What Is the CUDA Out of Memory Error and How to Fix It
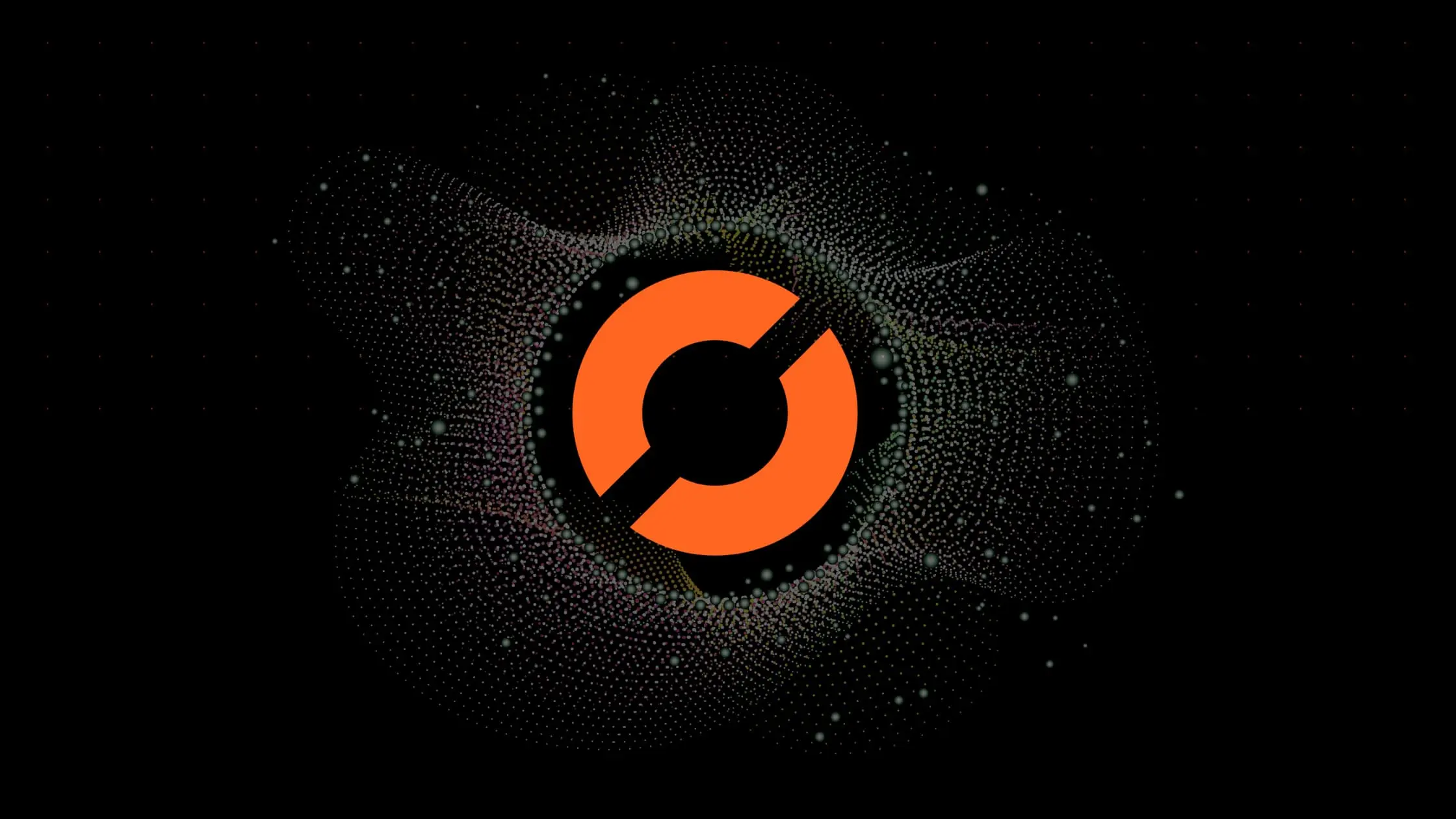
As a data scientist or software engineer working with deep learning models, you may have encountered the dreaded “CUDA out of memory” error. This error occurs when the GPU memory is empty, but the program still cannot allocate memory for a new operation. This error can be frustrating to deal with, especially when you have limited time to work on a project. In this article, we will discuss what causes the CUDA out of memory error and how to fix it.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.
Table of Contents
What Causes the CUDA Out of Memory Error?
The CUDA out of memory error occurs when the GPU has insufficient memory to execute a particular operation. This error can be caused by several factors, including:
1. Model Size
The size of your model can significantly impact the amount of GPU memory required to run it. If your model is too large for your GPU memory, you may encounter the CUDA out of memory error.
2. Batch Size
The batch size is another critical factor that can determine the amount of GPU memory required to run a model. Larger batch sizes require more GPU memory, and if the batch size is too large for your GPU, you may encounter the CUDA out of memory error.
3. GPU Memory Leaks
GPU memory leaks can also cause the CUDA out of memory error. Memory leaks occur when a program fails to release memory, leading to a gradual reduction in available memory. Over time, this can cause the program to run out of memory.
How to Fix the CUDA Out of Memory Error?
Now that we know what causes the CUDA out of memory error let’s explore how to fix it.
1. Reduce the Model Size
One of the most effective ways to fix the CUDA out of memory error is to reduce the size of your model. You can do this by reducing the number of layers, parameters, or features. You can also consider using a pre-trained model, which can significantly reduce the size of your model.
2. Reduce the Batch Size
Another way to fix the CUDA out of memory error is to reduce the batch size. This can be done by reducing the number of samples fed into the model at once. While this may impact the model’s performance, it can significantly reduce the amount of GPU memory required to run the model.
# Code example for reducing batch size
import torch
# Original batch size
batch_size = 64
# Reduce batch size
batch_size = 32
3. Use Mixed Precision
Mixed precision is a technique that can significantly reduce the amount of GPU memory required to run a model. This technique involves using lower-precision floating-point numbers, such as half-precision (FP16), instead of single-precision (FP32). This can reduce the memory footprint of the model without significantly impacting the model’s performance.
import torch
import torch.nn as nn
import torch.optim as optim
from torch.cuda.amp import GradScaler, autocast
# Create an instance of the model
model = SimpleModel()
# Define your loss function and optimizer
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
# Create a GradScaler for mixed precision training
scaler = GradScaler()
# Training loop
for epoch in range(num_epochs):
for inputs, labels in dataloader:
# Move data to GPU
inputs, labels = inputs.to(device), labels.to(device)
# Zero the gradients
optimizer.zero_grad()
# Forward pass and loss calculation using autocast
with autocast():
outputs = model(inputs)
loss = criterion(outputs, labels)
# Backward pass and optimization using scaled gradients
scaler.scale(loss).backward()
scaler.step(optimizer)
scaler.update()
# Print some information
if (iteration + 1) % log_interval == 0:
print(f'Epoch [{epoch+1}/{num_epochs}], Step [{iteration+1}/{total_steps}], Loss: {loss.item():.4f}')
# Remember to use scaler.update() to update the scale factor during training.
4. Use Gradient Checkpointing
Gradient checkpointing is another technique that can help reduce the GPU memory required to run a model. This technique involves storing only a subset of the intermediate activations during the forward pass and recomputing the rest during the backward pass. This can significantly reduce the amount of GPU memory required to run the model.
5. Fix GPU Memory Leaks
If the CUDA out of memory error is caused by GPU memory leaks, you can fix it by identifying and fixing the leaks. This can be done by using profiling tools to identify the memory leaks and modifying the code to release memory correctly.
# Code example for memory leak detection
import torch
# Some code with potential memory leaks
# Check GPU memory statistics
print(torch.cuda.memory_stats())
6. Freeing GPU Memory
Explicitly releasing GPU memory can be achieved by using tools like torch.cuda.empty_cache() or restarting the Python kernel.
# Code example for freeing GPU memory
import torch
# Some code consuming GPU memory
# Free up GPU memory
torch.cuda.empty_cache()
Conclusion
The CUDA out of memory error can be frustrating to deal with, but it is not insurmountable. By understanding what causes the error and using the techniques outlined in this article, you can effectively fix the error and continue working on your deep learning projects. Remember to always keep an eye on the size of your models and batch sizes, and use techniques such as mixed precision and gradient checkpointing to reduce the amount of GPU memory required to run your models.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.