Axios Post Request to Send Form Data
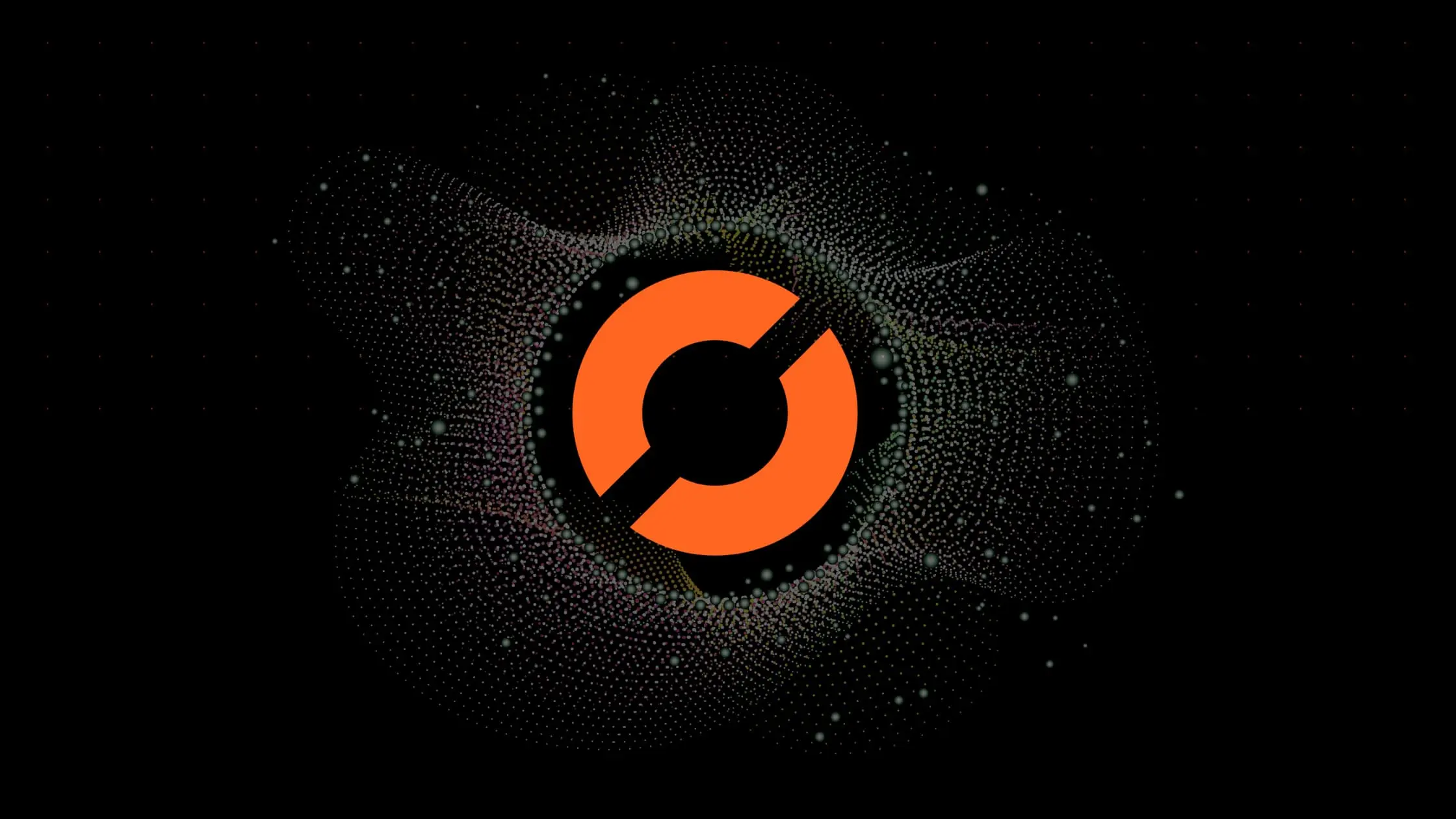
In this blog post, we will explore how to use Axios to make a post request to send form data.
What is Axios?
Axios is a promise-based HTTP client for JavaScript that can be used both in the browser and Node.js. It is a lightweight and easy-to-use library that provides an easy-to-use interface for making HTTP requests. Axios is built on top of the XMLHttpRequest API and the Fetch API.
Making a Post Request with Axios
To make a post request with Axios, we first need to install it. We can do this using npm or yarn.
npm install axios
Once we have installed Axios, we can use it to make a post request to send form data. Here is an example:
const axios = require('axios');
const querystring = require('querystring');
const formData = {
name: 'John Doe',
email: 'johndoe@example.com'
};
axios.post('/submit-form', querystring.stringify(formData), {
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
}
})
.then(function (response) {
console.log(response.data);
})
.catch(function (error) {
console.log(error);
});
In this example, we create a JavaScript object (formData) to represent the form fields and their values. We then use axios.post to send an HTTP POST request to the ‘/submit-form’ endpoint. The first argument to the post method is the URL we want to send the data to. The second argument, querystring.stringify(formData), serializes the formData object into a URL-encoded string. The third argument is an options object where we set the Content-Type header to indicate that we are sending form data.
Sending Data in JSON Format
In addition to sending form data, we can also send data in JSON format using Axios. Here is an example:
const axios = require('axios');
const data = {
name: 'John Doe',
email: 'johndoe@example.com'
};
axios.post('/submit-form', data)
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
});
In this example, we create a JavaScript object containing the data we want to send. We then use the Axios post method to send the data to the server. The post method automatically serializes the data to JSON format before sending it.
Handling Errors
When making HTTP requests, errors can occur. Axios provides a convenient way to handle errors using the catch method we used in the above two examples:
axios.post('/submit-form', formData)
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
});
If an error occurs, the catch method is called and we can log the error to the console or handle it in some other way.
Conclusion
In this blog post, we have explored how to use Axios to make a post request to send form data. We have seen how to send data in both form data and JSON format, and how to handle errors. Axios is a powerful and easy-to-use library for making HTTP requests in JavaScript, and it can be used both in the browser and Node.js.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.