What does model.train() do in PyTorch
model.train
function in PyTorch, which is pivotal for setting the model in training mode. The post aims to provide insights into the functionality of model.train
and its significant impact on the overall training process.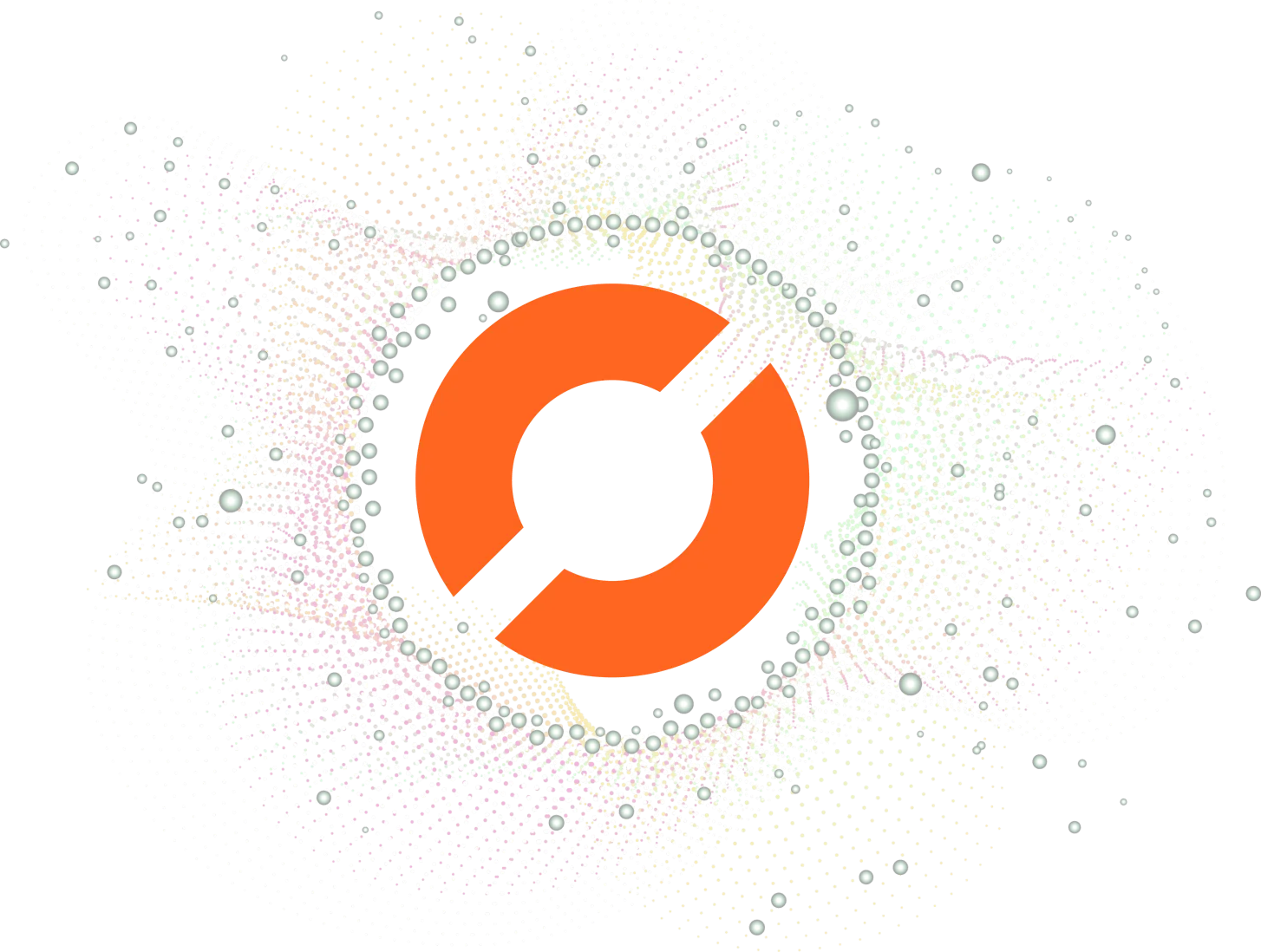
As a data scientist, you are probably familiar with PyTorch, a popular open-source machine learning library that allows you to build and train deep learning models. One of the most important functions in PyTorch is model.train()
, which sets the model in training mode. In this blog post, we will explore what model.train()
does and how it impacts the training process.
Table of Contents
- What is model.train()?
- How does model.train() impact the training process?
- Common Errors and How to Handle Them
- Conclusion
What is model.train()
?
model.train()
is a PyTorch function that sets the model in training mode. When you call model.train()
, PyTorch enables features such as dropout and batch normalization, which are typically used during training but not during inference. These features help prevent overfitting and improve the model’s generalization ability.
By default, PyTorch initializes a model in evaluation mode. In evaluation mode, the model behaves differently than in training mode. For example, dropout layers are disabled, and batch normalization layers use the running statistics instead of the mini-batch statistics. This is because during inference, we want the model to make predictions on new data, not to learn from it.
How does model.train()
impact the training process?
When you call model.train()
, PyTorch enables the training-specific features that are disabled by default in evaluation mode. These features include:
Dropout
Dropout is a regularization technique that randomly drops out some of the neurons during training. This helps prevent overfitting by forcing the model to learn more robust features that are not specific to the training data. When you call model.train()
, PyTorch enables dropout layers in the model.
Batch normalization
Batch normalization is a technique that normalizes the input to each layer, making the training process more stable and faster. During training, batch normalization uses the mini-batch statistics to normalize the input. However, during inference, we don’t have access to the mini-batch statistics, so we use the running statistics instead. When you call model.train()
, PyTorch enables batch normalization layers in the model.
Gradients
During training, PyTorch computes the gradients of the loss function with respect to the model parameters. These gradients are used to update the parameters using an optimization algorithm such as stochastic gradient descent. When you call model.train()
, PyTorch enables the computation of gradients.
Autograd
Autograd is the automatic differentiation engine in PyTorch that computes the gradients of the loss function with respect to the model parameters. When you call model.train()
, PyTorch enables autograd.
Forward and backward passes
When you call model.train()
, PyTorch enables the forward and backward passes through the model. During the forward pass, the inputs are passed through the layers in the model to generate the output. During the backward pass, the gradients are computed and used to update the parameters.
Common Errors and How to Handle Them
1. Forgetting to Switch Modes
One common error is forgetting to set the model to training mode using model.train(). This can lead to discrepancies in layer behaviors, affecting the model’s performance.
# Common Error
model = MyModel()
optimizer = torch.optim.SGD(model.parameters(), lr=0.001)
# Oops! Forgot to set the model to training mode
model.forward(input_data)
loss.backward()
optimizer.step()
Solution:
Always ensure to set the model to training mode using model.train()
before the training loop.
2. Memory Constraints
# Common Error
model = MyLargeModel()
data_loader = DataLoader(train_data, batch_size=64, shuffle=True)
# Training loop without managing memory
for inputs, labels in data_loader:
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
Solution: Consider reducing the batch size or using gradient accumulation to mitigate memory issues.
3. Inconsistent Batch Normalization Statistics
Incorrect usage of batch normalization during training can result in suboptimal model performance. Ensure that batch normalization layers utilize batch statistics during training by invoking model.train().
# Common Error
model = MyModel()
optimizer = torch.optim.SGD(model.parameters(), lr=0.001)
# Oops! Forgot to set the model to training mode
model.train()
for inputs, labels in train_loader:
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
Solution: Always set the model to training mode before processing batches, especially if batch normalization layers are present.
Conclusion
In this blog post, we’ve explored what model.train()
does in PyTorch and how it impacts the training process. By calling model.train()
, PyTorch enables the training-specific features such as dropout and batch normalization, which help prevent overfitting and improve the model’s generalization ability. PyTorch also computes the gradients of the loss function with respect to the model parameters using autograd and performs the forward and backward passes through the model. Understanding what model.train()
does is essential for building and training deep learning models in PyTorch.
About Saturn Cloud
Saturn Cloud is your all-in-one solution for data science & ML development, deployment, and data pipelines in the cloud. Spin up a notebook with 4TB of RAM, add a GPU, connect to a distributed cluster of workers, and more. Request a demo today to learn more.
Saturn Cloud provides customizable, ready-to-use cloud environments for collaborative data teams.
Try Saturn Cloud and join thousands of users moving to the cloud without
having to switch tools.