Convert a Tensor to a Numpy Array in Tensorflow
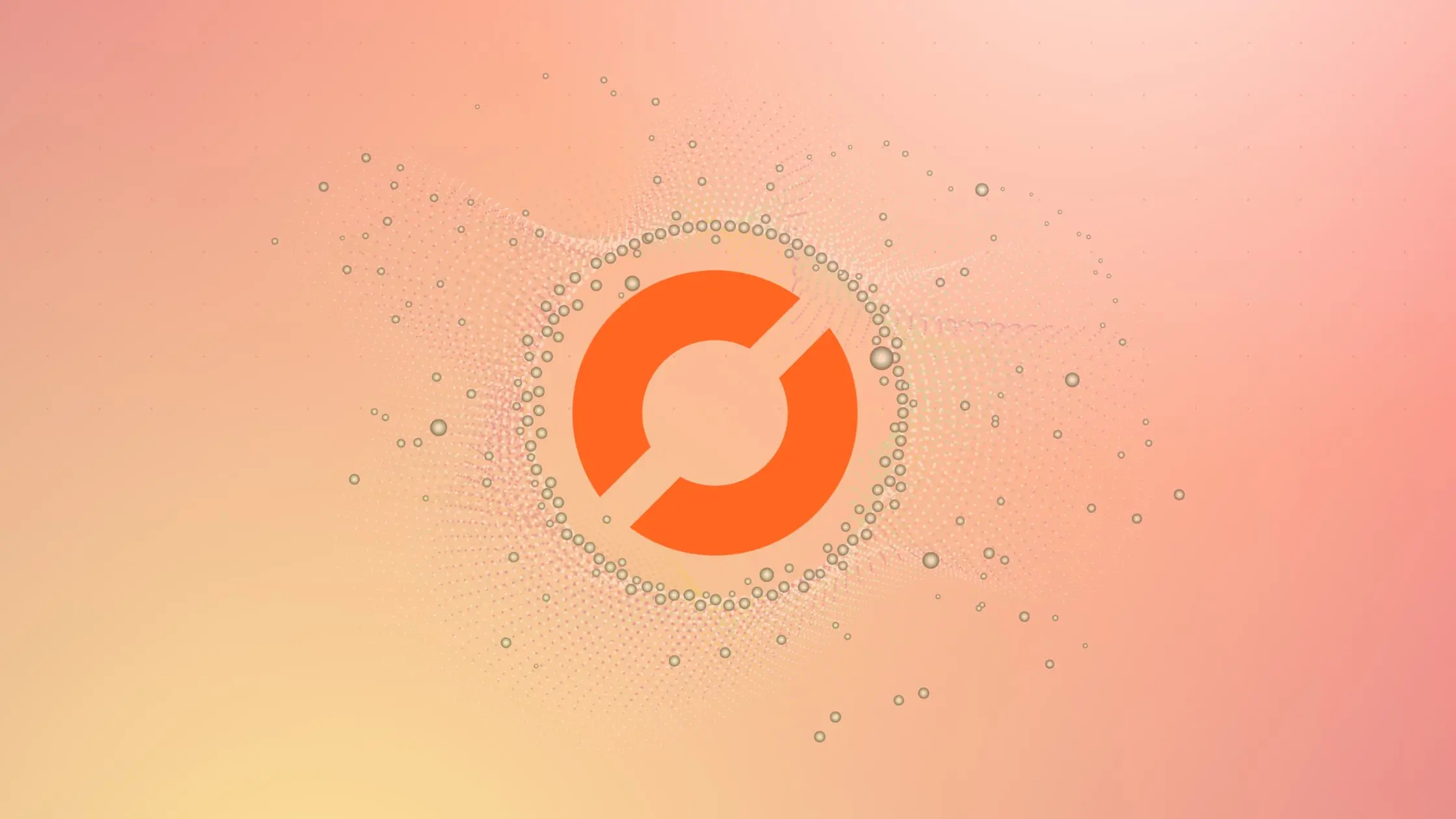
As a data scientist working with TensorFlow, you’ll often need to work with tensors, which are multi-dimensional arrays that represent the inputs and outputs of your TensorFlow models. However, there may be times when you need to convert a tensor to a NumPy array, which is a fundamental data structure in Python for numerical computing.
In this article, we’ll explore how to convert a tensor to a NumPy array in TensorFlow. We’ll cover the basics of tensors and NumPy arrays, as well as the different methods you can use to convert between the two.
What are Tensors?
Tensors are the fundamental data structures used in TensorFlow for representing data with multiple dimensions. Tensors can be thought of as multi-dimensional arrays, where each element in the array is identified by a set of indices.
Tensors are used to represent the inputs and outputs of your TensorFlow models, as well as the intermediate values computed during training or inference. Tensors can have any number of dimensions, from 0 (a scalar) to n (a tensor with n dimensions).
What are NumPy Arrays?
NumPy is a fundamental library in Python for numerical computing. NumPy provides a powerful array object that can be used to represent arrays of any dimensionality. NumPy arrays are similar to tensors in TensorFlow, in that they can represent multi-dimensional arrays of data.
NumPy arrays are used in a wide range of scientific and engineering applications, including machine learning, data analysis, and simulations. NumPy arrays are also used extensively in TensorFlow, as they provide a flexible and efficient way to represent data.
Converting a Tensor to a NumPy Array in TensorFlow
There are several ways to convert a tensor to a NumPy array in TensorFlow, depending on the context and the requirements of your application. Here are some of the most common methods:
Using the numpy() Method
The simplest way to convert a tensor to a NumPy array in TensorFlow is to use the numpy()
method of the tensor object. The numpy()
method returns a NumPy array with the same shape and elements as the tensor.
import tensorflow as tf
import numpy as np
# Create a tensor
tensor = tf.constant([[1, 2], [3, 4]])
# Convert the tensor to a NumPy array
numpy_array = tensor.numpy()
# Print the NumPy array
print(numpy_array)
Output:
[[1, 2],
[3, 4]]
Pros:
- Simplicity: This method is straightforward and easy to use, making it suitable for quick conversions.
- Readability: The code is concise, making it easy to understand for beginners and experienced developers alike.
- Direct Integration: The
numpy()
method is a native function of the TensorFlow tensor object, providing seamless integration.
Cons:
- Limited Customization: This method may not offer advanced customization options compared to other approaches.
- Performance: For large tensors, using this method might be less efficient than other options.
Using tf.make_ndarray() Function
Another way to convert a tensor to a NumPy array in TensorFlow is to use the tf.make_ndarray()
function. The tf.make_ndarray()
function takes a tensor and returns a NumPy array with the same shape and elements as the tensor.
import tensorflow as tf
import numpy as np
# Create a tensor
tensor = tf.constant([[1, 2], [3, 4]])
# Convert `tensor` to a proto tensor
proto_tensor = tf.make_tensor_proto(tensor)
# Convert the tensor to a NumPy array
numpy_array = tf.make_ndarray(proto_tensor)
# Print the NumPy array
print(numpy_array)
Output:
[[1 2]
[3 4]]
Pros:
- Explicit Conversion: The use of a dedicated function (
tf.make_ndarray()
) explicitly indicates the intention to convert a tensor to a NumPy array. - Compatibility: This method is useful when you need to work with lower-level TensorFlow functions and manipulate the tensor proto.
Cons:
- Additional Steps: It involves an extra step to convert the tensor to a proto tensor before obtaining the NumPy array.
- Complexity: The usage of a dedicated function may be perceived as slightly more complex than the
numpy()
method.
Using tf.Session() Object
In recent versions of TensorFlow, refrain from using tensor.eval()
to evaluate a tensor, as it is no longer recommended. Instead, opt for the use of tf.Session()
for this purpose. Here’s how you can do it:
import tensorflow as tf
import numpy as np
# need to disable eager in TF2.x
tf.compat.v1.disable_eager_execution()
# Create a tensor
tensor = tf.constant([[1, 2], [3, 4]])
# Create a session
sess = tf.compat.v1.Session()
# Convert the tensor to a NumPy array
numpy_array = sess.run(tensor)
# Print the NumPy array
print(numpy_array)
Output:
[[1 2]
[3 4]]
Pros:
- Versatility: The
tf.Session()
approach allows for more flexibility in evaluating tensors and performing additional operations within the session context. - Compatibility: Useful when working with older versions of TensorFlow or when eager execution is disabled.
Cons:
- Deprecated in TF2.x: The use of
tf.Session()
is discouraged in recent TensorFlow versions (TF2.x), and it requires disabling eager execution. - Code Verbosity: Compared to the other methods, using sessions introduces more boilerplate code.
Conclusion
In this article, we’ve explored how to convert a tensor to a NumPy array in TensorFlow. We’ve covered the basics of tensors and NumPy arrays, as well as the different methods you can use to convert between the two.
Whether you’re working on a machine learning project or a data analysis task, knowing how to convert between tensors and NumPy arrays is an essential skill for any data scientist working with TensorFlow. With the methods and techniques we’ve covered in this article, you’ll be able to easily convert between tensors and NumPy arrays in your TensorFlow projects.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.