Combining two Series into a DataFrame in pandas
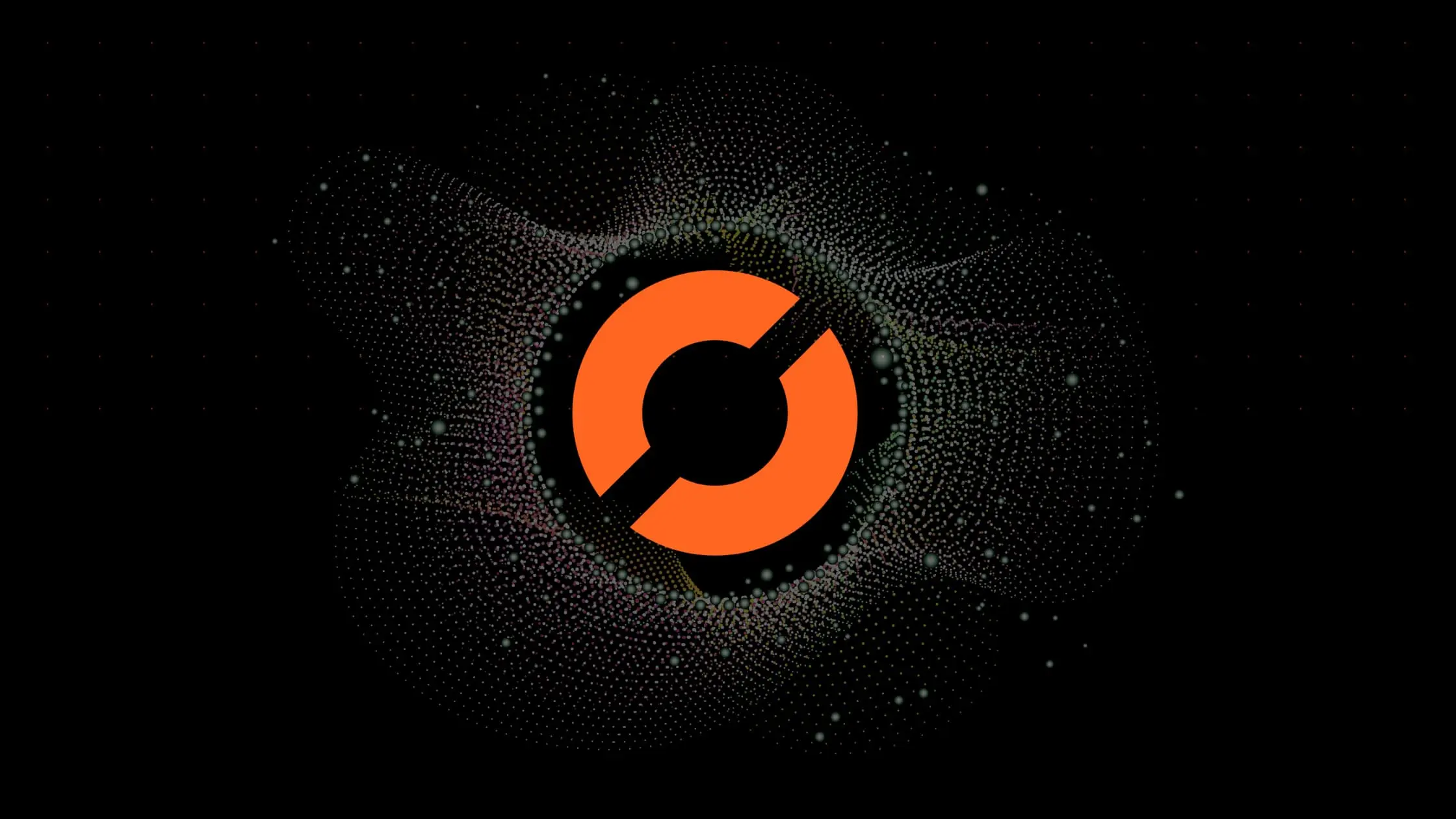
Table of Contents
Introduction to Series in pandas
Before we dive into how to combine two Series into a DataFrame, let’s quickly review what a Series is in pandas. A Series is a one-dimensional array-like object that can hold any data type, such as integers, floats, strings, or even Python objects. Each element in a Series is assigned a label, which is referred to as the index. The index can be used to access specific elements in the Series.
Here’s an example of creating a Series in pandas:
import pandas as pd
s = pd.Series([1, 2, 3, 4, 5], index=['a', 'b', 'c', 'd', 'e'])
print(s)
Output:
a 1
b 2
c 3
d 4
e 5
dtype: int64
In this example, we created a Series containing the integers 1 through 5, with the index labels ‘a’ through ‘e’.
How to combine two Series into a DataFrame
To combine two Series into a DataFrame in pandas, we can use the pd.DataFrame
constructor, pd.concat()
function, or pd.merge()
function. We will explore each one below.
Using pd.DataFrame() constructor:
We can combine two series into a dataframe by creating a new dataframe and passing the series as columns. Here’s an example below0:
import pandas as pd
# Creating two sample Series
series1 = pd.Series([1, 2, 3])
series2 = pd.Series(['A', 'B', 'C'])
# Combining the Series into a DataFrame using the DataFrame constructor
df = pd.DataFrame({'Column1': series1, 'Column2': series2})
# Display the resulting DataFrame
print(df)
This will produce the DataFrame below:
Column1 Column2
0 1 A
1 2 B
2 3 C
Using pd.concat()
This function concatenates two or more Series or DataFrames along a specific axis.
Here’s an example of concatenating two Series into a DataFrame:
import pandas as pd
s1 = pd.Series([1, 2, 3])
s2 = pd.Series(['A', 'B', 'C'])
df = pd.concat([s1, s2], axis=1)
print(df)
Output:
0 1
0 1 A
1 2 B
2 3 C
In this example, we created two Series s1
and s2
. We then used pd.concat()
to concatenate s1
and s2
along axis 1 (columns) to create a DataFrame df
. The resulting DataFrame has two columns, with the values from s1
in the first column and the values from s2
in the second column.
Note that the index labels from the original Series are preserved in the resulting DataFrame.
Using pd.merge()
This is more applicable when you have two Series with a common index. For example:
import pandas as pd
# Creating two sample Series with a common index
index = [0, 1, 2]
series1 = pd.Series([1, 2, 3], name='Column1', index=index)
series2 = pd.Series(['A', 'B', 'C'], name='Column2', index=index)
# Combining the Series into a DataFrame using pd.merge()
df = pd.merge(series1, series2, left_index=True, right_index=True)
# Display the resulting DataFrame
print(df)
This will also produce the same DataFrame:
Column1 Column2
0 1 A
1 2 B
2 3 C
Common Errors
Mismatched lengths: Attempting to combine Series with different lengths will result in errors. Ensure both Series have the same number of elements or specify how to handle mismatched rows/columns (e.g., dropping or filling).
Non-unique indexes: When using pd.merge(), duplicate index values can lead to unexpected results. Ensure unique indexes or specify how to handle duplicates (e.g., first/last occurrence).
Index mismatch with constructor: The pd.DataFrame constructor assumes aligned indexes for the provided Series. Mismatched indexes will cause errors.
Incompatible data types: Trying to combine Series with incompatible data types (e.g., integers and strings) can lead to coercion errors or unexpected conversions. Convert data to a compatible type before merging.
Bonus Tip: Always test your merging operation with a small sample of your data before applying it to the entire dataset to avoid surprises and wasted processing time.
Pros and Cons
- pd.DataFrame Constructor:
Pros: simple and ideal for quick and straightforward merges, familiar for those already comfortable with creating DataFrames.
Cons: limited flexibility as it requires aligned indexes and matching column names, not suitable for complex merges
- pd.concat():
Pros: handles merging on both rows and columns and supports different data types. Also allows specifying axis, join methods, and handling of missing values.
Cons: complex operations might require detailed parameter specifications and choosing the wrong axis can lead to unexpected results.
- pd.merge():
Pros: efficient for joins with shared indexes and easy to use for simple joins with matching column names.
Cons: less beginner-friendly as it requires understanding of join types and index alignment. Also not suitable for axis-based merging or handling mismatched data types.
Choosing the Right Method: The ideal method for merging your Series depends on your specific needs and data structure. Consider these factors:
Complexity of the merge: Simpler merges might benefit from the constructor’s ease, while complex operations might require pd.concat()’s flexibility.
Data types and indexes: If your Series have mismatched data types or indexes, pd.concat() or specific conversion steps might be necessary.
Join type: For relational joins with shared indexes, pd.merge() is often the most efficient choice.
By understanding the pros, cons, and appropriate use cases of each method, you can confidently choose the right tool for your Series merging needs, streamlining your data analysis workflow.
Conclusion
In this blog post, we’ve explored how to combine two Series into a DataFrame in pandas using three methods: pd.DataFrame
constructor, pd.concat()
function, or pd.merge()
function. This can be a useful operation when you have two sets of data that you would like to analyze together. Choose the method that best fits your specific use case and data structure.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.