Calculating the Accuracy of PyTorch Models Every Epoch
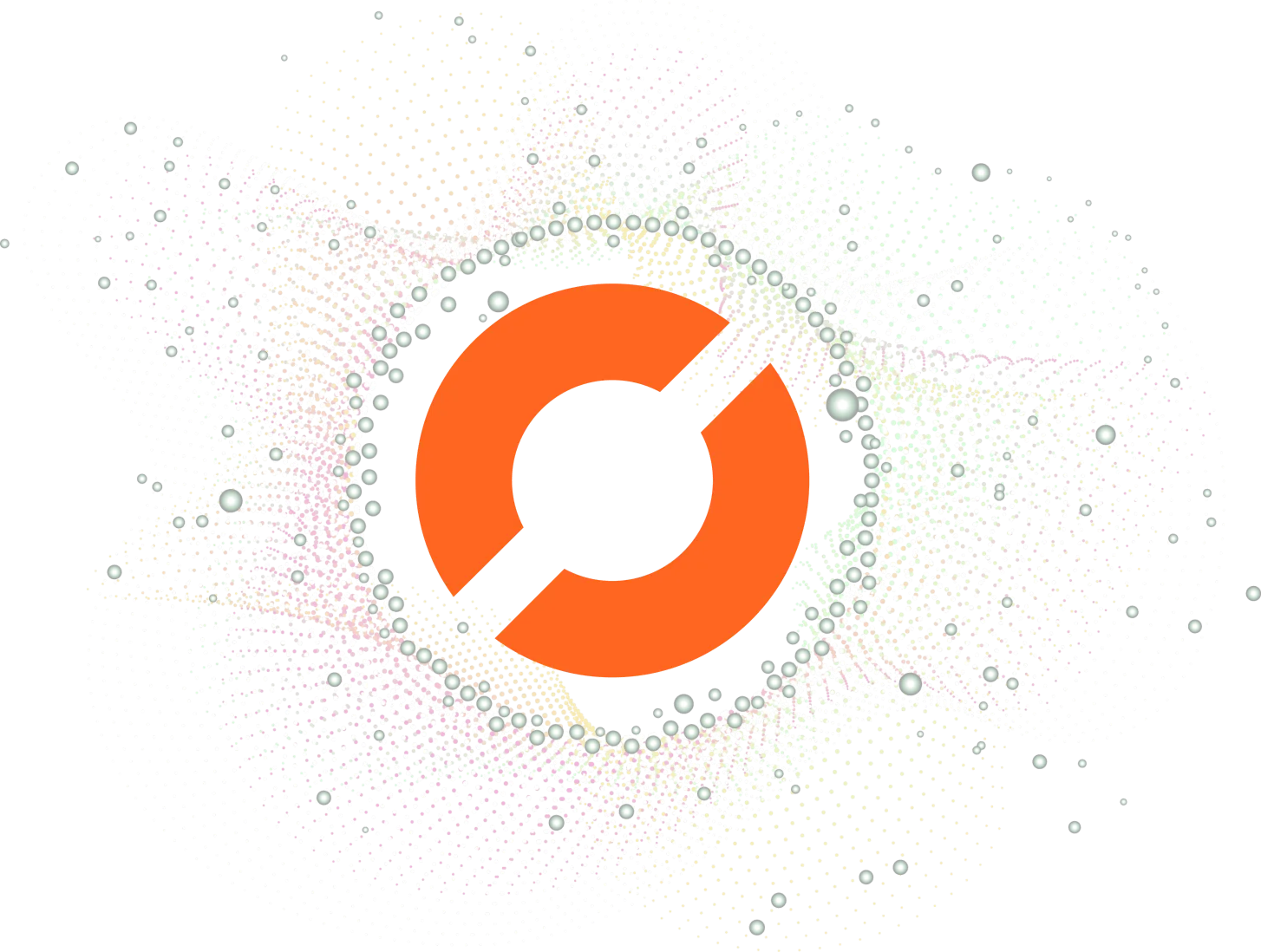
As a data scientist, you may be familiar with PyTorch, a popular open-source machine learning library that allows you to build and train deep learning models. One of the most important metrics in evaluating the performance of a model is its accuracy. In this blog post, we will discuss how to calculate the accuracy of a PyTorch model every epoch.
Table of Contents
- What is Accuracy?
- Calculating Accuracy in PyTorch
- Visualizing Accuracy
- Common Errors and Solutions
- Best Practices
- Conclusion
What is Accuracy?
Accuracy is a common metric used in classification tasks to evaluate how well a model classifies data into the correct classes. It is defined as the percentage of correctly classified samples out of all the samples in the dataset. The formula for accuracy is:
accuracy = (number of correctly classified samples) / (total number of samples)
For example, if you have a dataset with 100 samples and your model correctly classifies 80 of them, then the accuracy of your model is 80%.
Calculating Accuracy in PyTorch
In order to calculate the accuracy of a PyTorch model, we need to compare the predicted labels with the actual labels for each batch of data during training. PyTorch provides a simple way to do this using the torch.argmax
function, which returns the index of the maximum value in a tensor along a specified dimension.
Assuming that you have already defined your PyTorch model and dataloader, here’s how you can calculate the accuracy every epoch:
import torch
# Define your PyTorch model, optimizer, and loss function here
for epoch in range(num_epochs):
total_correct = 0
total_samples = 0
for images, labels in dataloader:
# Move the data to the device (CPU or GPU)
images = images.to(device)
labels = labels.to(device)
# Forward pass
outputs = model(images)
_, predicted = torch.max(outputs, 1)
# Update the running total of correct predictions and samples
total_correct += (predicted == labels).sum().item()
total_samples += labels.size(0)
# Calculate the accuracy for this epoch
accuracy = 100 * total_correct / total_samples
print(f'Epoch {epoch+1}: Accuracy = {accuracy:.2f}%')
Let’s break down the code above. We first define two variables total_correct
and total_samples
to keep track of the number of correctly classified samples and the total number of samples, respectively. We then loop over the batches of data in the dataloader, move the data to the device (CPU or GPU), and perform a forward pass through the model to get the predicted labels using torch.max
.
We then update the running total of correct predictions and samples by comparing the predicted labels with the actual labels using the ==
operator and summing up the number of matches using the sum
function. Finally, we calculate the accuracy for this epoch by dividing the total number of correct predictions by the total number of samples and multiplying by 100 to get a percentage.
Visualizing Accuracy
Once you have calculated the accuracy for each epoch, it’s a good idea to visualize the results using a graph. This can help you identify if your model is overfitting or underfitting, and whether you need to adjust the learning rate or other hyperparameters.
Here’s an example of how you can plot the accuracy using the popular matplotlib
library:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot(range(num_epochs), accuracies)
ax.set_xlabel('Epoch')
ax.set_ylabel('Accuracy')
ax.set_title('Accuracy per Epoch')
plt.show()
Assuming that you have stored the accuracies for each epoch in a list called accuracies
, the code above will create a simple line plot of the accuracy per epoch.
Common Errors and Solutions
1. Tensor Shape Mismatch Error:
Error Code:
outputs = model(images)
_, predicted = torch.max(outputs, 1) # Assuming 2 classes, which may not be true
Solution: Ensure that the number of classes in your model output matches the expected number. You can modify the code as follows:
outputs = model(images)
_, predicted = torch.max(outputs, dim=1)
2. GPU Memory Exhaustion:
Error Code:
images = images.to(device)
labels = labels.to(device)
Solution: If you encounter GPU memory issues, consider moving the model and optimizer to the device only once outside the batch loop:
model = model.to(device)
optimizer = optimizer(model.parameters(), lr=learning_rate)
Best Practices
1. Regular Model Evaluation:
Regularly evaluate your model on a separate validation set to monitor its performance. This helps in detecting issues early and allows for adjustments.
2. Learning Rate Scheduling:
Implement learning rate scheduling to dynamically adjust the learning rate during training. This can prevent overshooting or slow convergence.
3. Data Augmentation:
Apply data augmentation techniques to enhance the model’s generalization capabilities. PyTorch provides torchvision.transforms for convenient data augmentation.
4. Early Stopping:
Implement early stopping based on the validation accuracy to prevent overfitting. Save the model when the validation accuracy improves and stop training if there’s no improvement after a certain number of epochs.
5. Model Checkpointing:
Save model checkpoints during training. This allows you to resume training from the last checkpoint if needed.
Conclusion
Calculating the accuracy of a PyTorch model every epoch is an essential step in evaluating the performance of your model during training. By comparing the predicted labels with the actual labels for each batch of data, you can get a sense of how well your model is classifying the data and whether you need to make any adjustments to your model or hyperparameters.
In this blog post, we showed you how to calculate the accuracy of a PyTorch model using the torch.max
function, and how to visualize the results using matplotlib
. We hope that this guide will be helpful to you as you continue to work with PyTorch and build even better deep learning models.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.