Appending a List as a Row to a Pandas DataFrame
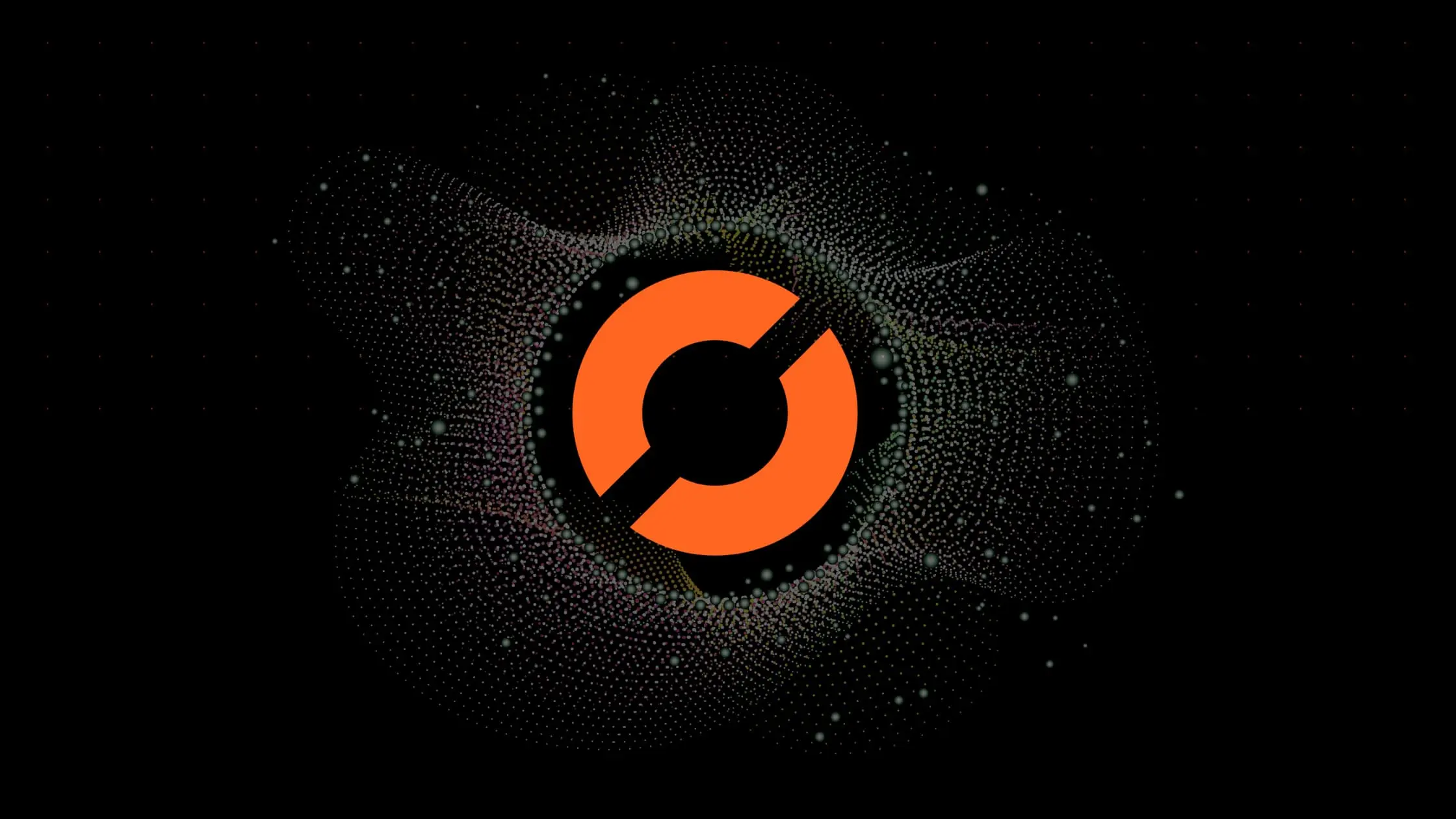
Introduction
Appending a list to a DataFrame is a common operation in data manipulation. However, it can be tricky when you want to add a new row with a specific index. This guide will help you understand how to do this efficiently using Pandas.
- Note:
append
method (deprecated as of v1. 4.0) has been completely removed as of pandas 2.0
Prerequisites
Before we start, make sure you have the following:
- Python 3.6 or later installed
- Pandas library installed
- Basic understanding of Python and Pandas
Step 1: Importing the Pandas Library
First, we need to import the Pandas library. If you haven’t installed it yet, you can do so using pip:
pip install pandas
Then, import the library in your Python script:
import pandas as pd
Step 2: Creating a DataFrame
Let’s create a simple DataFrame for demonstration:
df = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9]
})
Step 3: Appending a List as a a New Row
To append a list as a new row, we use the loc[]
function. Here’s how:
list = [10, 11, 12]
df.loc[len(df)] = list
The new dataframe would look like this:
A B C
0 1 4 7
1 2 5 8
2 3 6 9
3 12 13 14
Appending a List with a Specific Index
If you want to append a list at a specific index, you would use the iloc[]
method. Here is how it works:
import pandas as pd
df = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9]
})
list2 = [13,14,15]
df.iloc[0] = list2
print(df)
The output would look like this:
A B C
0 13 14 15
1 2 5 8
2 3 6 9
Note: It is used for location-based indexing so it works for only the existing index and replaces the row element.
Conclusion
Appending a list as a new row in a DataFrame with a specific index is a common operation in data manipulation. This guide has shown you how to do it efficiently using Pandas. However, appending rows to a df is time-consuming and should be avoided. Sometimes it is necessary but a good practice is to build your table outside of pandas then create a df to process the data which is much faster than adding rows.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.