Adding Headers to a DataFrame in Pandas: A Guide
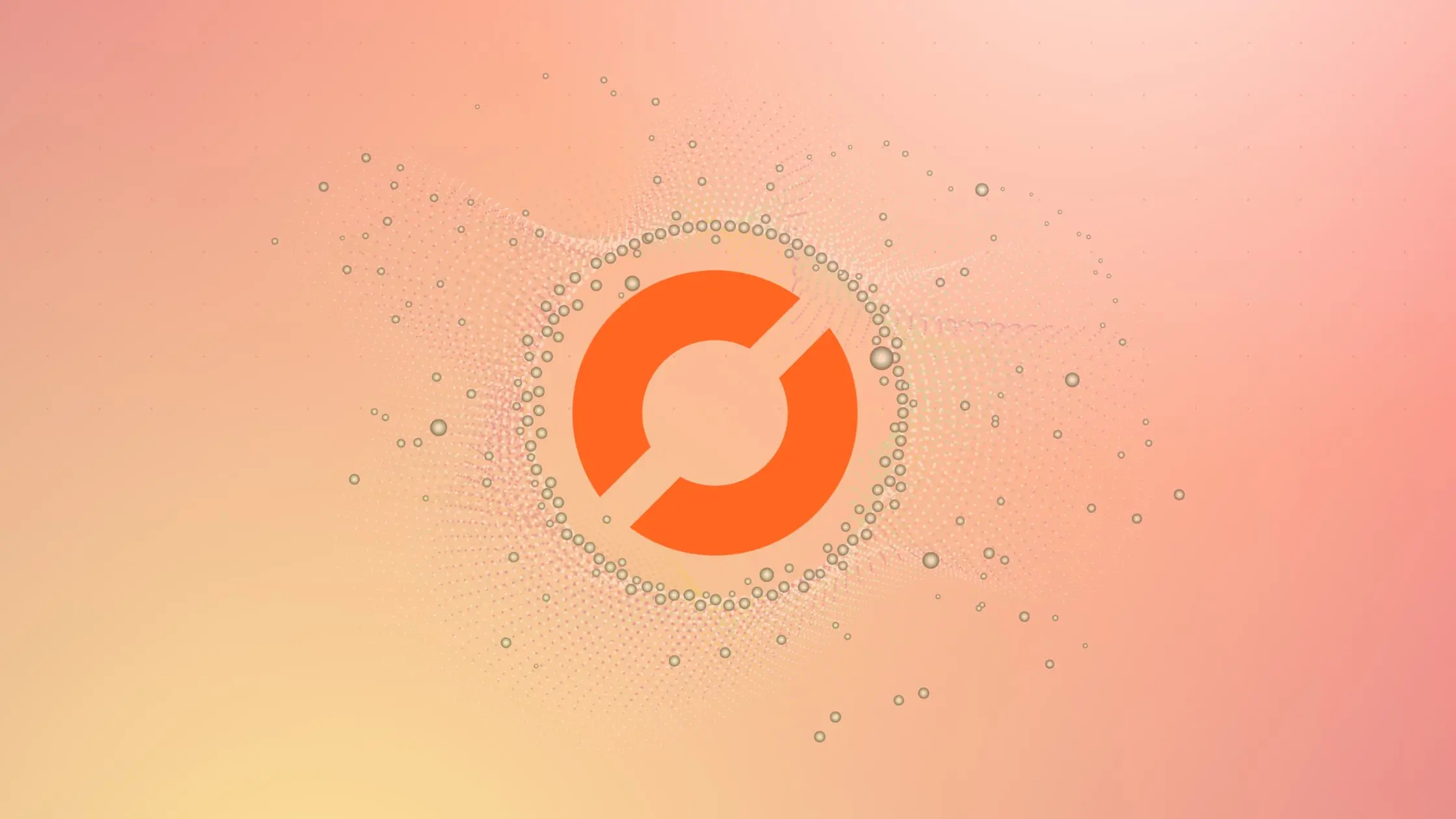
Adding Headers to a DataFrame in Pandas: A Guide
Pandas is a powerful data manipulation library in Python that provides data structures and functions needed for manipulating structured data. One common task that data scientists often encounter is adding headers to a DataFrame. This blog post will guide you through the process of adding headers to a DataFrame in Pandas.
What is a DataFrame?
A DataFrame is a two-dimensional labeled data structure with columns of potentially different types. It is similar to a spreadsheet or SQL table, or a dictionary of Series objects. DataFrames are generally the most commonly used pandas object.
Why Add Headers to a DataFrame?
Headers provide a name to each column in the DataFrame, making it easier to reference specific columns. They are essential for understanding the data by providing context. If your DataFrame doesn’t have headers, or you want to change the existing ones, Pandas provides several methods to add or modify them.
How to Add Headers to a DataFrame in Pandas
Let’s dive into the process of adding headers to a DataFrame. We’ll start by importing the necessary libraries and creating a simple DataFrame without headers.
import pandas as pd
data = [[1, 'John', 'Smith'], [2, 'Anna', 'Brown'], [3, 'Tom', 'Davis']]
df = pd.DataFrame(data)
print(df)
This will output:
0 1 2
0 1 John Smith
1 2 Anna Brown
2 3 Tom Davis
As you can see, our DataFrame doesn’t have headers. Let’s add them.
Using the columns attribute
The simplest way to add headers to a DataFrame is by assigning a list of column names to the columns
attribute of the DataFrame.
df.columns = ['ID', 'First Name', 'Last Name']
print(df)
This will output:
ID First Name Last Name
0 1 John Smith
1 2 Anna Brown
2 3 Tom Davis
Using the rename method
Another way to add headers to a DataFrame is by using the rename
method. This method is particularly useful when you want to change only a few column names.
df = df.rename(columns={0: 'ID', 1: 'First Name', 2: 'Last Name'})
print(df)
This will output the same result as the previous method.
Conclusion
Adding headers to a DataFrame in Pandas is a simple yet essential task in data manipulation. It provides context to your data and makes it easier to reference specific columns. Whether you’re using the columns
attribute or the rename
method, Pandas provides flexible options to add or modify headers in a DataFrame.
Remember, the key to mastering data manipulation in Pandas is practice. So, keep experimenting with different methods and techniques. Happy coding!
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.