What is the most efficient way to deep clone an object in JavaScript
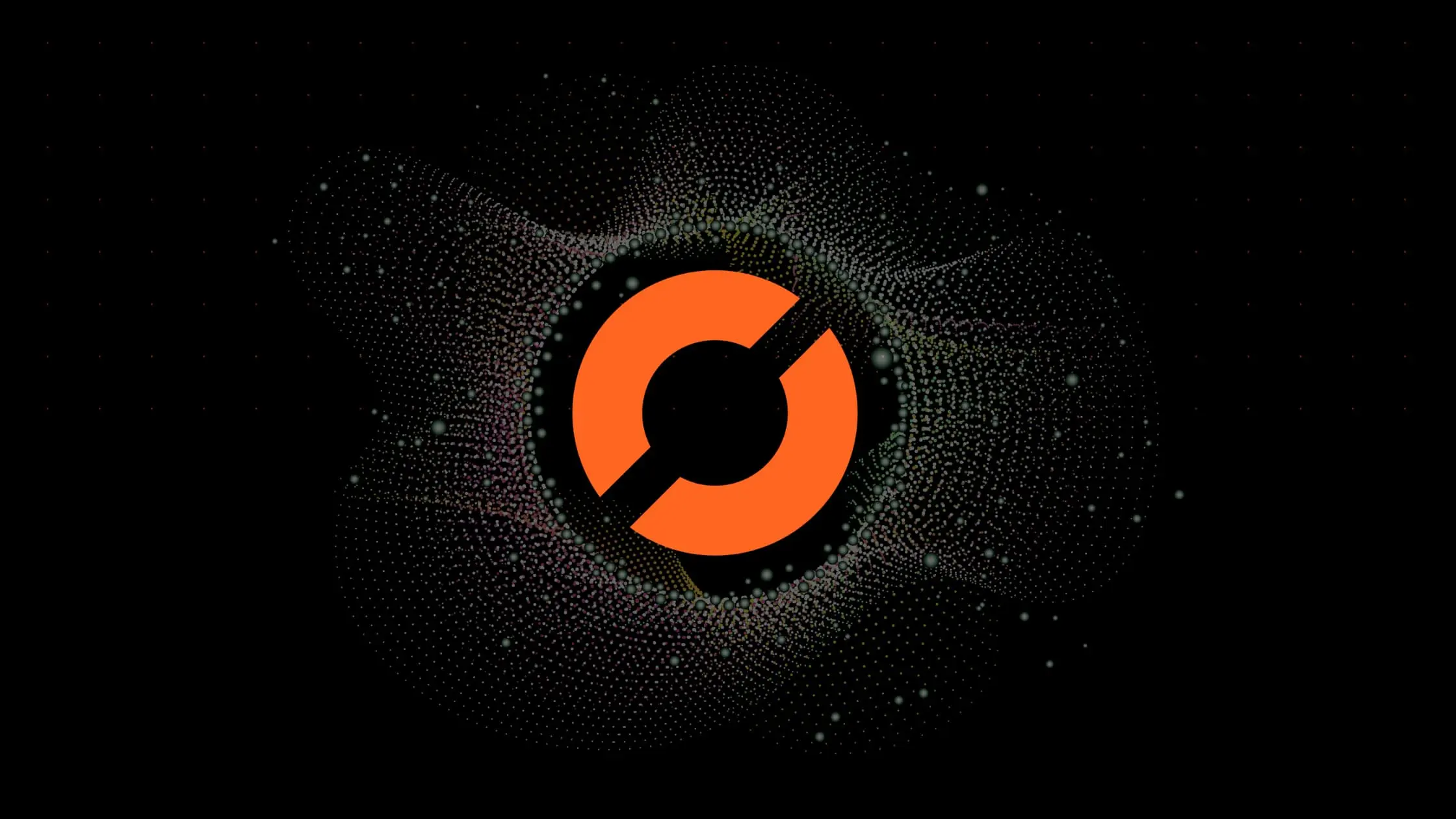
As a software engineer, you have probably encountered situations where you need to create a copy of an object in JavaScript. However, simply copying an object using the assignment operator results in a shallow copy, which means that any changes made to the copy will also affect the original object. To avoid this, you need to create a deep clone of the object.
A deep clone is a copy of an object that contains all of its properties and sub-properties. There are several ways to create a deep clone in JavaScript, but some methods are more efficient than others. In this blog post, we will explore the most efficient ways to deep clone an object in JavaScript.
Table of Contents
- Introduction
- The Problem with Shallow Copy
- Method 1: JSON.parse() and JSON.stringify()
- Method 2: Object.assign()
- Method 3: Recursive Function
- Conclusion
The Problem with Shallow Copy
Before we dive into deep cloning, let’s take a moment to understand the problem with shallow copy. In JavaScript, objects are reference types, which means that when you copy an object using the assignment operator, you are only copying the reference to the object, not the object itself. This results in a shallow copy, where any changes made to the copy will also affect the original object.
const obj1 = { name: "John", age: 30 };
const obj2 = obj1; // shallow copy
obj2.age = 40;
console.log(obj1.age); // Output: 40
In the example above, we create an object obj1
with two properties name
and age
. We then create a shallow copy of obj1
and assign it to obj2
. We then change the age
property of obj2
to 40. When we log the age
property of obj1
, we get 40 instead of the expected value of 30.
Method 1: JSON.parse() and JSON.stringify()
One of the most popular ways to deep clone an object in JavaScript is by using JSON.parse()
and JSON.stringify()
. This method works by first converting the object to a JSON string using JSON.stringify()
, and then parsing the JSON string back into an object using JSON.parse()
.
const obj1 = { name: "John", age: 30 };
const obj2 = JSON.parse(JSON.stringify(obj1)); // deep clone
obj2.age = 40;
console.log(obj1.age); // Output: 30
In the example above, we create an object obj1
with two properties name
and age
. We then create a deep clone of obj1
using JSON.parse()
and JSON.stringify()
and assign it to obj2
. We then change the age
property of obj2
to 40. When we log the age
property of obj1
, we get 30, which is the expected value.
This method works for most objects, but it has some limitations. Objects with circular references or functions cannot be converted to JSON, so this method will not work for those types of objects.
Method 2: Object.assign()
Another way to create a deep clone of an object in JavaScript is by using Object.assign()
. This method works by creating a new object and copying all of the properties from the original object to the new object.
const obj1 = { name: "John", age: 30 };
const obj2 = Object.assign({}, obj1); // deep clone
obj2.age = 40;
console.log(obj1.age); // Output: 30
In the example above, we create an object obj1
with two properties name
and age
. We then create a deep clone of obj1
using Object.assign()
and assign it to obj2
. We then change the age
property of obj2
to 40. When we log the age
property of obj1
, we get 30, which is the expected value.
This method works for most objects, but it has some limitations. Objects with circular references cannot be cloned using this method.
Method 3: Recursive Function
A third way to deep clone an object in JavaScript is by using a recursive function. This method works by iterating through all the properties of the object and creating a copy of each property. If a property is an object, the function will recursively call itself to create a deep clone of the object.
function deepClone(obj) {
if (typeof obj !== "object" || obj === null) {
return obj;
}
let clone = {};
for (let key in obj) {
clone[key] = deepClone(obj[key]);
}
return clone;
}
const obj1 = { name: "John", age: 30, address: { city: "New York", state: "NY" } };
const obj2 = deepClone(obj1); // deep clone
obj2.address.city = "Los Angeles";
console.log(obj1.address.city); // Output: New York
In the example above, we create an object obj1
with three properties name
, age
, and address
. The address
property is itself an object with two properties city
and state
. We then create a deep clone of obj1
using a recursive function and assign it to obj2
. We then change the city
property of obj2.address
to “Los Angeles”. When we log the city
property of obj1.address
, we get “New York”, which is the expected value.
This method works for all types of objects, including objects with circular references or functions.
Conclusion
In conclusion, there are several ways to deep clone an object in JavaScript, but some methods are more efficient than others. JSON.parse()
and JSON.stringify()
is a popular method, but it has some limitations. Object.assign()
is another method that works for most objects, but it also has some limitations. A recursive function is the most versatile method and can handle all types of objects, including objects with circular references or functions.
When choosing a method, it is important to consider the type of object you are cloning and the performance requirements of your application. Choose the method that best suits your needs and write efficient code that works flawlessly.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.