Why the Decision Tree Structure is Only Binary for scikit-learn's DecisionTreeClassifier?
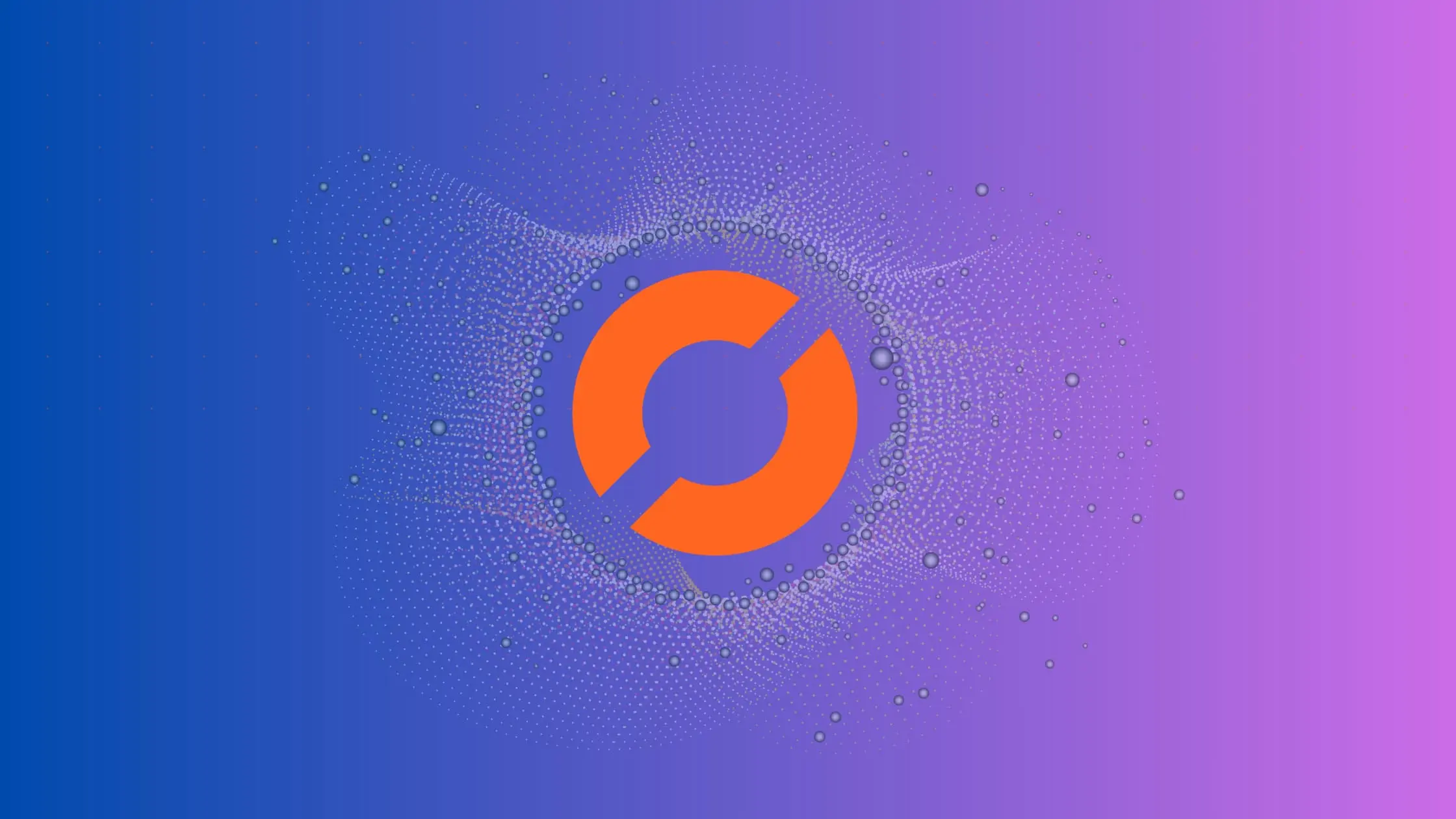
Why the Decision Tree Structure is Only Binary for scikit-learn’s DecisionTreeClassifier?
As a data scientist or software engineer, you may have encountered scikit-learn’s DecisionTreeClassifier, a popular machine learning algorithm used for classification tasks. One of the peculiarities of this algorithm is that it constructs a binary decision tree, where each node has at most two child nodes. In this article, we will explore why the decision tree structure is only binary for scikit-learn’s DecisionTreeClassifier.
What is a Decision Tree?
A decision tree is a supervised learning algorithm that can be used for both classification and regression tasks. It is a tree-like model where each internal node represents a test on an attribute, each branch represents the outcome of the test, and each leaf node represents a class label or a numerical value.
How Does a Decision Tree Work?
A decision tree works by recursively partitioning the feature space into smaller and smaller regions, based on the values of the input features. At each node of the tree, the algorithm chooses the feature that best splits the data into two subsets, such that the subsets are as homogeneous as possible with respect to the target variable. This process continues until a stopping criterion is met, such as reaching a maximum depth or a minimum number of samples per leaf.
Why is the Decision Tree Structure Binary in scikit-learn?
Scikit-learn’s DecisionTreeClassifier is based on the CART (Classification and Regression Trees) algorithm, which constructs binary decision trees. The reason for this is that binary trees have some desirable properties that make them more efficient and easier to interpret than multiway trees.
1. Efficiency
Binary trees have a lower computational complexity than multiway trees because the number of splits required to partition the feature space is logarithmic in the number of samples. In contrast, multiway trees may require an exponential number of splits, which can quickly become computationally infeasible for large datasets.
2. Interpretability
Binary trees are also easier to interpret than multiway trees because they can be visualized more intuitively. A binary tree can be represented as a series of nested if-else statements, where each decision is based on a single feature. This makes it easier for humans to understand how the algorithm is making decisions and to identify which features are the most important for the classification task.
3. Regularization
Binary trees also have better regularization properties than multiway trees. Regularization is the process of adding constraints to a model to prevent overfitting, i.e., fitting the noise in the data instead of the underlying pattern. Binary trees are more prone to underfitting than overfitting, which means that they are less likely to fit the noise in the data. In contrast, multiway trees are more prone to overfitting, which can be mitigated by adding regularization constraints.
Common Errors and How to Handle Them
1. Overfitting
Overfitting is a common issue in decision trees, where the model becomes too specific to the training data.
How to Handle Overfitting:
- Tune Hyperparameters: Adjust parameters like
max_depth
andmin_samples_split
. - Pruning: Implement pruning techniques to remove unnecessary branches.
2. Underfitting
Underfitting occurs when the decision tree is too simple and fails to capture the underlying patterns in the data.
How to Handle Underfitting:
- Increase Complexity: Adjust parameters to increase the tree’s complexity.
- Feature Engineering: Introduce additional relevant features.
3. Handling Missing Values
Decision trees struggle with missing values, potentially affecting model performance.
How to Handle Missing Values:
- Imputation: Fill missing values with the mean, median, or mode.
- Surrogate Splits: Use surrogate splits to make decisions when primary features have missing values.
Examples
1. Building a Binary Decision Tree
# Import necessary libraries
from sklearn.tree import DecisionTreeClassifier
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Load the Iris dataset
iris = load_iris()
X_train, X_test, y_train, y_test = train_test_split(iris.data, iris.target, test_size=0.2, random_state=42)
# Build a binary Decision Tree
clf = DecisionTreeClassifier()
clf.fit(X_train, y_train)
# Make predictions
predictions = clf.predict(X_test)
# Evaluate accuracy
accuracy = accuracy_score(y_test, predictions)
print(f"Accuracy: {accuracy}")
2. Handling Overfitting
# Example of tuning hyperparameters to handle overfitting
clf = DecisionTreeClassifier(max_depth=3, min_samples_split=5)
clf.fit(X_train, y_train)
3. Dealing with Missing Values
# Example of handling missing values with imputation
from sklearn.impute import SimpleImputer
# Assume X_train has missing values
imputer = SimpleImputer(strategy='mean')
X_train_imputed = imputer.fit_transform(X_train)
Conclusion
In conclusion, the decision tree structure is only binary for scikit-learn’s DecisionTreeClassifier because binary trees have some desirable properties that make them more efficient, interpretable, and regularized than multiway trees. Binary trees have a lower computational complexity, are easier to visualize and understand, and have better regularization properties. As a data scientist or software engineer, understanding the decision tree structure can help you to choose the right algorithm for your classification task and to optimize its performance.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.