Take Your MLOps
to Any Cloud
Get the power of SageMaker but with flexibility,
ease, and affordability
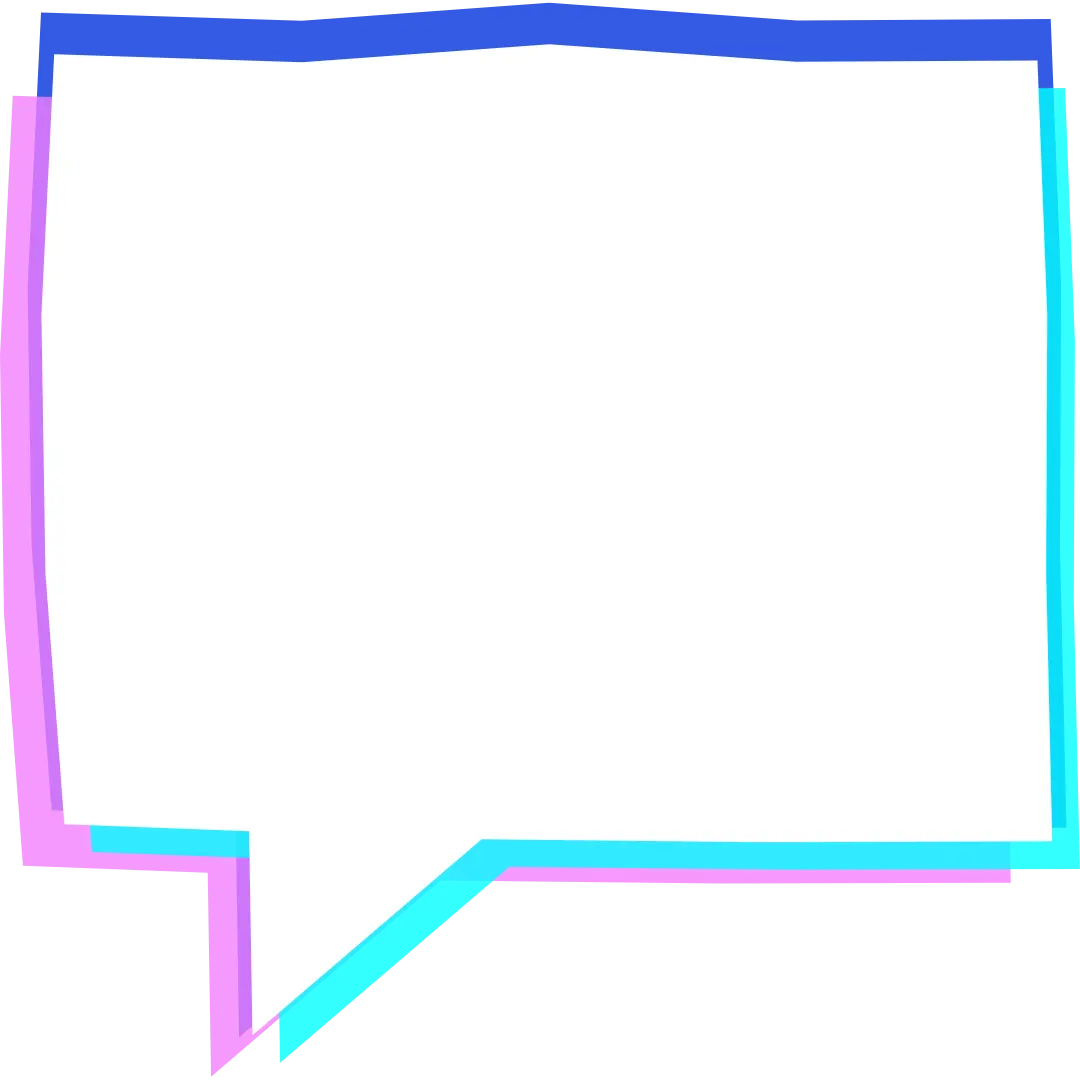
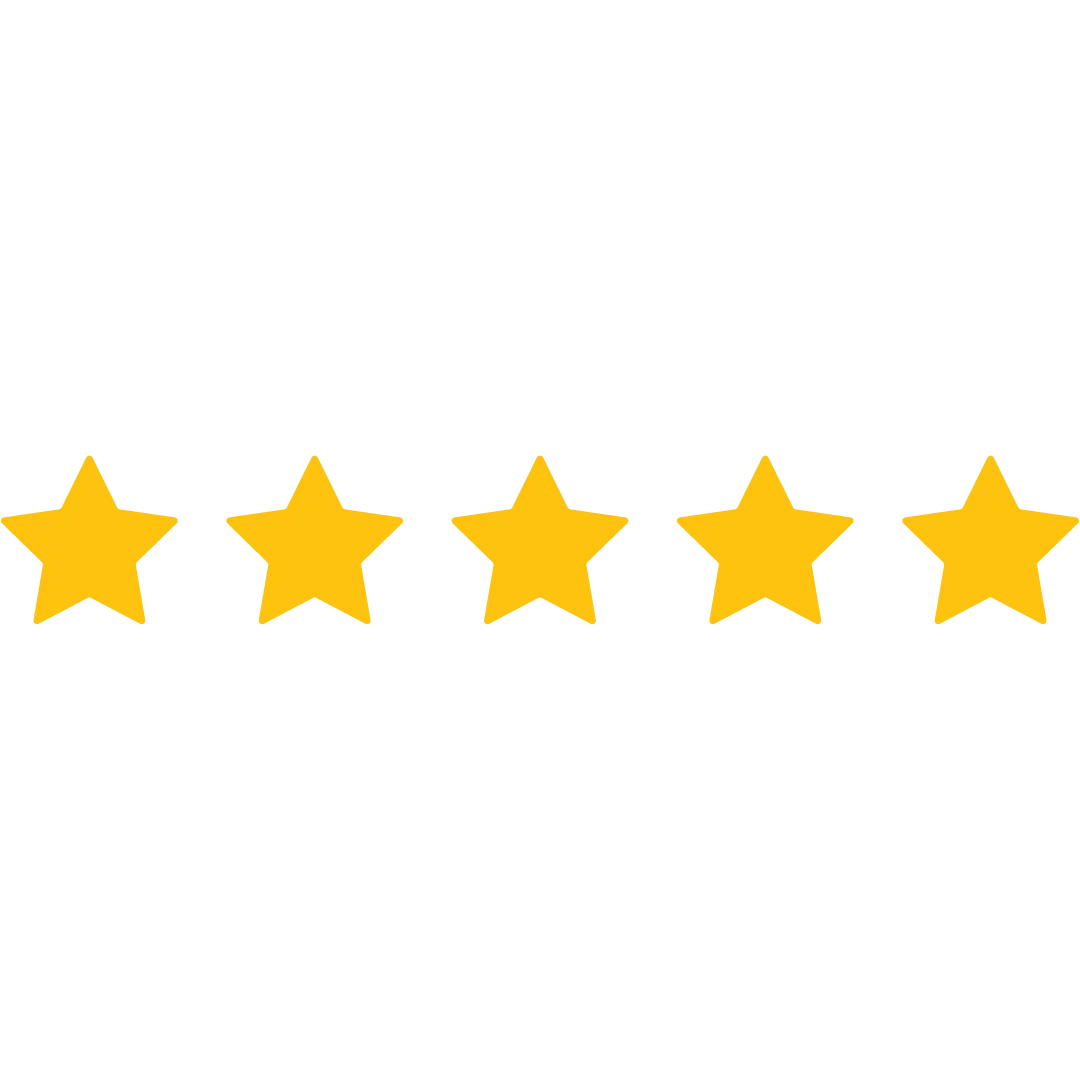
"Best platform to experiment with LLM models. Extremely easy to launch a new server instance or just run your code on a Jupyter notebook and completely flexible CPU/GPU usage!"
| Prashanth, Data Scientist
Powering 100,000+ data science users and teams

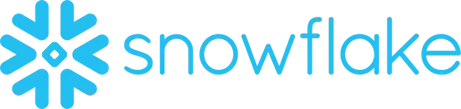
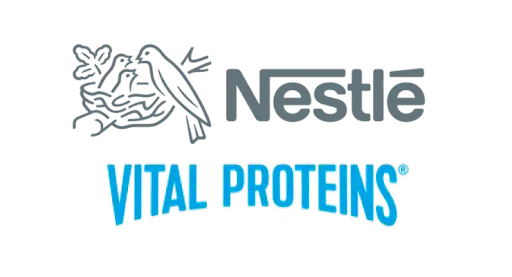
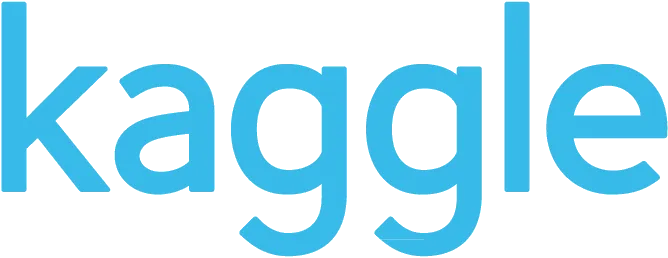
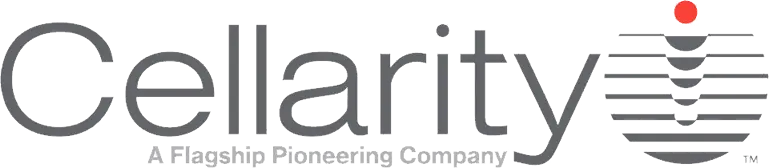
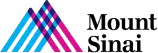
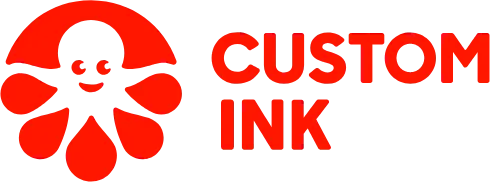
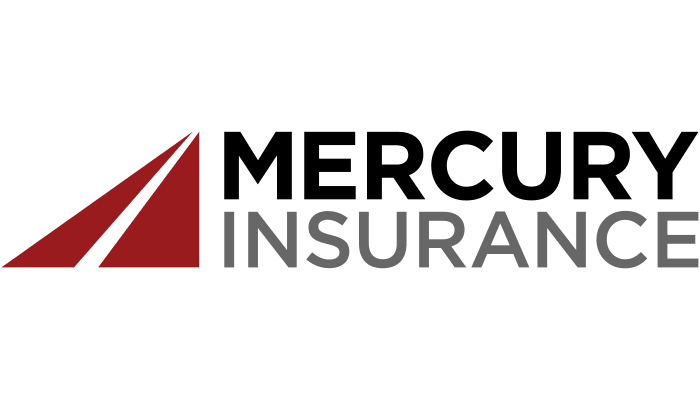
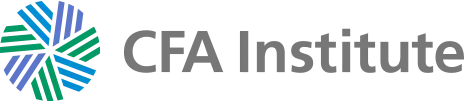

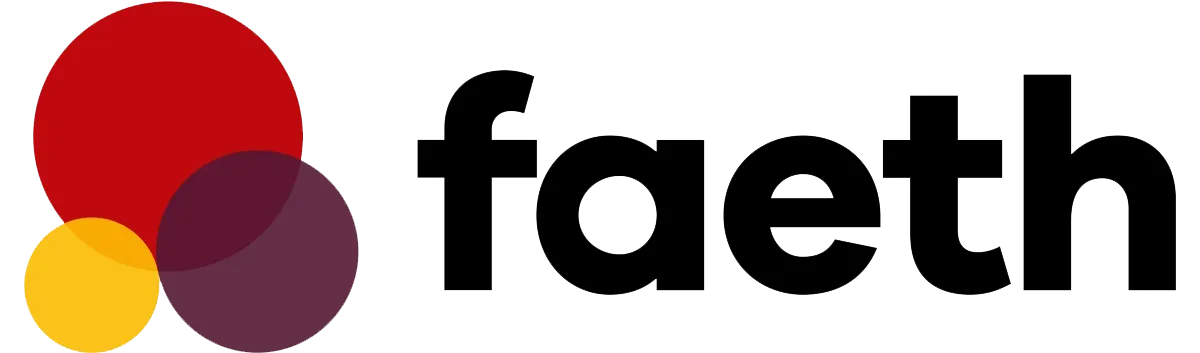
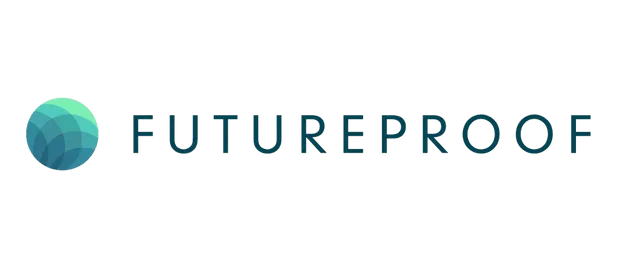

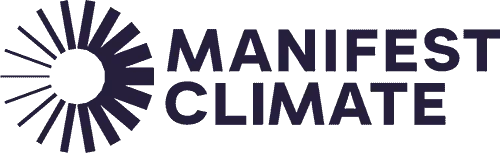
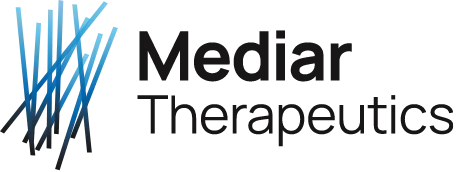
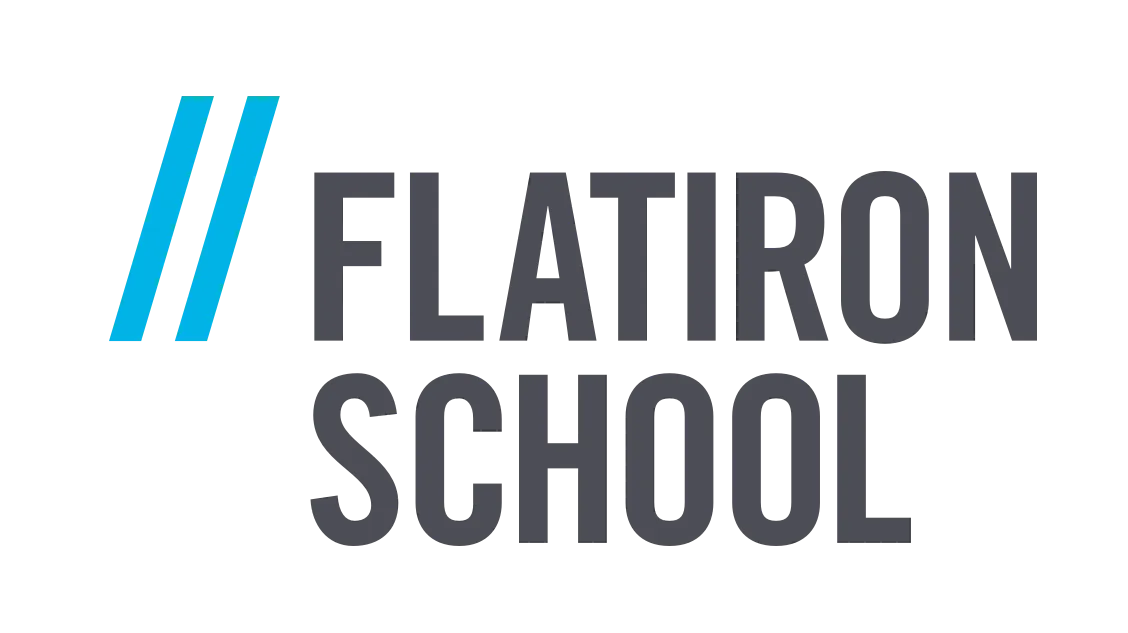
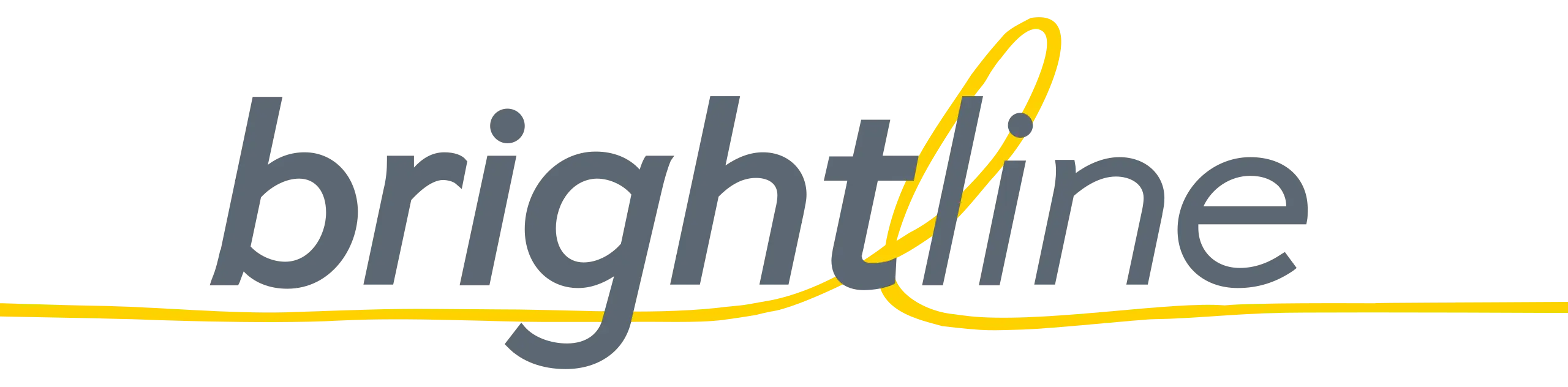
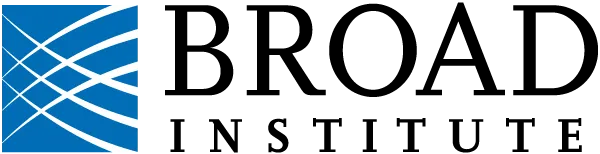
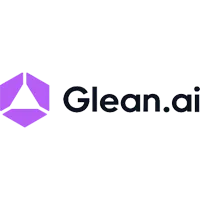
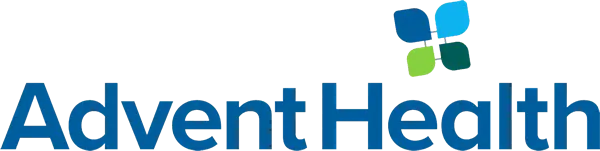

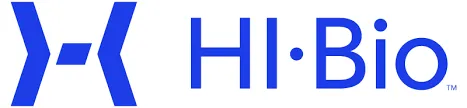

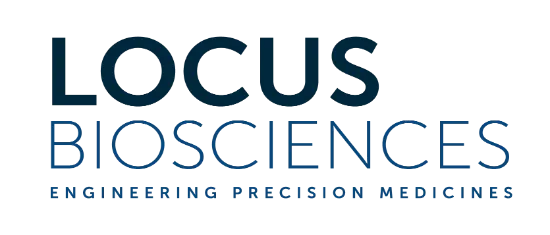
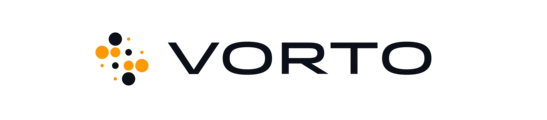
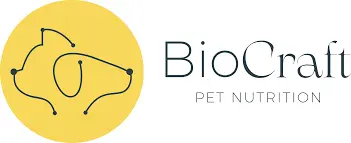
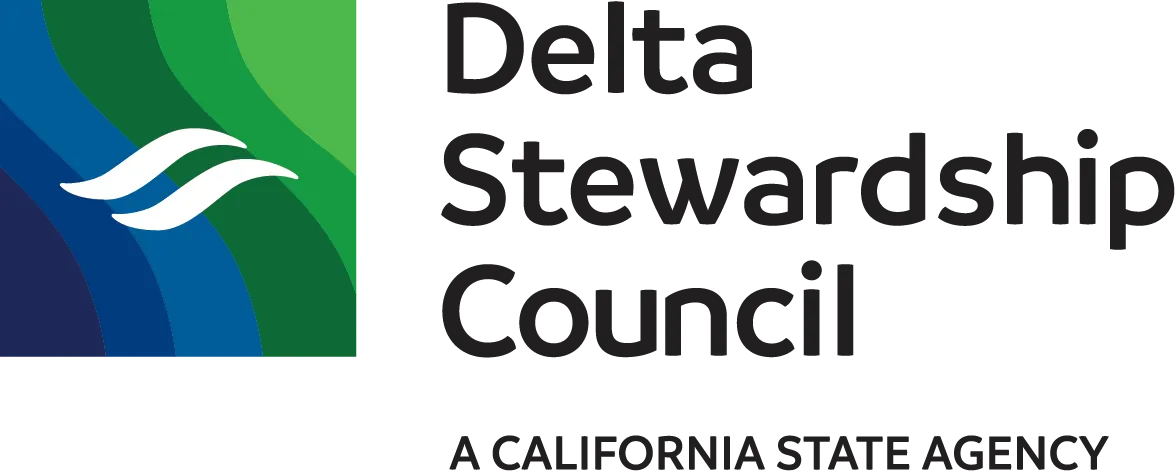
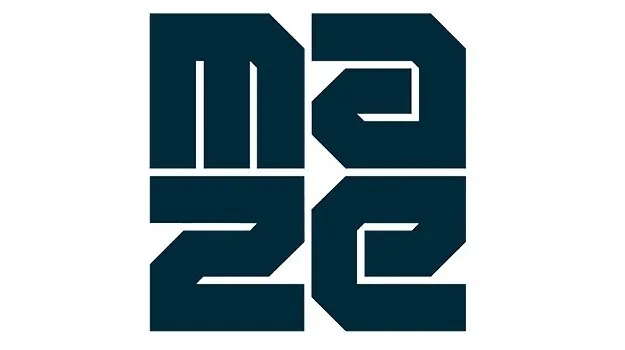
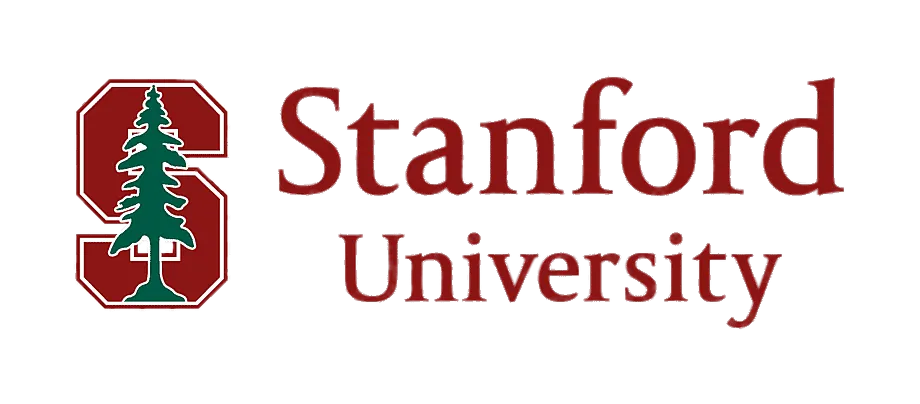
Manage all your work in one place
Flexibility
Everything that runs in Saturn Cloud is just a docker container with code from your Git repositories. If it can be installed in a Docker container, it can run in Saturn Cloud.
Scalability
Run on up to 4TB of RAM and 8 GPU machines. Or scale out with parallel processing jobs, Dask and Ray clusters.
Compatibility
Compatible with every ML framework(PyTorch, Tensorflow, Scikit-Learn, XGBoost, lightGBM, RAPIDS) and every visualization stack (streamlit, matplotlib, plotly, dash, bokeh, panel)
Controls and Governance
Control the types of machines and the amount of spend your team members can consume, so there are no surprises at the end of the month.
Saturn Cloud is available on
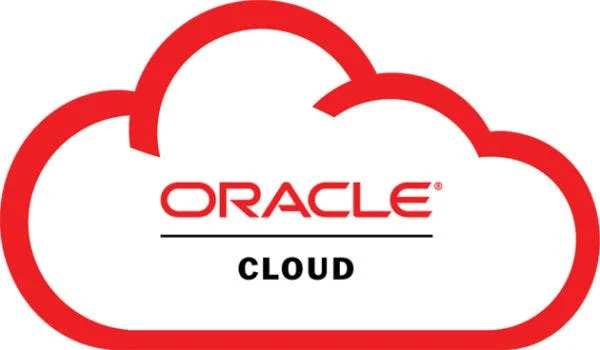
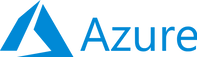
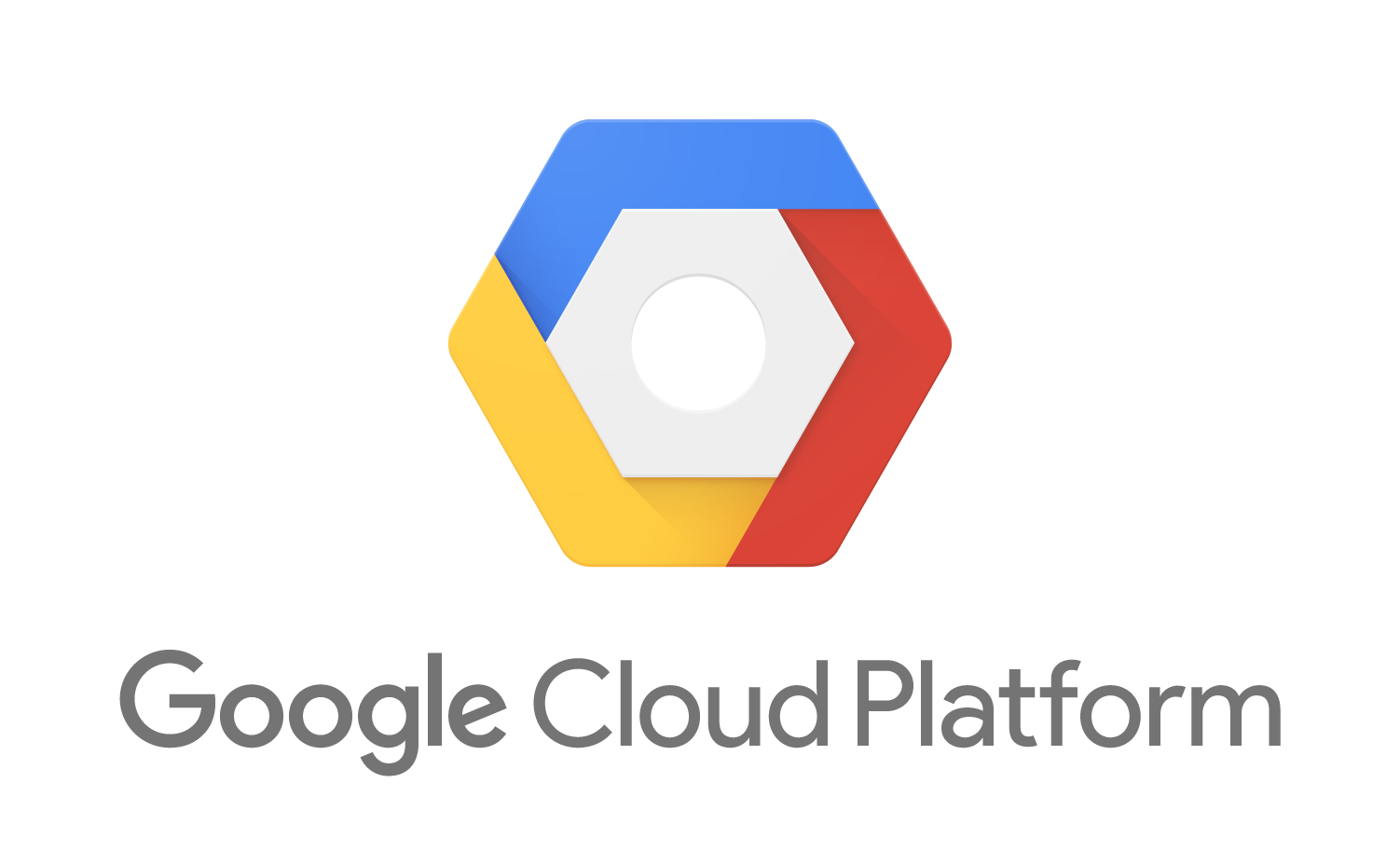
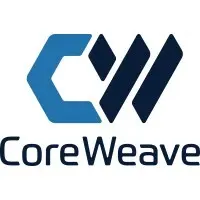
Tech specs
What's Included
- ✓Jupyter and R development environments
- ✓Support for desktop IDEs including PyCharm and VSCode
- ✓Training, fine-tuning and data processing jobs
- ✓Job Scheduling
- ✓Model deployment including endpoints for LLMs
- ✓Managed Dashboards including Streamlit, Plotly, Bokeh and Panel
- ✓Fine grained access control including IAM role support
- ✓Administrative tools, cost controls, and quotas
- ✓Installation within your cloud account and VPN
- ✓Support for restricted internet access and transparent proxies
Available Integrations
- ✓Model versioning
- ✓Model monitoring
- ✓Experiment tracking
- ✓Vector databases
- ✓Data storage and data warehouses
- ✓Enterprise identity providers and SSO
- ✓Connections to on-premise infrastructure
- ✓Networked storage
- ✓Intrusion detection and response platforms
Teams love Saturn Cloud
Don't take our word for it. See how Saturn Cloud helps teams get things done efficiently.
- Saturn makes it easy for our analysts to spin up Jupyter servers of any type on demand. The flexibility in being able to define custom docker images, startup scripts, etc. is phenomenal. I love the integration with Prefect Cloud, as it lets us seamlessly run our data ingestion pipeline using the same code and in the same environment as our analysts do their work.
- Saturn Cloud makes my work so much easier. When I sit down at the beginning of the day, I just want my environment to work. I want my favorite packages installed and available on demand. I want it to be easy to scale my workspace and have it shut down automatically when I'm done. Saturn Cloud solves all of that. Their customer service is also top-notch.
- We had a portfolio backtest taking nearly 20 minutes to run before the team at Saturn Cloud took a look at it. Between advising us on our code and dispersing the work across parallel processing via Dask, this backtest was completed at a rate several hundred times faster, taking only a few seconds to execute.
- Taking runtime down from 60 days to 11 hours is such an incredible improvement. We are able to fit in many more iterations on our models. This has a significant positive impact on the effectiveness of our product, which takes many iterations to perform at the standard necessary for our customers.
Trusted by leading ML businesses
How Senseye Achieved 120x
Faster Machine Learning
Senseye uses Saturn Cloud to train machine learning models on GPUs at a massive scale.
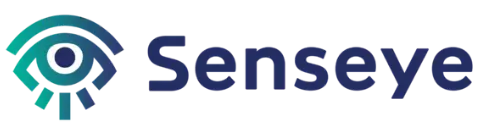
Team Size
29
Technology
Healthcare
Run Saturn Cloud anywhere, anyway you want
Run Saturn Cloud on the cloud or on-premises. Saturn Cloud is available for you to self-manage anywhere or as a fully-managed cloud service.
Featured partners
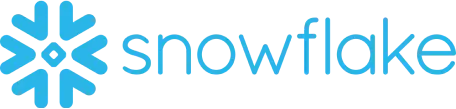
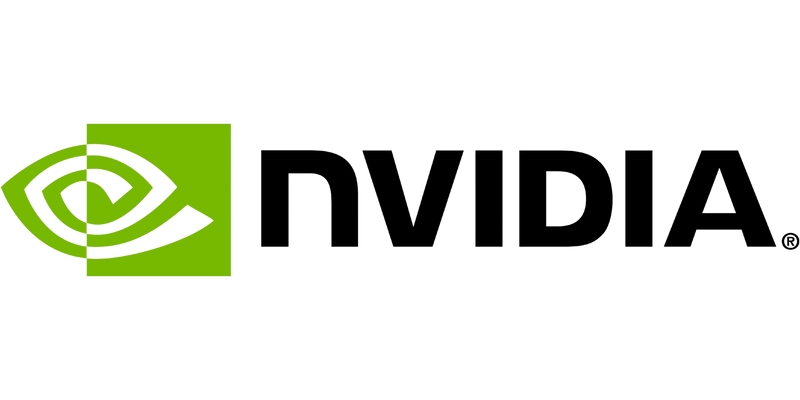
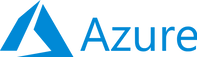
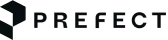
Awards
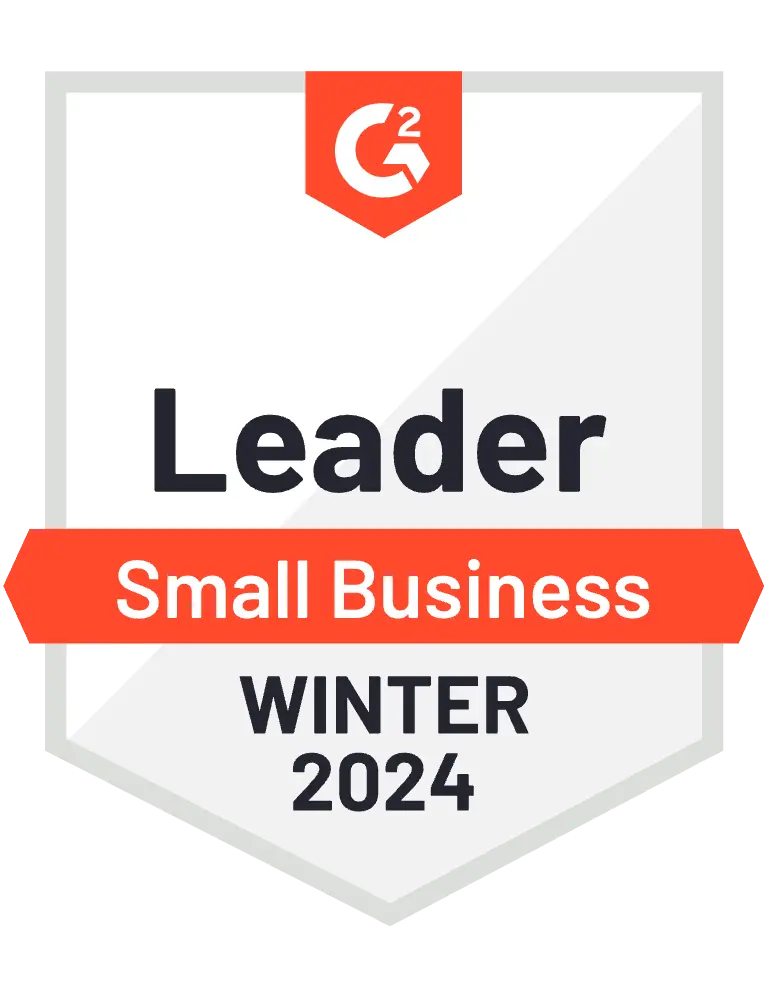
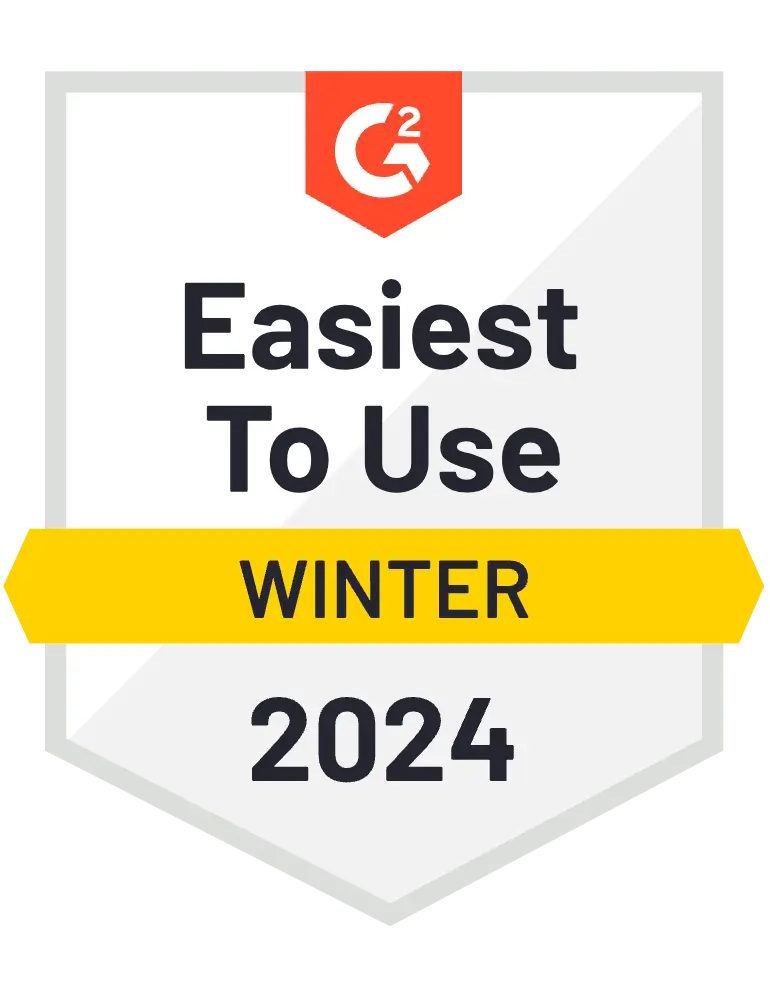
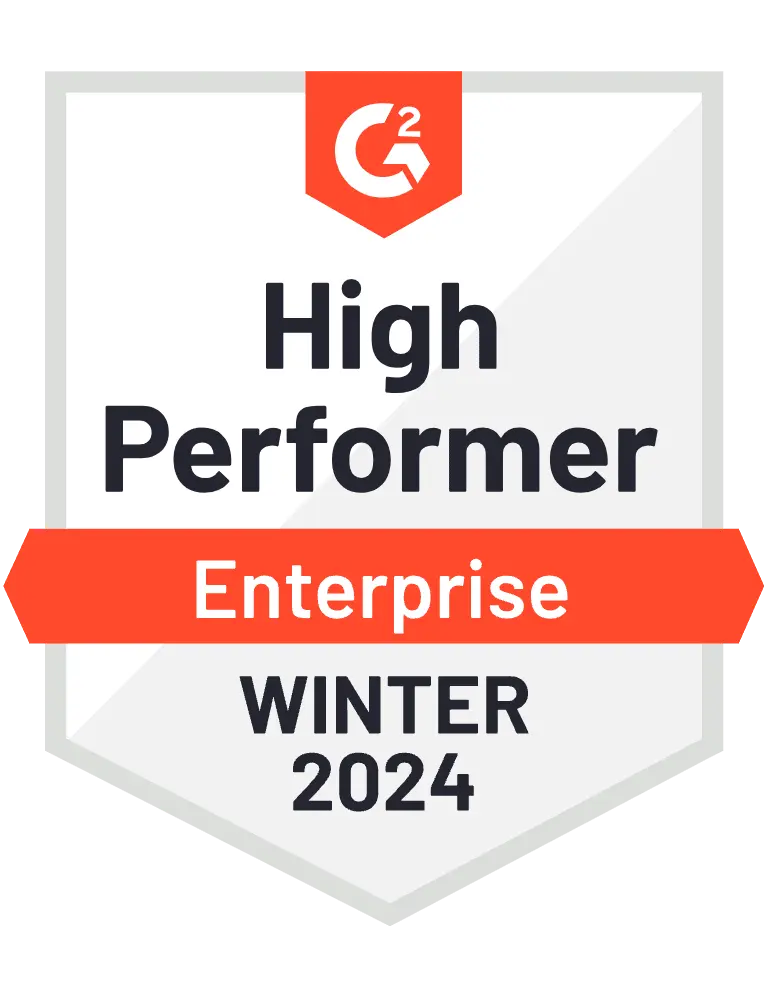
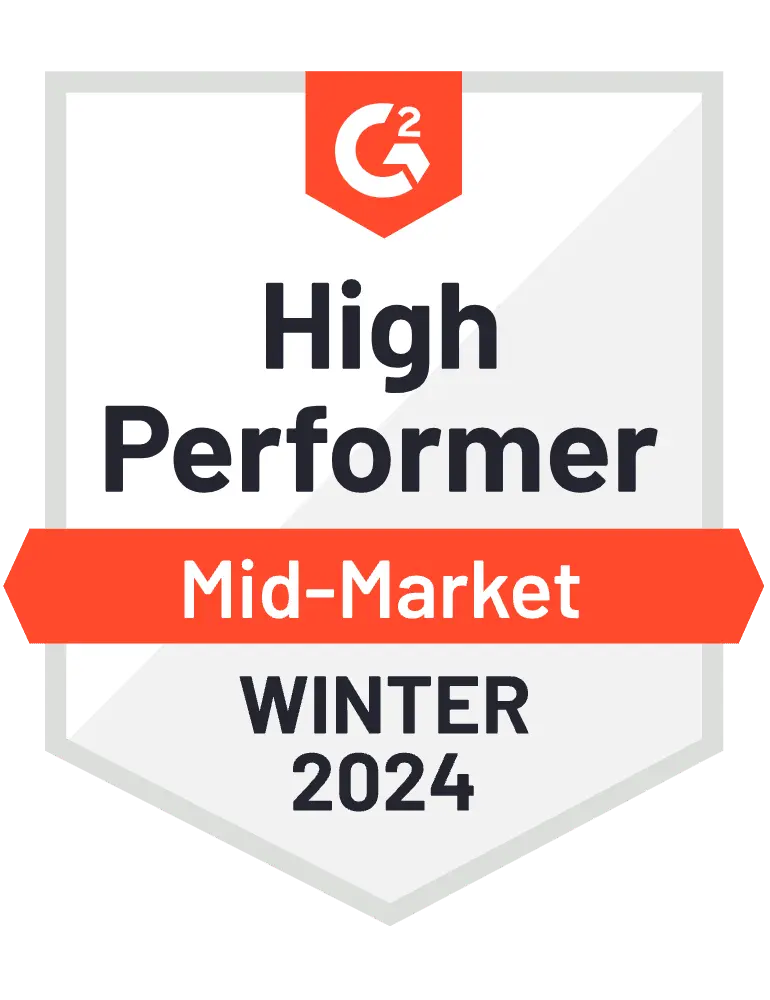
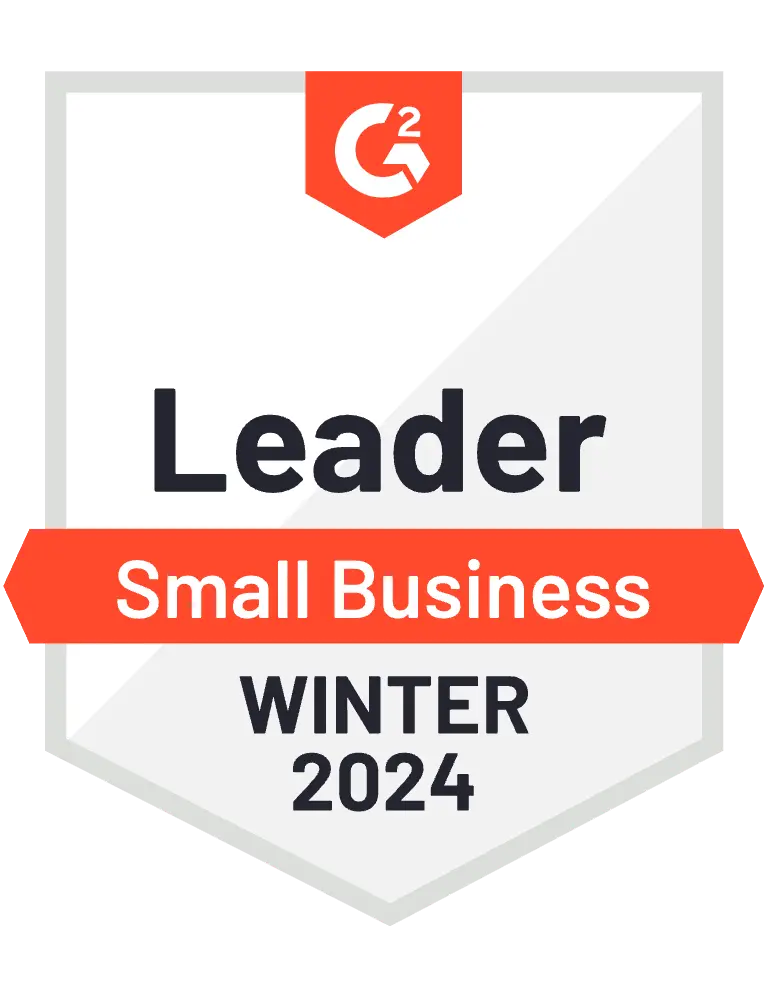
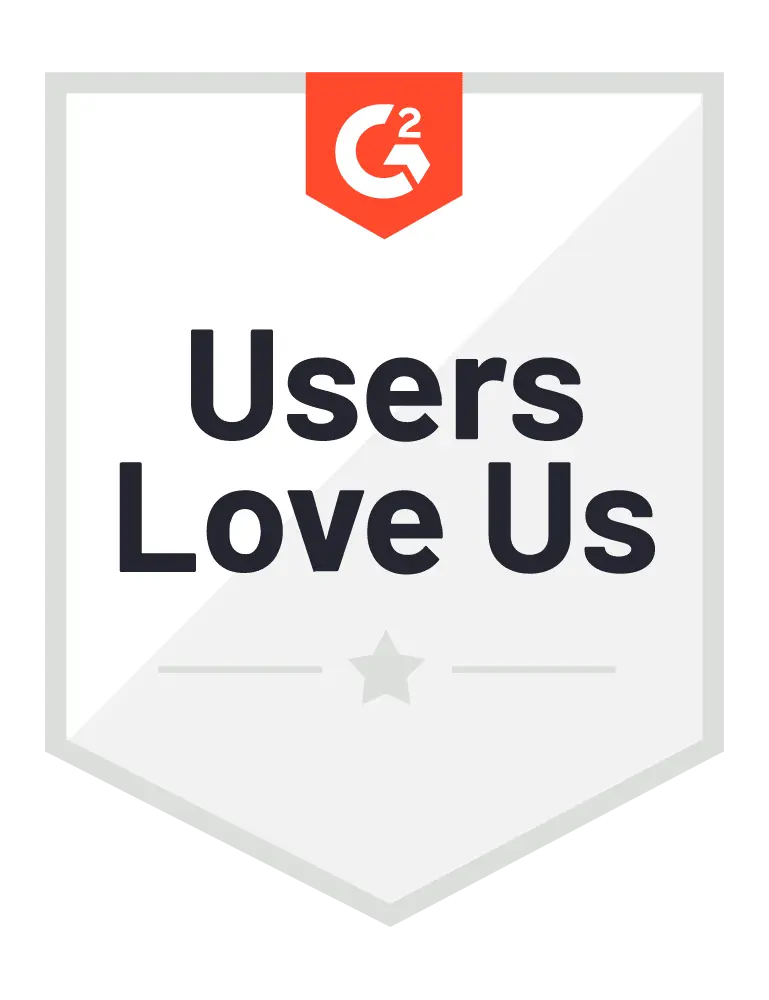
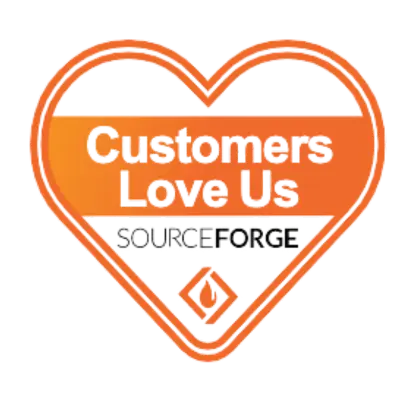
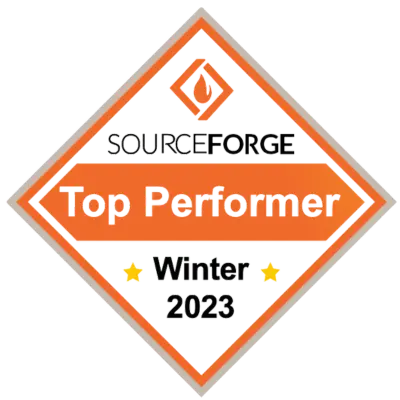
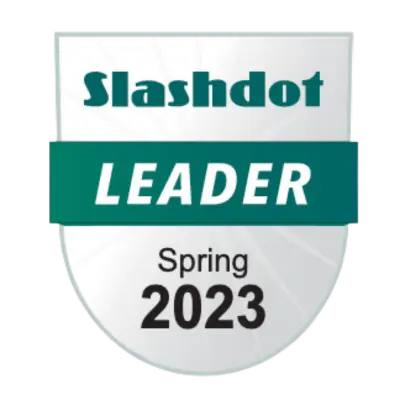
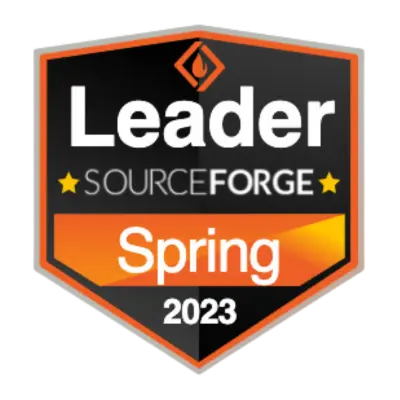
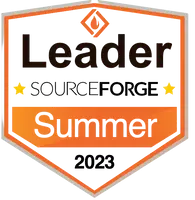
Flexible pricing for every data scientist, team, and company
Hosted Free
For individual data scientists, hobbyists, and exploratory learners
$0per month
- ✓64GB RAM and GPU instances
- ✓Access to JupyterLab, RStudio, and Dask
- ✓Models, Dashboards and Jobs
- ✓Upgrade to Hosted Pro for more hardware - up to 4TB of RAM and 8 GPUs. Billed hourly in $10 increments.
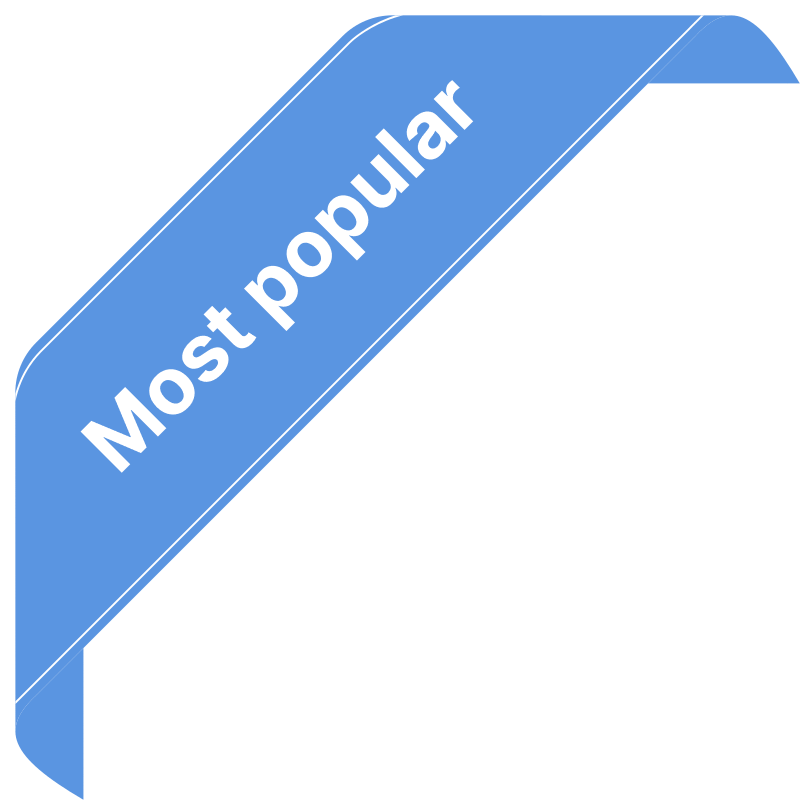
Hosted Orgs
For teams of data scientists, ML/AI engineers, and researchers
$5credit purchase to start
- ✓Pay by the hour billed in $10 increments.
- ✓Share resources easily across team
- ✓Create and manage group-owned resources
- ✓Admin tools to monitor and control usage
Enterprise
For teams, startups, and research groups requiring additional security
Chat with us
- ✓Installs in cloud environments offered by AWS, Microsoft Azure, Google, Oracle, and CoreWeave
- ✓Advanced security: SSO and installation into custom VPCs and private subnets available
- ✓Dedicated technical support
THE LEADING ML PLATFORM
Try Saturn Cloud today
Start on a free Hosted Pro plan to see what Saturn Cloud can do. On a team? Contact Us!