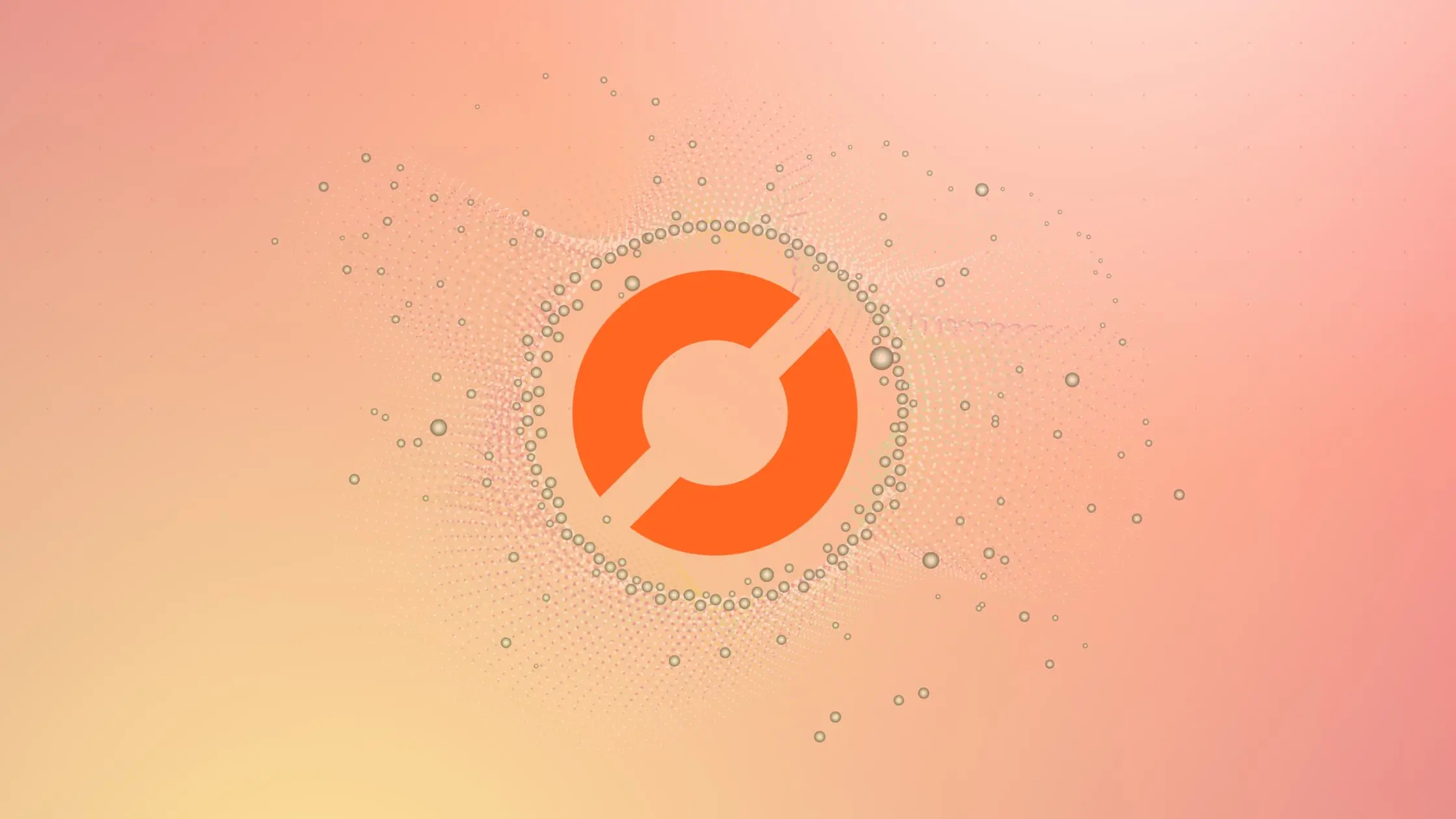
Data manipulation is a fundamental skill for any data scientist. One common task is converting a 2D Numpy array to DataFrame rows. This post will guide you through this process, step-by-step, using Python’s Pandas library.
Table of Contents
- Introduction
- Why Convert a 2D Numpy Array to DataFrame Rows?
- Step-by-Step Guide to Converting a 2D Numpy Array to DataFrame Rows
- Best Practices
- Common Errors and How to Handle Them
- Conclusion
- Further Reading
Introduction
Numpy and Pandas are two of the most widely used libraries in the Python data science ecosystem. Numpy provides support for large, multi-dimensional arrays and matrices, while Pandas is used for data manipulation and analysis. Converting between these two formats is a common task, and this guide will show you how to do it efficiently.
Why Convert a 2D Numpy Array to DataFrame Rows?
There are several reasons why you might want to convert a 2D Numpy array to DataFrame rows:
- Data Analysis: Pandas DataFrames provide a more intuitive interface for data analysis, with built-in functions for statistical analysis, data cleaning, and visualization.
- Data Preprocessing: Many machine learning libraries, such as Scikit-learn, require input data in DataFrame format.
- Data Storage: DataFrames can be easily exported to various file formats (CSV, Excel, SQL databases, etc.), making them ideal for data storage and sharing.
Step-by-Step Guide to Converting a 2D Numpy Array to DataFrame Rows
Step 1: Import the Necessary Libraries
First, we need to import the necessary libraries. If you haven’t installed Numpy and Pandas yet, you can do so using pip:
pip install numpy pandas
Then, import them into your Python script:
import numpy as np
import pandas as pd
Step 2: Create a 2D Numpy Array
For this guide, we’ll create a simple 2D Numpy array. In practice, you might be working with data loaded from a file or generated by a function.
array = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print(array)
Output:
[[1 2 3]
[4 5 6]
[7 8 9]]
Step 3: Convert the 2D Numpy Array to a DataFrame
Now, we can convert the 2D Numpy array to a DataFrame using the pd.DataFrame()
function:
df = pd.DataFrame(array)
print(df)
Output:
0 1 2
0 1 2 3
1 4 5 6
2 7 8 9
By default, the DataFrame will have integer column names (0, 1, 2, etc.). If you want to specify column names, you can pass them as a list to the columns
parameter:
df = pd.DataFrame(array, columns=['Column1', 'Column2', 'Column3'])
print(df)
Output:
Column1 Column2 Column3
0 1 2 3
1 4 5 6
2 7 8 9
Best Practices
- Define Column Names: Always define column names to avoid ambiguity and ensure data integrity.
- Consistent Data Types: Ensure that the Numpy array has consistent data types for each column.
Common Errors and How to Handle Them
Shape Mismatch
If the shape of the array does not match the expected shape for DataFrame rows, a ValueError will occur. Handle this by reshaping or transposing the array.
import pandas as pd
import numpy as np
data = np.array([[1, 2],
['John', 'Jane'],
[25, 30]])
# Transpose the array to match the expected shape
df = pd.DataFrame(data.T, columns=['ID', 'Name', 'Age'])
print(df)
Output:
ID Name Age
0 1 John 25
1 2 Jane 30
Missing Column Names
Omitting column names in the conversion can lead to confusion and errors. Provide column names explicitly during conversion.
Mixed Data Types
Pandas DataFrames require consistent data types within each column. Handle mixed data types by converting them to a common type or using a structured Numpy array.
import pandas as pd
import numpy as np
data = np.array([(1, 'John', 25),
(2, 'Jane', '30'), # Age as a string
(3, 'Bob', 22)])
# Convert the age column to int
df = pd.DataFrame.from_records(data, columns=['ID', 'Name', 'Age'])
df['Age'] = df['Age'].astype(int)
print(df)
Output:
ID Name Age
0 1 John 25
1 2 Jane 30
2 3 Bob 22
Conclusion
Converting a 2D Numpy array to DataFrame rows is a common task in data science. This guide has shown you how to do it step-by-step. Remember, the key is to use the pd.DataFrame()
function, which can convert a 2D Numpy array to a DataFrame in a single line of code.
Further Reading
If you want to learn more about Numpy and Pandas, check out the following resources:
- Numpy Documentation
- Pandas Documentation
- Python for Data Analysis by Wes McKinney, creator of Pandas.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.