Check if String in List of Strings is in a Pandas DataFrame Column: A Guide
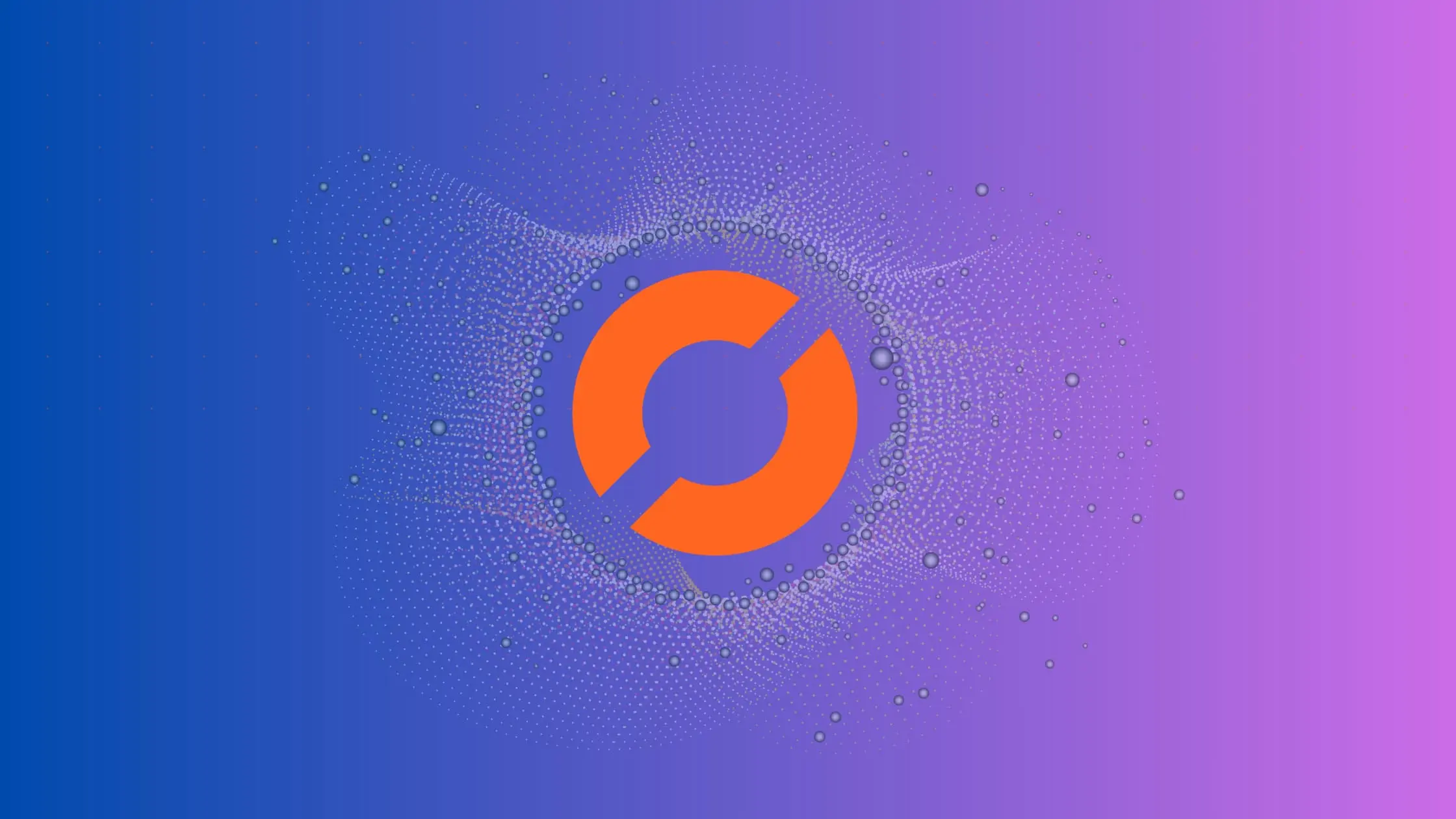
In the world of data science, it’s common to encounter scenarios where you need to check if a string from a list of strings is present in a Pandas DataFrame column. This task may seem simple, but it can be tricky, especially when dealing with large datasets. This blog post will guide you through the process, providing a step-by-step tutorial on how to accomplish this task efficiently.
Table of Contents
- Prerequisites
- Step 1: Importing the Necessary Libraries
- Step 2: Creating a Pandas DataFrame
- Step 3: Creating a List of Strings
- Step 4: Checking if String in List of Strings is in DataFrame Column
- Step 5: Filtering the DataFrame Based on the Condition
- Common Error and Solution
- Conclusion
Prerequisites
Before we dive in, make sure you have the following:
- Python installed (preferably Python 3.6 or later)
- Pandas library installed
- Basic understanding of Python and Pandas
Step 1: Importing the Necessary Libraries
First, we need to import the necessary libraries. In this case, we only need Pandas.
import pandas as pd
Step 2: Creating a Pandas DataFrame
For the purpose of this tutorial, let’s create a simple DataFrame.
data = {'Name': ['John', 'Anna', 'Peter', 'Linda', 'James']}
df = pd.DataFrame(data)
print(df)
This will create a DataFrame with a single column ‘Name’ containing five names.
Output:
Name
0 John
1 Anna
2 Peter
3 Linda
4 James
Step 3: Creating a List of Strings
Next, we create a list of strings. These are the strings we will check for in the DataFrame column.
list_of_strings = ['Anna', 'James', 'Michael']
Step 4: Checking if String in List of Strings is in DataFrame Column
Now, we come to the main part of the tutorial. We will use the isin()
function provided by Pandas. This function checks whether each element in the DataFrame is contained in the passed list of strings.
df['Name'].isin(list_of_strings)
This will return a Series of Boolean values. True if the string is in the list, and False if not.
Step 5: Filtering the DataFrame Based on the Condition
If you want to filter the DataFrame based on this condition, you can do so as follows:
filtered_df = df[df['Name'].isin(list_of_strings)]
print(filtered_df)
This will return a DataFrame containing only the rows where the ‘Name’ is in the list of strings.
Output:
Name
1 Anna
4 James
Common Error and Solution
Error : Case Sensitivity
By default, string matching is case-sensitive. If case-insensitive matching is desired, it may lead to incorrect results.
Example Code:
# Case-sensitive matching
list_of_strings = ['anna', 'james', 'michael']
df['Name'].isin(list_of_strings)
Solution:
# Convert both DataFrame column and list of strings to lowercase
df['Name'] = df['Name'].str.lower()
list_of_strings = [s.lower() for s in list_of_strings]
df['Name'].isin(list_of_strings)
Conclusion
In this blog post, we’ve covered how to check if a string from a list of strings is present in a Pandas DataFrame column. This is a common task in data science and understanding how to do it efficiently can save you a lot of time.
We hope you found this guide helpful. If you have any questions or comments, feel free to leave them below.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.