Convert Column to Timestamp Pandas Dataframe
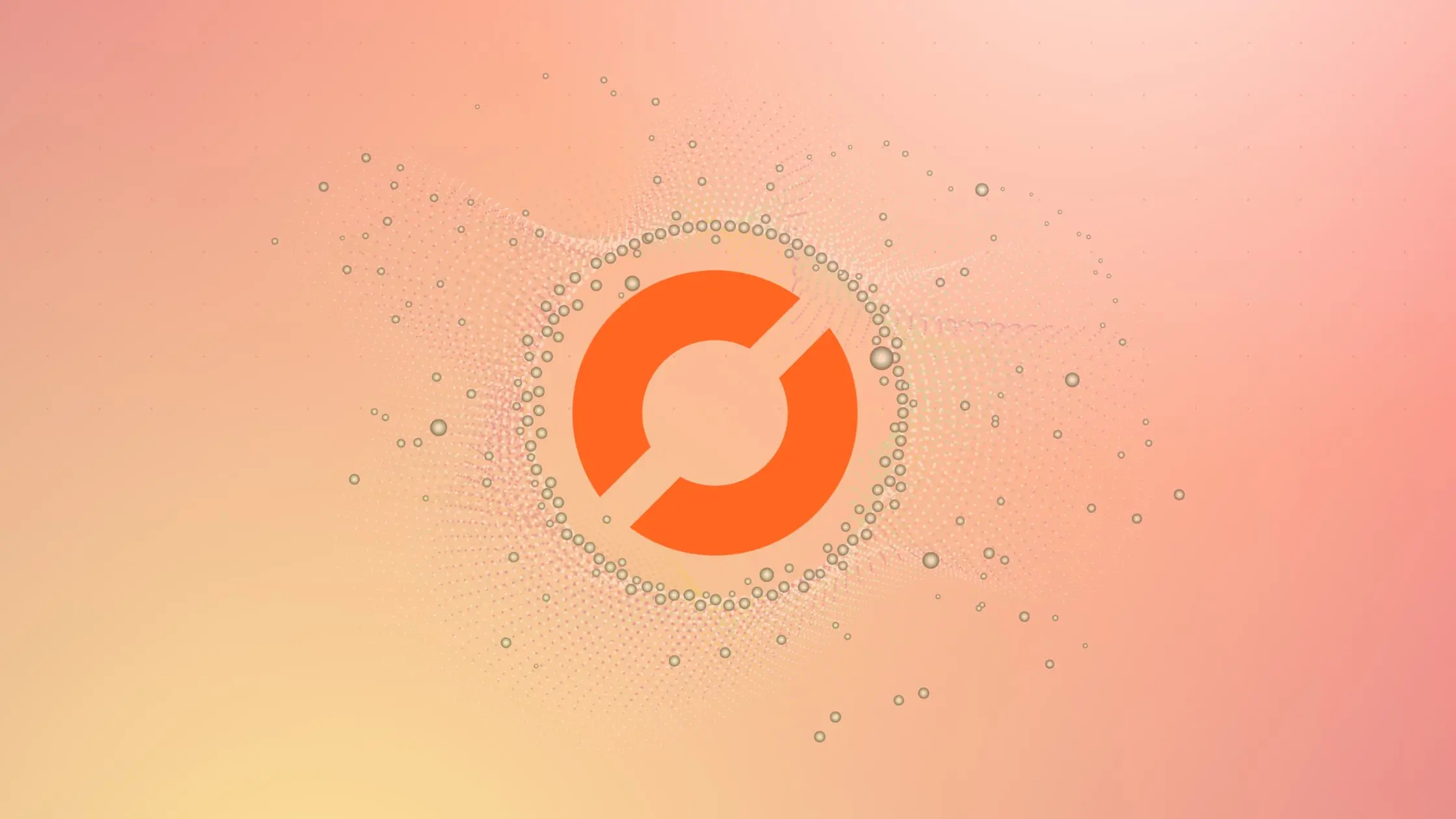
As a data scientist or software engineer, working with large datasets is a common task. Often, the data we work with contains information in various formats, which we need to transform before we can use it effectively. One common scenario is working with dates and times, which often come in a variety of formats. In this article, we will explore how to convert a column to a timestamp in a Pandas Dataframe.
Table of Contents
- What is a Timestamp?
- Why Convert a Column to Timestamp?
- How to Convert a Column to Timestamp
- Handling Time Zones
- Common Errors and Solutions
- Conclusion
What is a Timestamp?
A timestamp is a data structure that represents a specific point in time. It is often used in data analysis and processing to represent dates and times. A timestamp can be represented in different formats, such as Unix time, ISO 8601, and others.
Why Convert a Column to Timestamp?
In many cases, data is stored as strings or other data types that are not directly usable as timestamps. For example, a date might be stored as a string in the format “YYYY-MM-DD”, or as a Unix timestamp in seconds since the epoch. Converting these data types to a timestamp allows us to perform date and time arithmetic, filtering, and other operations more easily.
How to Convert a Column to Timestamp
In Pandas, converting a column to a timestamp is a straightforward process. First, we need to identify the column that contains the date or time data. In this example, we will use a sample dataset containing a column of dates in the format “YYYY-MM-DD HH:MM:SS”.
import pandas as pd
# create sample dataframe
data = {'date': ['2022-01-01 00:00:00', '2022-01-02 01:00:00', '2022-01-03 02:00:00']}
df = pd.DataFrame(data)
# print original dataframe
print(df)
print("----------")
print(df.dtypes)
Output:
date
0 2022-01-01 00:00:00
1 2022-01-02 01:00:00
2 2022-01-03 02:00:00
----------
date object
dtype: object
We can see that the date
column is currently stored as a string. To convert it to a timestamp, we can use the to_datetime
function from Pandas. This function can parse a variety of date and time formats, and convert them to timestamps.
# convert string to timestamp
df['date'] = pd.to_datetime(df['date'])
# print updated dataframe
print(df)
print("----------")
print(df.dtypes)
Output:
date
0 2022-01-01 00:00:00
1 2022-01-02 01:00:00
2 2022-01-03 02:00:00
----------
date datetime64[ns]
dtype: object
We can see that the date
column has been converted to a timestamp, represented as a datetime64 data type.
Handling Time Zones
In some cases, the original date or time data may not include a time zone. When converting to a timestamp, Pandas will assume the data is in the local time zone. To specify a different time zone, we can use the tz_localize
function.
# create sample dataframe with no time zone info
data = {'date': ['2022-01-01 00:00:00', '2022-01-02 01:00:00', '2022-01-03 02:00:00']}
df = pd.DataFrame(data)
# convert string to timestamp with specified time zone
df['date'] = pd.to_datetime(df['date']).dt.tz_localize('UTC')
# print updated dataframe
print(df)
Output:
date
0 2022-01-01 00:00:00+00:00
1 2022-01-02 01:00:00+00:00
2 2022-01-03 02:00:00+00:00
We can see that the date
column now includes the UTC time zone information.
Common Errors and Solutions
Error 1: ValueError - Inferred frequency is in the future This error occurs when Pandas infers a frequency that is not valid for the given data. To solve this, explicitly set the
errors
parameter tocoerce
, which will replace invalid parsing withNaT
(Not a Time).df['date'] = pd.to_datetime(df['date'], errors='coerce')
Error 2: ValueError - day is out of range for month This error happens when the day value in the date is not valid for the specified month. To address this, set the
errors
parameter tocoerce
to handle invalid dates.df['date'] = pd.to_datetime(df['date'], errors='coerce')
Error 3: ValueError - time data ‘invalid_date’ does not match format When the date format is not consistent, specify a format using the
format
parameter. This helps Pandas parse the dates correctly.df['date'] = pd.to_datetime(df['date'], format='%Y-%m-%d', errors='coerce')
Conclusion
Converting a column to a timestamp in a Pandas Dataframe is a simple and powerful way to work with date and time data. By using the to_datetime
function, we can convert a variety of formats to timestamps, allowing us to perform date and time arithmetic, filtering, and other operations more easily. We can also handle time zones by using the tz_localize
function. With this knowledge, you can efficiently work with date and time data in your data analysis and processing tasks.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.