C Algorithm to Tint a Color a Certain Percent
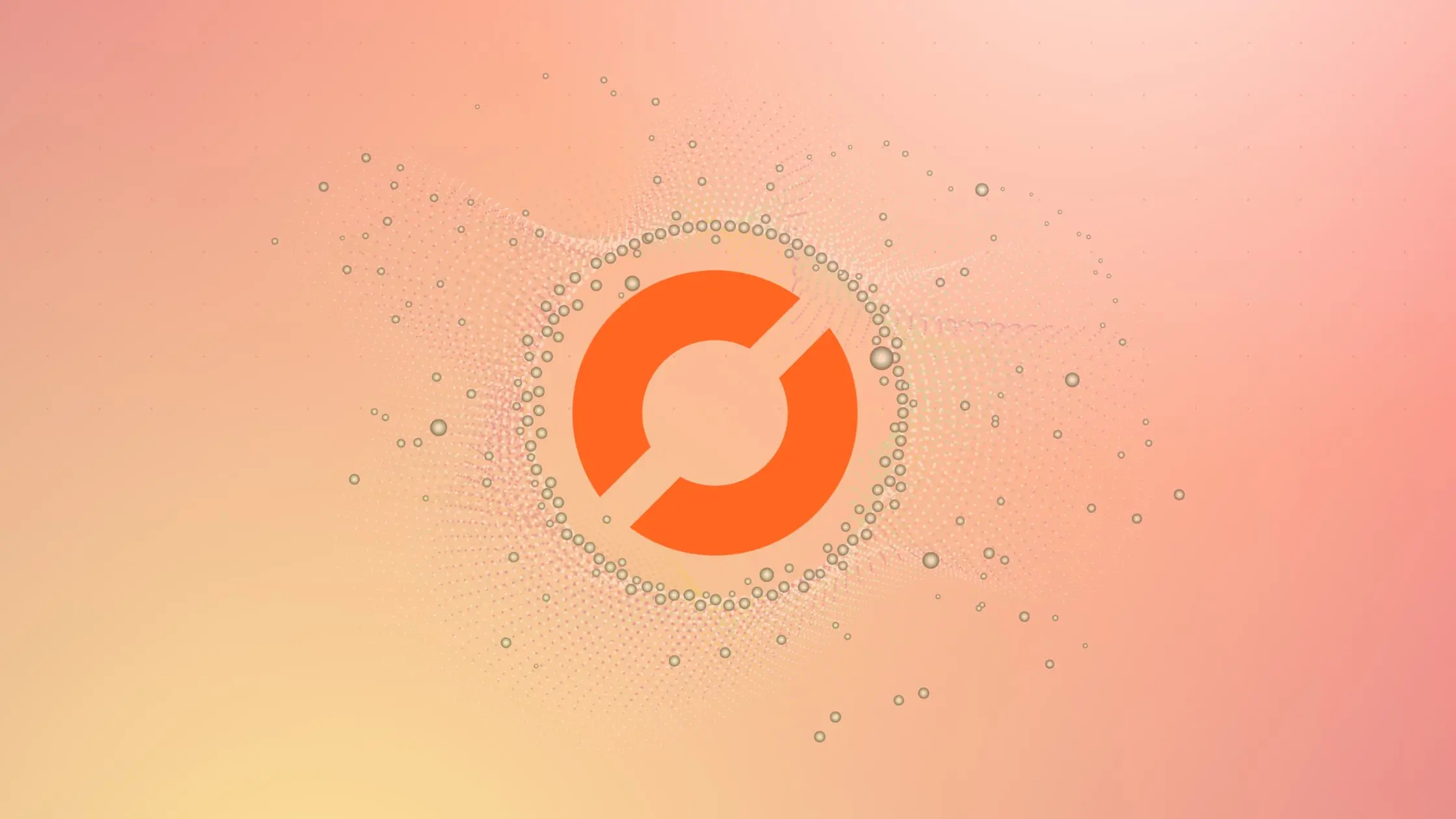
Table of Contents
- Introduction
- What is Color Tinting?
- The RGB Color Model
- The Tinting Algorithm in C for RGB Colors
- Best Practices in Color Manipulation
- Common Errors and How to Handle Them
- Conclusion
Introduction
As a data scientist or software engineer, it is crucial to have a deep understanding of algorithms and their implementations. One common task is tinting a color by a certain percentage, which can be useful in various applications such as image processing or data visualization. In this article, we will explore how to create a C# algorithm to tint a color by a specific percentage.
What is Color Tinting?
Before diving into the algorithm, let’s first understand what color tinting entails. Color tinting is the process of changing the hue or saturation of a color while maintaining its brightness or lightness. Tinting a color by a certain percentage means increasing or decreasing the color’s saturation while preserving its original hue and brightness.
The RGB Color Model
In computer graphics, colors are often represented using the RGB color model. This model defines colors based on the intensity of red (R), green (G), and blue (B) primary colors. Each color component ranges from 0 to 255, where 0 represents no intensity, and 255 represents full intensity.
To tint a color, we need to adjust its RGB values while keeping the original hue intact. A common approach is to convert the RGB color to the HSL (Hue, Saturation, Lightness) color model, modify the saturation value, and then convert it back to RGB.
The Tinting Algorithm in C for RGB Colors
#include <stdio.h>
// Tint an RGB color
void tintColorRGB(int *red, int *green, int *blue, float percent) {
// Ensure the percentage is within the valid range [0, 100]
percent = (percent < 0) ? 0 : (percent > 100) ? 100 : percent;
// Calculate the tinted color values
*red = *red + (255 - *red) * (percent / 100);
*green = *green + (255 - *green) * (percent / 100);
*blue = *blue + (255 - *blue) * (percent / 100);
}
int main() {
// Example usage for RGB color tinting
int originalRed = 100, originalGreen = 150, originalBlue = 200;
float tintPercentage = 25.0;
tintColorRGB(&originalRed, &originalGreen, &originalBlue, tintPercentage);
// Display the tinted RGB color
printf("Tinted RGB Color: (%d, %d, %d)\n", originalRed, originalGreen, originalBlue);
return 0;
}
Best Practices in Color Manipulation
- Validate input values to ensure they fall within the expected ranges.
- Use meaningful variable names for better code readability.
- Comment the code to explain complex operations and edge cases.
Common Errors and How to Handle Them
Error 1: Overflow Issues
One common issue is the overflow of color values beyond the valid range (0-255). To address this, check and limit the values during calculation.
Error 2: Incorrect Percentage Values
Ensure that the tinting percentage is between 0 and 100. Clamp the percentage value to this range to avoid unexpected results.
Error 3: Invalid Color Representations
Handle cases where the input color values are invalid, such as negative or out-of-range values. Validate inputs before applying the algorithm.
Conclusion
Tinting a color by a certain percentage is a common task in various software applications, especially in the field of data visualization and image processing. By converting the RGB color to the HSL color model, modifying the saturation, and converting it back to RGB, we can achieve the desired color tint.
In this article, we explored a C# algorithm for tinting a color by a specific percentage. By understanding the underlying formulas and implementing the code, you can now apply this technique to your own projects. Experiment with different tint percentages and explore the endless possibilities of color manipulation in your software applications.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.