Bresenham Line Algorithm: A Powerful Tool for Efficient Line Drawing
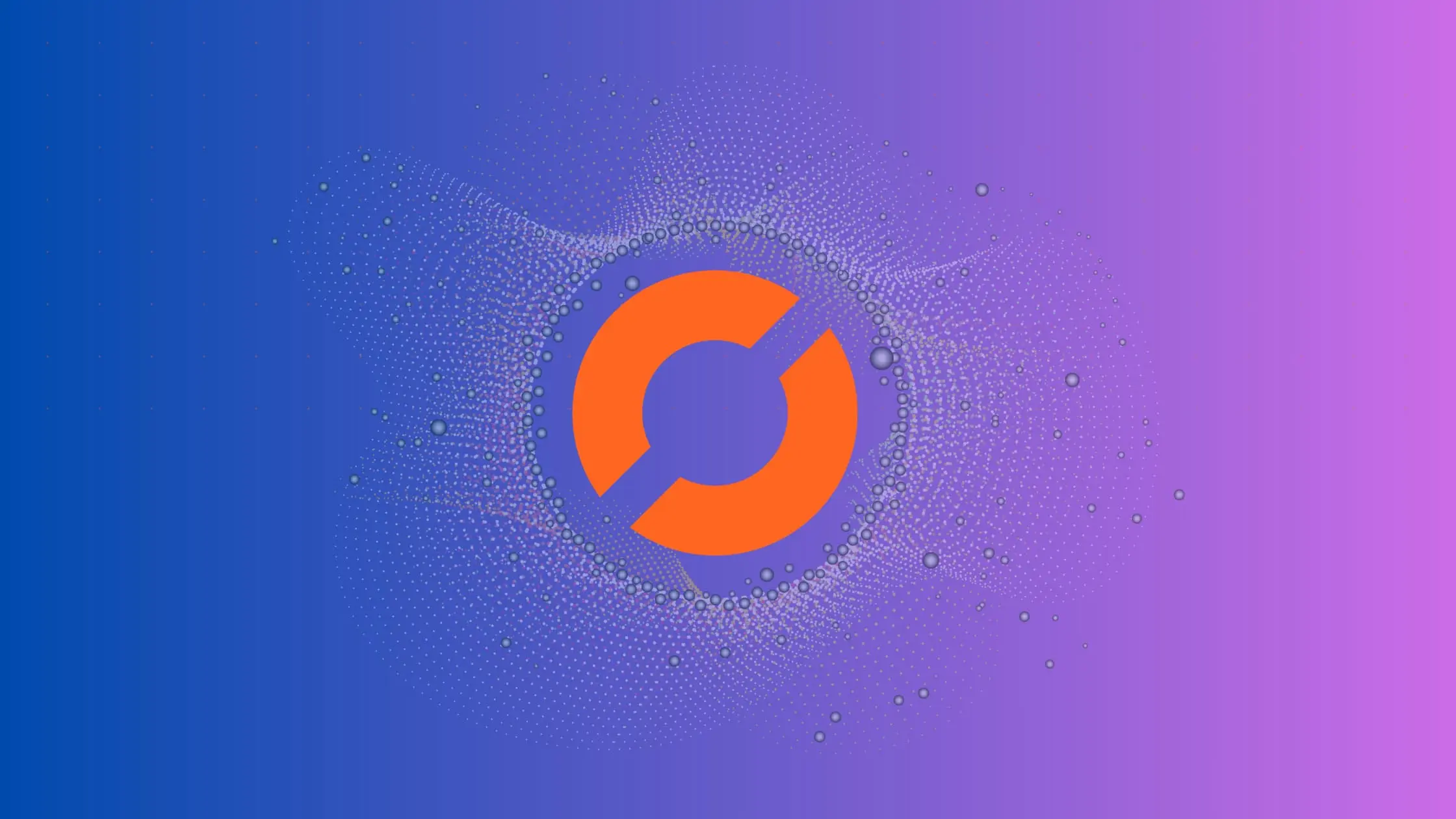
What is the Bresenham Line Algorithm?
The Bresenham Line Algorithm is an efficient method for drawing lines on a grid-based display or calculating line-related operations. It was developed by Jack E. Bresenham in 1962 and has since become a fundamental algorithm in computer graphics and image processing.
The primary advantage of the Bresenham Line Algorithm is its ability to determine the points of a line that should be plotted to achieve the most accurate and efficient line representation. Unlike other line-drawing algorithms, such as the Digital Differential Analyzer (DDA) algorithm, which uses floating-point calculations, the Bresenham algorithm operates solely on integer arithmetic, making it faster and more suitable for implementation on resource-constrained systems.
How Does the Bresenham Line Algorithm Work?
The Bresenham Line Algorithm uses the concept of integer-based error accumulation to calculate the most appropriate pixels to plot for a given line. Here’s a step-by-step breakdown of how the algorithm works:
- Accept the coordinates of the start and end points of the line: (x1, y1) and (x2, y2), respectively.
- Determine the differences between the x and y coordinates: dx = x2 - x1 and dy = y2 - y1.
- Calculate the decision parameter, also known as the error term: d = 2 * dy - dx.
- Initialize two variables: x = x1 and y = y1, which represent the current coordinates being plotted.
- Begin plotting the line by setting the initial pixel at (x, y).
- Iterate over the x-axis from x1 to x2:
- If d > 0, increment y by 1 and update d using the formula: d = d + 2 * (dy - dx).
- If d ≤ 0, update d using the formula: d = d + 2 * dy.
- Increment x by 1.
- Plot the pixel at (x, y).
- Repeat step 6 until x reaches x2.
By iteratively updating the decision parameter and selectively incrementing the y-coordinate, the Bresenham Line Algorithm efficiently determines the points to plot, resulting in a smooth and accurate line representation.
Applications of the Bresenham Line Algorithm
The Bresenham Line Algorithm finds applications in various domains, including computer graphics, image processing, and computational geometry. Here are a few use cases where this algorithm is particularly useful:
Line Drawing
The primary application of the Bresenham Line Algorithm is in drawing lines on a digital display or canvas. Its efficiency makes it suitable for real-time rendering in applications such as computer-aided design (CAD), video games, and computer simulations.
Rasterization
In computer graphics, rasterization is the process of converting vector graphics into raster images or bitmaps. The Bresenham Line Algorithm plays a crucial role in rasterization by determining the pixels to plot for each line segment, resulting in a smooth and accurate image representation.
Line Clipping
Line clipping is the process of determining which portions of a line lie within a specific region of interest. The Bresenham Line Algorithm can be extended to handle line clipping efficiently, making it an essential component of algorithms like the Cohen-Sutherland line clipping algorithm.
Implementing the Bresenham Line Algorithm
To implement the Bresenham Line Algorithm, you can use any programming language of your choice. Here’s a Python implementation to get you started:
def bresenham_line(x1, y1, x2, y2):
dx = abs(x2 - x1)
dy = abs(y2 - y1)
slope = dy > dx
if slope:
x1, y1 = y1, x1
x2, y2 = y2, x2
if x1 > x2:
x1, x2 = x2, x1
y1, y2 = y2, y1
dx = abs(x2 - x1)
dy = abs(y2 - y1)
error = dx // 2
y = y1
ystep = 1 if y1 < y2 else -1
points = []
for x in range(x1, x2 + 1):
coord = (y, x) if slope else (x, y)
points.append(coord)
error -= dy
if error < 0:
y += ystep
error += dx
return points
Feel free to adapt this code to your specific requirements or translate it into your preferred programming language.
Conclusion
The Bresenham Line Algorithm is a powerful tool for efficient line drawing and line-related operations. Its ability to determine the most accurate pixels to plot using integer arithmetic makes it a popular choice in computer graphics, image processing, and computational geometry.
By understanding the inner workings of the Bresenham Line Algorithm and its applications, you can leverage its efficiency to optimize line drawing in your projects. So go ahead, implement the Bresenham Line Algorithm, and explore the possibilities it offers for smooth and accurate line representations.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.