A List of Pandas readcsv Encoding Options
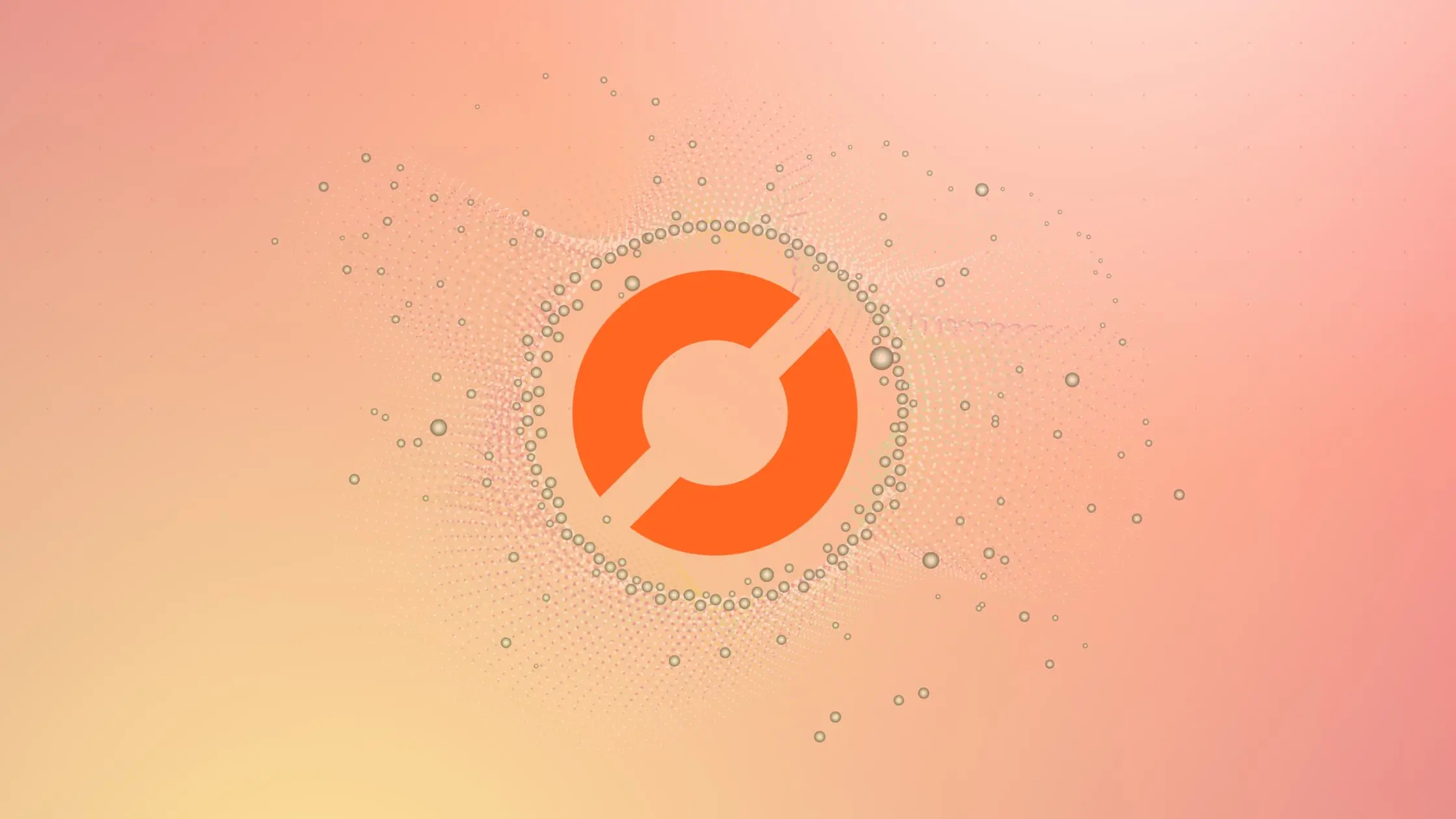
As a data scientist or software engineer, you know that handling data is an essential part of your job. One of the most common tasks in data handling is reading data from various sources, including CSV files.
Pandas is a powerful library for data manipulation in Python, and it provides a read_csv
function that makes reading CSV files a breeze. However, one common issue that data scientists and software engineers face when reading CSV files is dealing with different encodings.
In this article, we’ll provide a list of encoding options for the read_csv
function in Pandas. We’ll discuss what encoding is, why it matters, and provide examples of how to use different encoding options in Pandas.
What is Encoding?
Encoding is the process of converting characters from one representation to another. When we work with text data, we need to represent each character using a unique code. There are numerous encoding formats, including ASCII, UTF-8, and ISO-8859-1.
The encoding format determines how the text is represented in a computer’s memory. Different encoding formats use different numbers of bits to represent each character. For instance, ASCII represents each character using 7 bits, while UTF-8 uses variable-length encoding, with each character represented by one to four bytes.
When reading data from a CSV file, you need to specify the encoding format used in the file. If you don’t specify the encoding format, Pandas will try to infer it automatically, but this can lead to errors and incorrect data.
Assume that we have the following DataFrame:
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie', 'Dölek', 'Élise'],
'Age': [25, 30, 35, 40, 45]}
df = pd.DataFrame(data)
# Save the DataFrame to a CSV file with 'utf-8' encoding
df.to_csv('example_utf8.csv', index=False, encoding='utf-8')
In this example, the Name
column contains non-ASCII characters (e.g., Dölek
and Élise
). When you save this DataFrame to a CSV file using utf-8
encoding and later read it using read_csv, you should specify utf-8
as the encoding to correctly handle these special characters:
List of Encoding Options in Pandas read_csv
Here’s a list of encoding options that you can use with the read_csv
function in Pandas:
df = pd.read_csv('example_utf8.csv', encoding='utf-8')
print(df)
Output:
Name Age
0 Alice 25
1 Bob 30
2 Charlie 35
3 Dölek 40
4 Élise 45
If you do not specify or you use another encoding method, the returning DataFrame will be affected:
df = pd.read_csv('example_utf8.csv', encoding='utf-8')
print(df)
Output:
Name Age
0 Alice 25
1 Bob 30
2 Charlie 35
3 Dölek 40
4 Ãlise 45
Let’s explore several types of encoding method which are supported by Pandas.
1. UTF-8
UTF-8 is the most widely used encoding format for text data. It supports all characters in the Unicode standard and is compatible with ASCII. To specify UTF-8 encoding in Pandas, use the encoding='utf-8'
parameter.
import pandas as pd
df = pd.read_csv('file.csv', encoding='utf-8')
2. ISO-8859-1
ISO-8859-1, also known as Latin-1, is another popular encoding format for text data. It supports most Western European languages and is compatible with ASCII. To specify ISO-8859-1 encoding in Pandas, use the encoding='iso-8859-1'
parameter.
import pandas as pd
df = pd.read_csv('file.csv', encoding='iso-8859-1')
3. Windows-1252
Windows-1252 is an extension of ISO-8859-1 and is the default encoding format in Windows. To specify Windows-1252 encoding in Pandas, use the encoding='windows-1252'
parameter.
import pandas as pd
df = pd.read_csv('file.csv', encoding='windows-1252')
4. Latin-2
Latin-2, also known as ISO-8859-2, is an encoding format for Central and Eastern European languages. To specify Latin-2 encoding in Pandas, use the encoding='iso-8859-2'
parameter.
import pandas as pd
df = pd.read_csv('file.csv', encoding='iso-8859-2')
5. UTF-16LE and UTF-16BE
UTF-16LE and UTF-16BE are encoding formats for Unicode text data that use 16 bits to represent each character. UTF-16LE is little-endian, which means that the least significant byte comes first, while UTF-16BE is big-endian, which means that the most significant byte comes first. To specify UTF-16LE or UTF-16BE encoding in Pandas, use the encoding='utf-16le'
or encoding='utf-16be'
parameter, respectively.
import pandas as pd
df = pd.read_csv('file.csv', encoding='utf-16le')
import pandas as pd
df = pd.read_csv('file.csv', encoding='utf-16be')
6. ASCII
ASCII is a 7-bit encoding format that represents only the basic English alphabet, digits, and punctuation marks. To specify ASCII encoding in Pandas, use the encoding='ascii'
parameter.
import pandas as pd
df = pd.read_csv('file.csv', encoding='ascii')
7. CP1252
CP1252 is a Microsoft-specific extension of ISO-8859-1. It supports most Western European languages and is the default encoding format in many Microsoft applications. To specify CP1252 encoding in Pandas, use the encoding='cp1252'
parameter.
import pandas as pd
df = pd.read_csv('file.csv', encoding='cp1252')
8. Other Encodings
If your CSV file uses a different encoding format, you can specify it using the appropriate encoding name. You can find a list of encoding names supported by Python in the Python documentation. To specify a custom encoding format in Pandas, use the encoding='
import pandas as pd
df = pd.read_csv('file.csv', encoding='<encoding-name>')
Conclusion
In conclusion, reading CSV files is a crucial part of data handling in data science and software engineering. Pandas provides a powerful read_csv
function that makes reading CSV files easy. However, different encoding formats can cause problems when reading CSV files.
In this article, we’ve provided a list of encoding options that you can use with the read_csv
function in Pandas. We’ve discussed what encoding is, why it matters, and provided examples of how to use different encoding options in Pandas.
By understanding encoding and using the appropriate encoding options in Pandas, you can avoid errors and ensure that your data is correctly represented.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.