Combining Numpy Arrays into a Pandas DataFrame: A Guide for Data Scientists
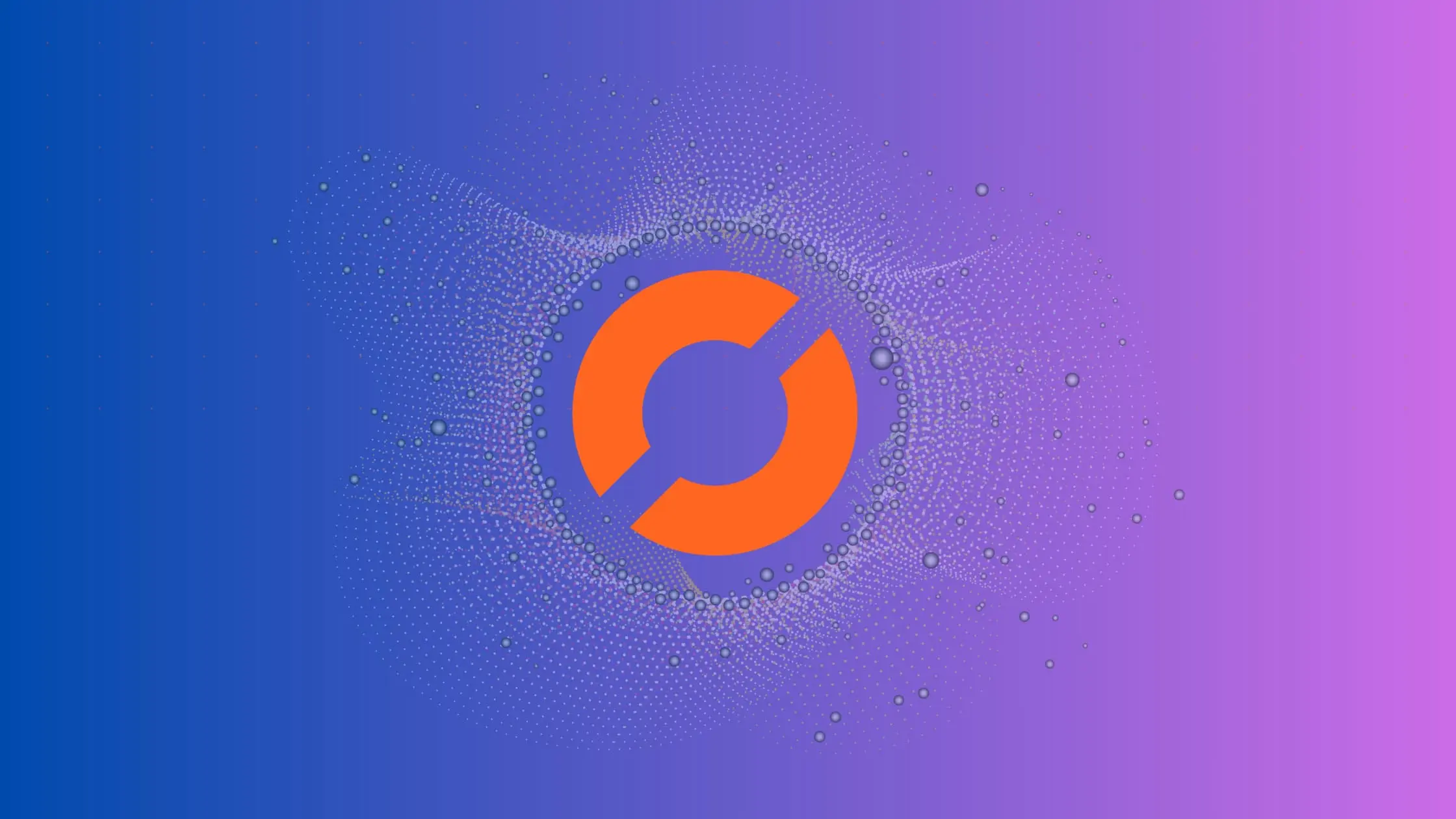
Data scientists often encounter the need to convert Numpy arrays into a Pandas DataFrame. However, sometimes these arrays come in a peculiar format that can make this process a bit challenging. In this blog post, we’ll explore how to handle such situations effectively.
Table of Contents
- Introduction
- Understanding the Challenge
- Step-by-Step Guide
- Best Practices
- Common Errors and Solutions
- Conclusion
Introduction
Numpy and Pandas are two of the most widely used libraries in Python for data manipulation. Numpy provides support for large, multi-dimensional arrays and matrices, while Pandas is used for data manipulation and analysis, particularly for manipulating numerical tables and time series.
While both libraries are powerful in their own right, there are times when you might need to convert data from a Numpy array into a Pandas DataFrame. This is especially true when the data is in a strange format. In this guide, we’ll walk you through the process of doing just that.
Understanding the Challenge
Let’s say you have a Numpy array in a format that isn’t immediately compatible with a Pandas DataFrame. For instance, you might have a 3D array, or an array of arrays, or perhaps an array with complex numbers. These are not formats that Pandas can handle natively, so we need to do some preprocessing before we can convert them into a DataFrame.
Step-by-Step Guide
Step 1: Import the Necessary Libraries
First, we need to import the necessary libraries. We’ll need Numpy for handling the arrays and Pandas for creating the DataFrame.
import numpy as np
import pandas as pd
Step 2: Creating Numpy Arrays
For the purpose of this guide, let’s create some sample Numpy arrays:
import numpy as np
array1 = np.array([[1, 2], [3, 4]])
array2 = np.array([[5, 6], [7, 8]])
Step 3: Importing Pandas and Numpy
Import the necessary libraries:
import pandas as pd
import numpy as np
Step 4: Combining Numpy Arrays into a Pandas DataFrame
4.1. Horizontal Stack
df_horizontal = pd.DataFrame(np.hstack((array1, array2)), columns=['A', 'B', 'C', 'D'])
4.2. Vertical Stack
df_vertical = pd.DataFrame(np.vstack((array1, array2)), columns=['A', 'B'])
4.3. Combining Arrays with Different Shapes
array3 = np.array([9, 10])
df_concat = pd.concat([df_horizontal, pd.DataFrame(array3, columns=['E'])], axis=1)
Best Practices
- Consistent Column Names: Ensure that the column names are consistent across arrays to avoid confusion during merging.
- Data Type Alignment: Check that the data types of columns match between arrays to prevent unexpected type errors.
Common Errors and Solutions
Shape Mismatch
Error: ValueError: all the input array dimensions for the concatenation axis must match exactly.
Solution: Verify that the dimensions of arrays being combined align along the specified axis.
Incorrect Axis Alignment
Error: ValueError: Shape of passed values is (X, Y), indices imply (A, B).
Solution: Double-check the axis parameter in functions like hstack
and vstack
to ensure proper alignment.
Duplicate Column Names
Error: ValueError: Index has duplicates.
Solution: Make sure there are no duplicate column names to prevent ambiguity in DataFrame creation.
Conclusion
Combining Numpy arrays into Pandas DataFrames is a vital skill for any data scientist. By understanding best practices, common errors, and exploring detailed examples, you can streamline your data preprocessing workflow and handle various scenarios with confidence. Mastering this process contributes to the efficiency and effectiveness of your data manipulation tasks.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.