What Is the Factorial Algorithm and How Does It Calculate Large Factorials?
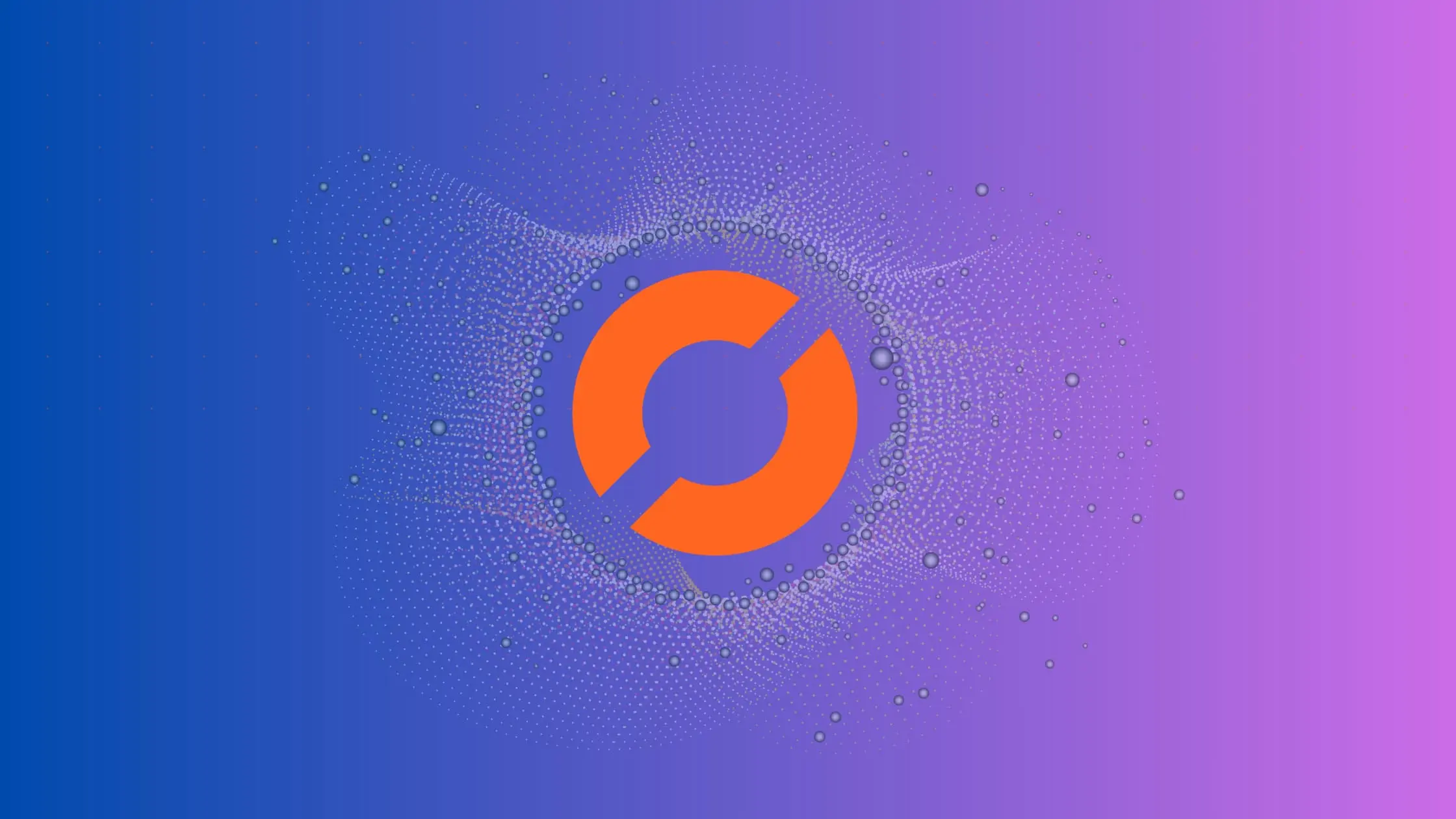
As a data scientist or software engineer, you may come across situations where you need to calculate factorials of large numbers. Factorials are commonly used in mathematics and statistics, particularly in combinatorics and probability theory. However, calculating factorials for large numbers can be challenging due to the rapid growth of factorial values.
In this article, we will explain the algorithm used to calculate large factorials and discuss its implementation. By the end, you will have a clear understanding of how to calculate factorials efficiently, even for extremely large numbers.
Understanding Factorials
Before diving into the algorithm, let’s quickly recap what factorials are. The factorial of a non-negative integer n
, denoted as n!
, is the product of all positive integers less than or equal to n
. For example, 5!
(read as “5 factorial”) is calculated as:
5! = 5 * 4 * 3 * 2 * 1 = 120
Factorials grow rapidly as the input number increases. For instance, 20!
is equal to 2,432,902,008,176,640,000
. Calculating such large factorials using a naive approach would be extremely time-consuming and inefficient.
The Recursive Algorithm
One of the most common algorithms for calculating factorials is the recursive approach. This algorithm breaks down the factorial calculation into smaller subproblems until it reaches the base case. Here’s the recursive algorithm for calculating factorials:
function factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
Let’s walk through the algorithm step by step:
- If the input
n
is equal to 0, we have reached the base case and return 1, as0!
is defined as 1. - Otherwise, we recursively call the
factorial
function with the argumentn-1
and multiply the result byn
.
This algorithm works well for smaller numbers, but for large factorials, it can quickly consume a significant amount of memory and time due to the repeated function calls and stack usage.
Advantages:
Simplicity: The recursive algorithm is conceptually straightforward, making it easy to understand and implement.
Elegance: It reflects the mathematical definition of factorials, breaking down the problem into smaller subproblems.
Clarity: The recursive structure enhances code readability, aiding in comprehension for those familiar with recursive paradigms.
Disadvantages:
Memory Consumption: Recursive calls may lead to a large stack usage, consuming significant memory, especially for large factorials.
Performance: For extremely large factorials, the recursive approach can be inefficient and time-consuming due to repeated function calls.
Stack Limitations: Recursive depth is constrained by stack limits, potentially causing stack overflow errors for very large input values.
The Iterative Algorithm
To calculate large factorials more efficiently, we can use an iterative algorithm. This approach avoids the overhead of function calls and utilizes a loop to calculate the factorial. Here’s the iterative algorithm for calculating factorials:
function factorial(n):
result = 1
for i from 2 to n:
result *= i
return result
Let’s break down the iterative algorithm:
- We initialize the
result
variable to 1, as1!
is defined as 1. - Starting from 2, we iterate through all the integers up to
n
. - In each iteration, we multiply the
result
by the current integeri
. - Finally, we return the
result
as the factorial ofn
.
The iterative algorithm calculates factorials more efficiently than the recursive algorithm, especially for large numbers. It avoids the overhead of function calls and utilizes a single loop to calculate the factorial in a straightforward manner.
Advantages:
Efficiency: The iterative algorithm is more efficient for large factorials, avoiding the overhead of recursive function calls.
Reduced Memory Usage: It uses a single loop, minimizing memory consumption and eliminating the risk of stack overflow.
Scalability: Well-suited for handling extremely large factorials, offering better scalability compared to the recursive approach.
Disadvantages:
Learning Curve: The iterative approach may have a steeper learning curve for those less familiar with loop-based algorithms.
Code Complexity: The code may be perceived as less elegant compared to the recursive version, especially for those with a preference for recursive paradigms.
Algorithmic Understanding: The iterative algorithm may deviate from the mathematical definition of factorials, potentially making it less intuitive.
Handling Large Factorials
Even with the iterative algorithm, calculating factorials for extremely large numbers can still pose challenges. The factorial values grow rapidly, and they can exceed the limits of the available data types. To handle such cases, you may need to use libraries or data structures that support arbitrary precision arithmetic.
For example, Python provides the math
and decimal
modules, which offer functions and classes to handle large factorials and arbitrary precision arithmetic. These libraries allow you to perform calculations with precision and accuracy, even for extremely large numbers.
Error Handling:
Input Validation: Implement input validation to ensure that the input is a non-negative integer, as factorials are only defined for non-negative integers.
Data Type Checks: Warn users about potential data type limitations and suggest using libraries or techniques supporting arbitrary precision arithmetic for accurate calculations.
Conclusion
In this article, we explored the algorithm for calculating large factorials. We discussed both the recursive and iterative approaches and highlighted the advantages of the iterative algorithm for efficiency. We also touched upon handling large factorials using libraries or data structures that support arbitrary precision arithmetic.
By understanding these algorithms and utilizing appropriate techniques, you can efficiently calculate factorials for both small and large numbers. Factorials are essential in many areas of mathematics, and having a solid grasp of the algorithms behind their calculation is valuable for data scientists and software engineers alike.
Remember, when dealing with large factorials, it’s important to consider the limitations of data types and explore libraries or techniques that support arbitrary precision arithmetic. This will ensure accurate and efficient calculations, even for factorial values that exceed the capabilities of standard data types.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.