5 Easy Ways to Get Pandas DataFrame Row Count
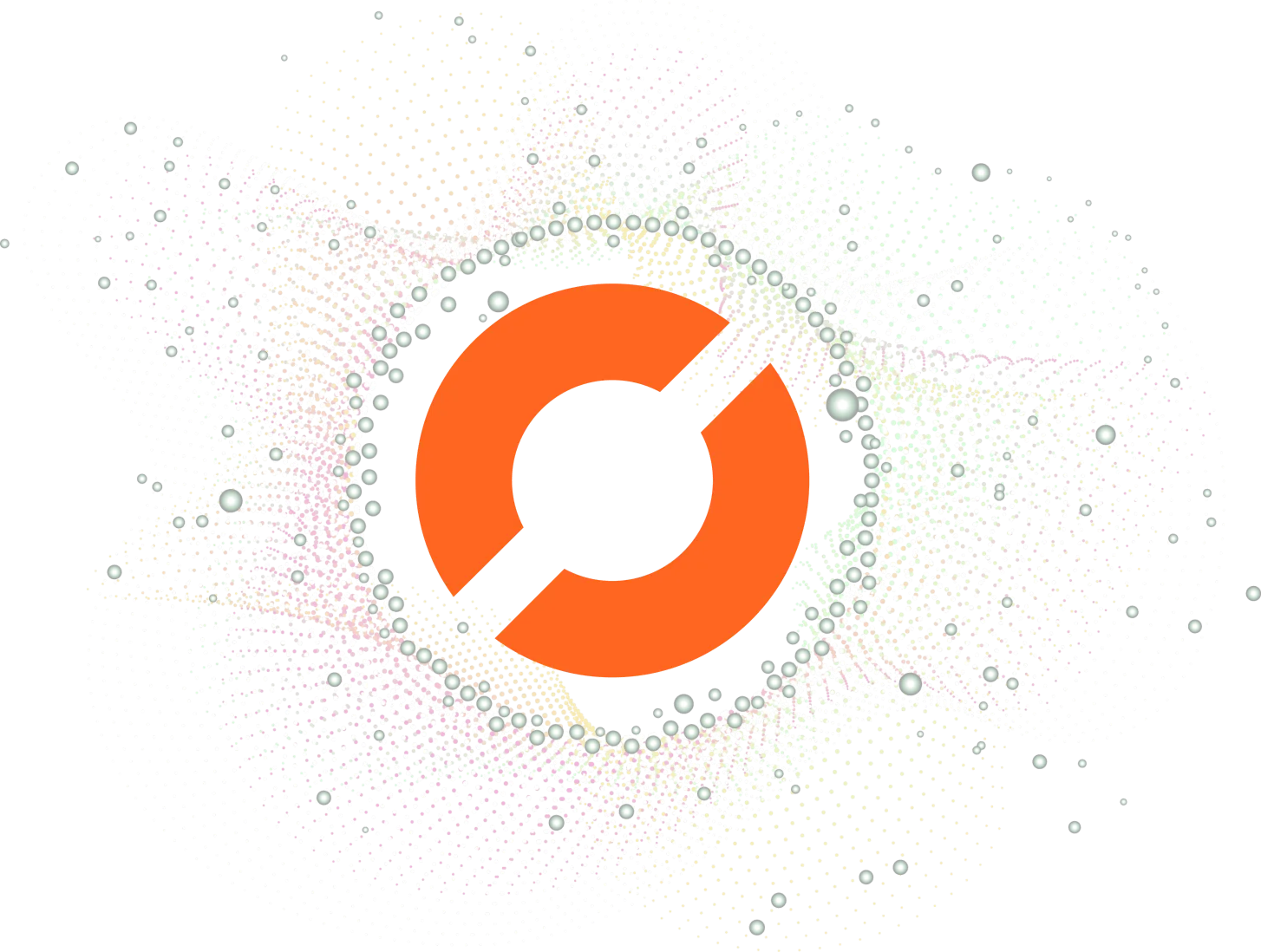
Pandas is a popular open-source data manipulation and analysis library in Python. It offers a wide range of functionalities to work with structured and tabular data, including the DataFrame class, which is a two-dimensional table-like data structure that stores data in rows and columns. One of the most common operations performed on a DataFrame is to get the number of rows it contains. In this blog post, we will explore all the ways to get the row count of a Pandas DataFrame and discuss their advantages and disadvantages. You can also use Pandas with more computing power for free on Saturn Cloud.
Method 1: Using len()
function
The simplest and most straightforward way to get the row count of a Pandas DataFrame is to use the built-in len()
function, which returns the length of an object. Since a DataFrame is a collection of rows, len()
function returns the number of rows in the DataFrame.
Example:
import pandas as pd
df = pd.read_csv('data.csv')
row_count = len(df)
print(f'The DataFrame has {row_count} rows.')
Output:
The DataFrame has 1000 rows.
Advantages:
- Simplicity:
len()
is a simple and intuitive way to get the number of rows in a DataFrame. - Compatibility: It works with various Python data structures, not just DataFrames.
Disadvantages:
- Limited Information:
len()
only returns the number of rows. You won’t get additional details about the DataFrame’s structure or data types.
Method 2: Using shape attribute
Another way to get the row count of a Pandas DataFrame is to use the shape attribute, which returns a tuple of the number of rows and columns in the DataFrame. We can extract the row count by accessing the first element of the tuple.
Example:
import pandas as pd
df = pd.read_csv('data.csv')
row_count = df.shape[0]
print(f'The DataFrame has {row_count} rows.')
Output:
The DataFrame has 1000 rows.
Advantages:
- Detailed Information: shape is specifically designed for DataFrames and provides both the number of rows and columns. This can be helpful for quickly understanding the DataFrame’s structure.
Disadvantages:
- Slightly Less Intuitive: While still relatively simple, shape returns a tuple of
(rows, columns)
, which might require more unpacking if you only want the row count.
Method 3: Using index attribute
The index attribute of a Pandas DataFrame contains the row labels of the DataFrame. We can get the row count by getting the length of the index attribute.
Example:
import pandas as pd
df = pd.read_csv('data.csv')
row_count = len(df.index)
print(f'The DataFrame has {row_count} rows.')
Output:
The DataFrame has 1000 rows.
Advantages:
- Provides the Index:
index()
returns the index object of the DataFrame, which contains information about the row labels and their data type. - Helpful for Advanced Manipulation: It can be useful when you need to access or manipulate the row index in more advanced ways.
Disadvantages:
- Doesn’t Provide Row Count Directly: To get the row count, you would need to use
len(df.index)
ordf.index.size
. It’s not as straightforward as the other methods for this specific purpose.
Method 4: Using count() function
The count()
function of a Pandas DataFrame returns the number of non-null values in each column. We can get the row count by selecting any column and getting its count.
Example:
import pandas as pd
df = pd.read_csv('data.csv')
row_count = df['column_name'].count()
print(f'The DataFrame has {row_count} rows.')
Output:
The DataFrame has 1000 rows.
Advantages:
Flexibility: The
count()
method is quite flexible and can be applied to count non-null values in specific columns, allowing you to find the count of non-null values for each column in the DataFrame. This can be valuable when dealing with missing data.Column-Specific Counts: You can easily obtain counts for individual columns or a subset of columns, which is helpful when you want to analyze the completeness of data in different parts of the DataFrame.
Disadvantages:
Not for Row Count: While
count()
is great for counting non-null values in columns, it is not the most straightforward method for finding the total number of rows in the DataFrame. If your primary goal is to get the row count, there are simpler methods likelen()
and shape.Doesn’t Handle Null Values:
count()
focuses on counting non-null values. If you specifically want to count both non-null and null values (i.e., the total number of elements in the DataFrame), it won’t provide that information.
Method 5: Using info() function
The info()
function of a Pandas DataFrame provides a summary of the DataFrame, including the number of non-null values in each column and the memory usage. We can extract the row count from the summary.
Example:
import pandas as pd
df = pd.read_csv('data.csv')
df.info()
Output:
<class 'pandas.core.frame.DataFrame'>
RangeIndex: 1000 entries, 0 to 999
Data columns (total 5 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 column1 1000 non-null float64
1 column2 1000 non-null int64
2 column3 1000 non-null object
3 column4 1000 non-null bool
4 column5 1000 non-null object
dtypes: bool(1), float64(1), int64(1), object(2)
memory usage: 32.4+ KB
In this example, we can see that the DataFrame has 1000 entries, which corresponds to the number of rows.
Advantages:
- Comprehensive Information:
info()
provides detailed information about the DataFrame, including the number of non-null values in each column, data types, and memory usage. - Helpful for Data Exploration: It’s useful for initial data exploration and understanding the quality of your data.
Disadvantages:
- More Than Just Row Count: If you only need the row count, using
info()
can be overkill. It provides a lot of additional information that might not be necessary in some cases.
Conclusion:
In this blog post, we have explored all the ways to get the row count of a Pandas DataFrame and discussed their advantages and disadvantages. Depending on the specific use case, some methods may be more suitable than others. For small DataFrames or one-time operations, the len()
function or the shape attribute may be the most convenient. For larger DataFrames or more complex operations, the count()
function or the info()
function may be more appropriate. Regardless of the method chosen, it is important to have a clear understanding of how to manipulate and analyze data using Pandas, as it is a powerful tool for data science and machine learning applications.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.